How to Implicitly Declare A Function in C
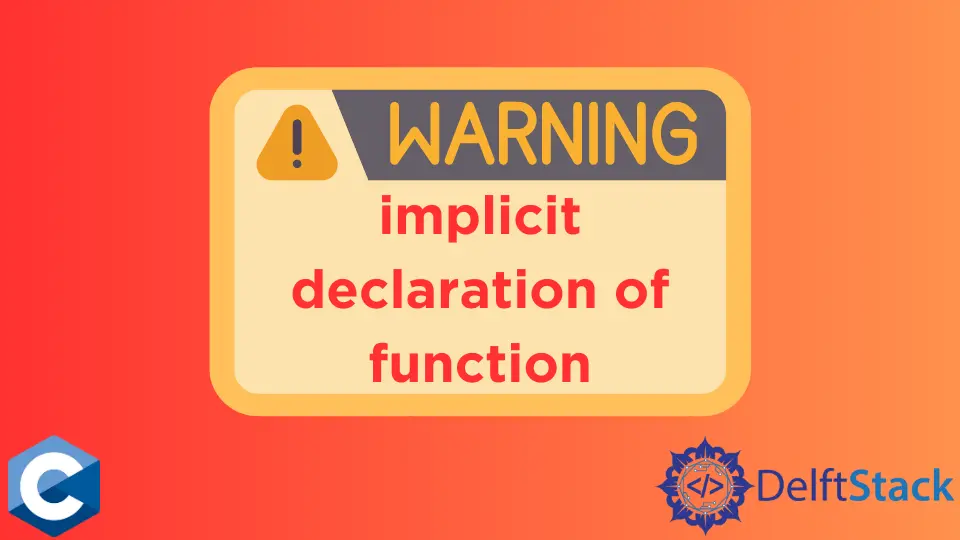
This tutorial discusses removing the warning of implicit function declaration by declaring the functions above the main function in C.
Implicit Declaration of Function in C
Sometimes, the compiler shows a warning of implicit declaration of the function in C language, which means that the function is not declared on top of the main()
function or its header file is not included.
For example, the printf()
function belongs to the stdio.h
header file, and if we don’t include it before using it in a C source file, the compiler will show a warning that the function declaration is implicit.
In this case, we have to include the header file, which includes the function declaration or declare the function above the main()
function. For example, let’s use the printf()
function without including its header file.
See the code below.
int main() {
int a = 10;
printf("a = %d", a);
return 0;
}
Output:
main.c: In function 'main':
main.c:5:5: warning: implicit declaration of function 'printf' [-Wimplicit-function-declaration]
5 | printf("a = %d",a);
| ^~~~~~
main.c:5:5: warning: incompatible implicit declaration of built-in function 'printf'
main.c:1:1: note: include '<stdio.h>' or provide a declaration of 'printf'
+++ |+#include <stdio.h>
1 |
a = 10
In the above output, we can see that the compiler gave a warning saying that the declaration of the printf()
function is implicit and we have to include the <stdio.h>
file, or we must provide a declaration of the printf()
function in the source file.
We can also see that the value of a
is printed, which means the printf()
function worked perfectly even though we have not included its header file.
Now, let’s include the header file and repeat the above example. See the code below.
#include <stdio.h>
int main() {
int a = 10;
printf("a = %d", a);
return 0;
}
Output:
a = 10
We can see above the warning of the implicit function declaration is not shown this time because we have included the header file for the printf()
function.
The implicit function declaration warning will also be shown if we have created a function in a source file but have not declared it above the main()
function.
The compiler warns when we try to call the function that the function declaration is implicit. For example, let’s create a function and call it from the main()
function without declaring it above the main()
function.
#include <stdio.h>
int main() {
int a = fun(2, 3);
printf("a = %d", a);
return 0;
}
int fun(int x, int p) {
int a = x + p;
return a;
}
Output:
main.c: In function 'main':
main.c:4:13: warning: implicit declaration of function 'fun' [-Wimplicit-function-declaration]
4 | int a = fun(2, 3);
| ^~~
a = 5
In the above output, we can see that the warning is shown for the function we created. We have to declare the function above the main()
function to solve this problem.
For example, let’s declare the function above the main()
function and repeat the above example. See the code below.
#include <stdio.h>
int fun(int x, int p);
int main() {
int a = fun(2, 3);
printf("a = %d", a);
return 0;
}
int fun(int x, int p) {
int a = x + p;
return a;
}
Output:
a = 5
We can see that the warning is now gone. We can also declare the function in a header file and then include the header file in the source file, which is useful in the case of many functions because it will simplify the code.