#ifndef Include Guards in C
- Understanding Include Guards
- Implementing #ifndef Include Guards
- Best Practices for Using Include Guards
- Conclusion
- FAQ
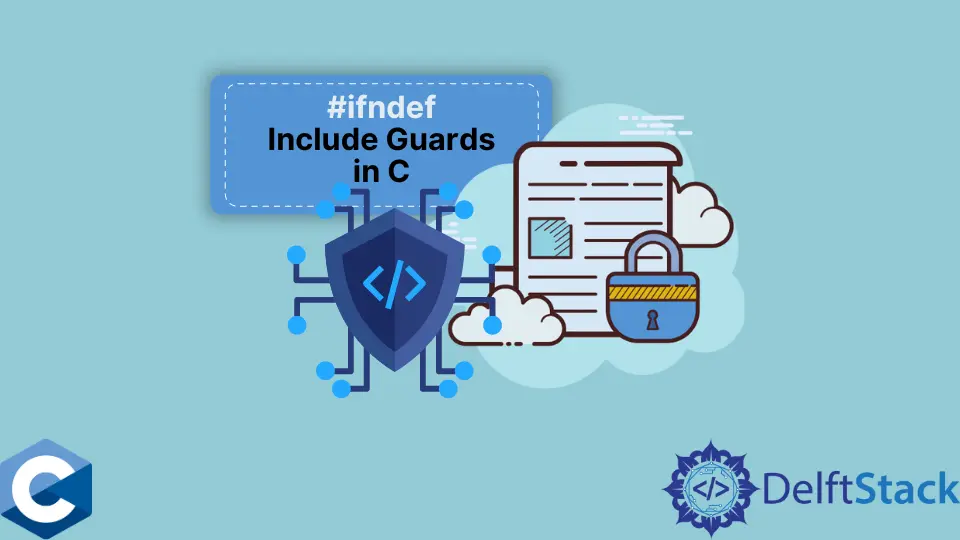
When working with C programming, one of the most critical practices is ensuring that your code is organized and free from compilation errors. A common issue arises when multiple files include the same header file, leading to redefinition errors. This is where #ifndef
include guards come into play.
This article will guide you through the concept of include guards in C, specifically focusing on the #ifndef
directive. By the end, you will understand how to implement these guards effectively, preventing potential issues in your code. Let’s dive into this essential aspect of C programming!
Understanding Include Guards
Include guards are preprocessor directives used in C and C++ to prevent the multiple inclusion of header files. When a header file is included multiple times in a single compilation unit, it can lead to redefinition errors. The #ifndef
directive, which stands for “if not defined,” is used to check if a specific macro is defined. If it is not defined, the code within the #ifndef
block will be included during compilation. This ensures that the header file is only processed once, safeguarding against duplicate definitions.
Here’s how a typical include guard looks:
#ifndef MY_HEADER_H
#define MY_HEADER_H
// Your header file content goes here
#endif // MY_HEADER_H
In this example, MY_HEADER_H
is a unique identifier for the header file. If MY_HEADER_H
is not defined, the preprocessor will include the content between #ifndef
and #endif
. Once it is included, MY_HEADER_H
is defined, preventing any further inclusion of the same header file.
Implementing #ifndef Include Guards
To implement #ifndef
include guards in your C projects, follow these steps:
-
Choose a Unique Identifier: The identifier should be unique to avoid conflicts with other header files. A common practice is to use the header file name in uppercase.
-
Wrap Your Header Content: Surround the content of your header file with the
#ifndef
,#define
, and#endif
directives. -
Compile and Test: After adding the include guards, compile your project to ensure that there are no redefinition errors.
Here’s an example of how to implement include guards in a header file named example.h
:
#ifndef EXAMPLE_H
#define EXAMPLE_H
void exampleFunction();
#endif // EXAMPLE_H
In this code, EXAMPLE_H
is the unique identifier. If example.h
is included in multiple source files, the function prototype for exampleFunction
will only be declared once, preventing any redefinition issues.
By using this method, you ensure that your header files are included only once per compilation unit, promoting better organization and reducing the risk of errors in your codebase.
Best Practices for Using Include Guards
While using #ifndef
include guards is essential, adhering to best practices can further enhance your coding experience. Here are some tips:
-
Use Descriptive Identifiers: Make your identifiers descriptive enough to indicate the content of the header file. This practice helps in avoiding conflicts and makes your code more readable.
-
Consistent Naming Conventions: Stick to a consistent naming convention across your project. For instance, if you use uppercase letters and underscores for identifiers, maintain that style throughout.
-
Avoid Nested Includes: If possible, avoid including headers within headers. This can lead to complex dependencies and make your code harder to manage.
-
Document Your Headers: Adding comments to your header files can help other developers understand the purpose of the file and its functions.
Here’s an example of a header file following these best practices:
#ifndef MY_LIBRARY_H
#define MY_LIBRARY_H
void initializeLibrary();
void cleanupLibrary();
#endif // MY_LIBRARY_H
In this example, MY_LIBRARY_H
clearly indicates that it belongs to a library, and the function names are descriptive of their purpose. This clarity aids in maintaining the code in the long run.
By following these best practices, you can create a more maintainable and error-free codebase, making your C programming journey smoother.
Conclusion
Incorporating #ifndef
include guards in your C programming is a simple yet effective way to prevent redefinition errors and enhance code organization. By understanding how to implement these guards and following best practices, you will not only write cleaner code but also foster a more collaborative environment for future development. Remember, the goal is to make your code as maintainable and error-free as possible. So, make include guards a part of your coding routine, and watch your C projects thrive!
FAQ
- What are include guards in C?
Include guards are preprocessor directives that prevent multiple inclusions of the same header file, avoiding redefinition errors during compilation.
-
How do I create an include guard?
Use the#ifndef
,#define
, and#endif
directives in your header file to create an include guard. -
Why are include guards important?
They prevent compilation errors caused by multiple inclusions of the same header file, ensuring that your code compiles correctly. -
Can I use include guards in C++?
Yes, include guards are also used in C++ and work in the same way as in C. -
What happens if I forget to use include guards?
Forgetting to use include guards can lead to redefinition errors, making your code fail to compile.
#ifndef include guards in C programming. Learn the importance of include guards, best practices, and effective implementation methods to prevent redefinition errors in your code. Enhance your coding skills and ensure a cleaner, more organized codebase with our comprehensive guide.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook