Map or Structure in C
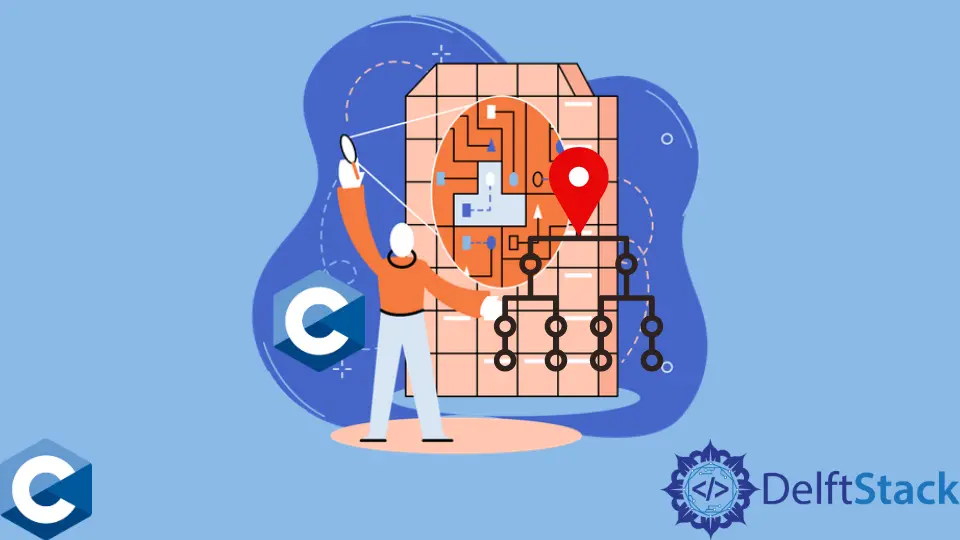
This tutorial will discuss using the map or structure to create a collection of variables in C.
Map or Structure in C
A structure is a collection of variables of different or the same data types. For example, if we want to create a profile for a person to store the person’s age, name, and height, we can use structure to store all the information under a single map or structure.
We can use the struct
keyword to create structures in the C language. The basic syntax of a structure is given below.
struct NameOfStructure {
dataType_1 member_1;
dataType_2 member_2;
....
};
For example, let’s create a structure to store the information of a person. See the code below.
struct Person {
char *name;
int age;
float height;
};
A structure is a class we can use to create objects containing all the variables defined inside the structure. We have to write the struct
keyword, then the structure’s name and then the object’s name to create an object of the structure.
We can access any structure member using the object name and the variable’s name attached with a dot. We can get the value of the variables or change their value using the assignment operator.
For example, let’s create two objects of the above structure, store some value in them, and print it using the printf()
function. See the code below.
#include <stdio.h>
struct Person {
char *name;
int age;
float height;
};
int main() {
struct Person person1, person2;
person1.name = "Max";
person1.age = 20;
person1.height = 6.2;
printf("Person 1 name = %s\n", person1.name);
printf("Person 2 name = %s", person2.name);
}
Output:
Person 1 name = Max
Person 2 name =
In the above code, we printed the name of the two person
objects. We can see in the output that the name of the first person is shown, but the name of the second person is not printed because we have not stored any name for the second person.
The variable values of the two structure objects can be identical or different but are independent. We can also get or change the values of other variables like in the above code.
We can also create structures inside other structures called nested structures. We have to create an object of the structure we want to attach with the other structure and then use the object name to access its variables.
For example, let’s create another structure and link it with the above structure. See the code below.
#include <stdio.h>
struct job {
char *name;
int duration;
};
struct Person {
struct job j;
char *name;
int age;
float height;
};
int main() {
struct Person person1, person2;
person1.j.name = "Engineer";
person1.name = "Max";
person1.age = 20;
person1.height = 6.2;
printf("Person 1 job name = %s\n", person1.j.name);
printf("Person 1 name = %s", person1.name);
}
Output:
Person 1 job name = Engineer
Person 1 name = Max
In the above code, we created another structure to store the information about the job of a person and to access its variables, we first have to access the parent structure, which is Person
in this case, and then we can access the variables of the structure job
.
We have created and linked two structures, but we can create as many structures as we want and link them to one another.
In the case of a large number of nested structures, we have to make sure we are using the correct map to get the information of a variable. A structure is useful when we want to store information about many things like information about people and their job.