How to Convert String to Lowercase in C
-
Use the
tolower
Function to Convert String to Lowercase in C - Use the Custom Function to Convert String to Lowercase in C
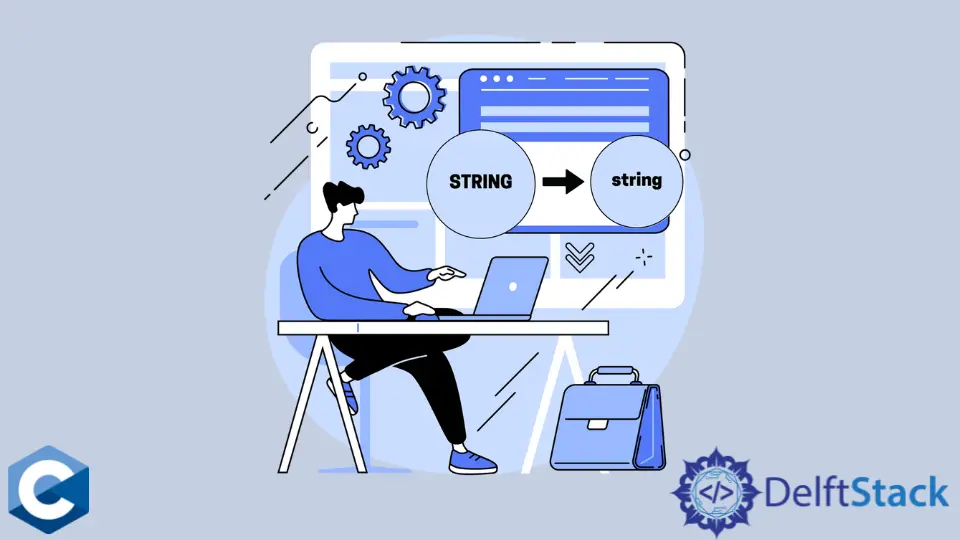
This article will demonstrate multiple methods about how to convert string to lowercase in C.
Use the tolower
Function to Convert String to Lowercase in C
The tolower
function is part of the C standard library defined in the <ctype.h>
header file. tolower
takes one int
type argument and returns the converted value of the given character if the corresponding lowercase representation exists.
Note that the passed character needs to be either EOF or unsigned char
type representable. In this case, we initialize the char
pointer with a string literal value and then iterate over each character to convert to lowercase values.
That passing char
type arguments to tolower
function must be cast to unsigned char
.
#include <ctype.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
char *str = "THIS STRING LITERAL IS ARBITRARY";
printf("%s\n", str);
for (size_t i = 0; i < strlen(str); ++i) {
printf("%c", tolower((unsigned char)str[i]));
}
printf("\n");
exit(EXIT_SUCCESS);
}
Output:
THIS STRING LITERAL IS ARBITRARY
this string literal is arbitrary
Previous example code overwrites contents of the original string with the converted, lowercase characters. Alternatively, we can allocate another char
pointer using calloc
, which is similar to malloc
except that it zeros out the allocated memory and store the converted string separately.
Notice that the pointer should be freed before the program exits, or if the process is long-running, it should be freed as soon as the string variable is not needed.
#include <ctype.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
char *str = "THIS STRING LITERAL IS ARBITRARY";
printf("%s\n", str);
size_t len = strlen(str);
char *lower = calloc(len + 1, sizeof(char));
for (size_t i = 0; i < len; ++i) {
lower[i] = tolower((unsigned char)str[i]);
}
printf("%s", lower);
free(lower);
exit(EXIT_SUCCESS);
}
Output:
THIS STRING LITERAL IS ARBITRARY
this string literal is arbitrary
Use the Custom Function to Convert String to Lowercase in C
A more flexible solution would be to implement a custom function that takes the string variable as the argument and returns the converted lowercase string at a separate memory location. This method is essentially the decoupling of the previous example from the main
function.
In this case, we created a function toLower
that takes char*
, where a null-terminated string is stored and a size_t
type integer denoting the string’s length. The function allocates the memory on the heap using the calloc
function; thus the caller is responsible for deallocating the memory before the program exits.
#include <ctype.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char *toLower(char *str, size_t len) {
char *str_l = calloc(len + 1, sizeof(char));
for (size_t i = 0; i < len; ++i) {
str_l[i] = tolower((unsigned char)str[i]);
}
return str_l;
}
int main() {
char *str = "THIS STRING LITERAL IS ARBITRARY";
printf("%s\n", str);
size_t len = strlen(str);
char *lower = toLower(str, len);
printf("%s", lower);
free(lower);
exit(EXIT_SUCCESS);
}
Output:
THIS STRING LITERAL IS ARBITRARY
this string literal is arbitrary
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook