Long Double in C
-
the
long double
in C - Format Specifier of Datatype in C
- Create a Project in C
- Implement Long Double in C
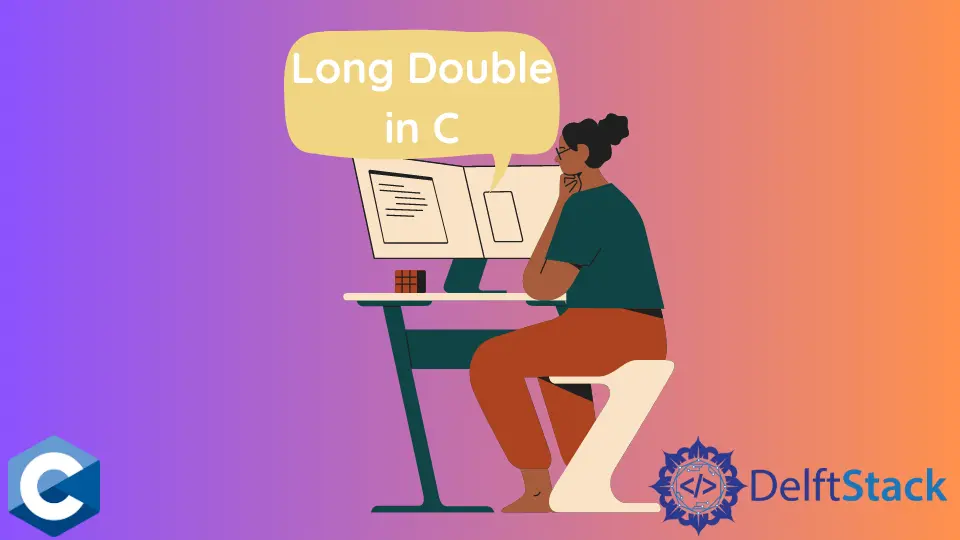
This tutorial will explain the datatype of long double
in C language and its format specifier.
the long double
in C
In C language, long double
is used as a variable’s datatype. We must specify the data type and the variable name in the variable declaration statement.
For example, In the following statement, long double
is the data type, and d
is the variable’s name.
long double d;
In C language, we have three data types used for decimal numbers. They are pretty similar, but their precision value is different, and their bytes in memory are different.
These data types are float
, double
, and long double
.
float
requires 4 bytes in memory, and its precision is up to 6 digits.double
requires 8 bytes in memory, and its precision is up to 15 digits.long double
requires 10 bytes in memory, and its precision is up to 19 digits.
Format Specifier of Datatype in C
Format specifiers specify the format in which the value of a variable is represented on the screen. These are defined in the format string
.
In the following code example, we used the format specifier of float, double and long double.
Code:
#include <stdio.h>
int main() {
float a = 5.5;
double b = 6.78;
long double c = 4.5678;
printf("The value of a is %f\n", a);
printf("The value of b is %lf\n", b);
printf("The value of a is %Lf\n", c);
return 0;
}
The %f
, %lf
, and %Lf
format specifiers of float
, double
, and long double
, respectively.
Output:
The value of a is 5.500000
The value of b is 6.780000
The value of a is 4.567800
Create a Project in C
-
The first step is to install a compiler. Steps to download and install C compiler.
-
In the next step, create an empty project in C language by clicking on the
File
in the menu bar. -
Save the file before compiling.
-
Execute the code. By clicking on
Compile & Run
. -
An execution screen will appear.
Implement Long Double in C
This code snippet shows the behavior of float, double and long double with decimal numbers in exponential form.
Code:
#include <stdio.h>
int main(void) {
float a = 450000.0;
double b = 3.314e3;
long double c = 8.567e-2;
printf("%f will be displayed as %e\n", a, a);
printf("%lf will be displayed as %e\n", b, b);
printf("%Lf will be displayed as %Le\n", c, c);
return 0;
}
First, we have a standard input/ output header file. Then, in the main
function, we initialized the three variables having decimal numbers in exponential form.
The three variables have different datatypes. Then in the format string
, we have displayed all three numbers using their respective format specifiers
.
After that, the function returns 0
because the main function has a return type of integer.
Output:
450000.000000 will be displayed as 4.500000e+05
3314.000000 will be displayed as 3.314000e+03
0.085670 will be displayed as 8.567000e-02