How to Find System Hostname in C
-
Use the
gethostname
Function to Find System Hostname in C -
Use the
uname
Function to Find System Hostname in C
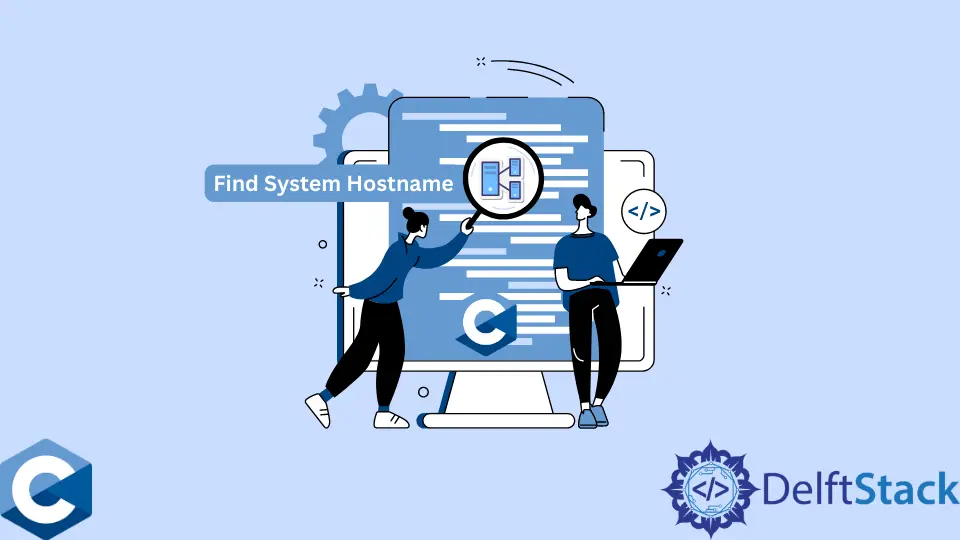
This article will explain several methods of how to find system hostname in C.
Use the gethostname
Function to Find System Hostname in C
The gethostname
function is part of the POSIX specification, and it’s used to access the system hostname. The function takes two arguments: char*
pointing to a buffer where retrieved hostname will be stored and the number of bytes denoting the buffer’s length. The function returns zero on success, and -1 is returned on error. Note that POSIX systems are likely to have a maximum number of bytes defined for hostname length, so the user should allocate the big enough buffer to store the retrieved value.
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
enum { MAX_SIZE = 256 };
int main(void) {
int ret;
char hostname[MAX_SIZE];
ret = gethostname(&hostname[0], MAX_SIZE);
if (ret == -1) {
perror("gethostname");
exit(EXIT_FAILURE);
}
printf("Hostname: %s\n", hostname);
exit(EXIT_SUCCESS);
}
Output:
Hostname: lenovo-pc
The previous example demonstrates the basic call to the gethostname
function, but one should always implement error reporting routines to ensure fail-safe code and informative logging messages. Since the gethostname
sets errno
values, we can evaluate it in a switch
statement and print the corresponding messages to the stderr
stream.
#include <errno.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
enum { MAX_SIZE = 256 };
int main(void) {
int ret;
char hostname[MAX_SIZE];
errno = 0;
ret = gethostname(&hostname[0], MAX_SIZE);
if (ret == -1) {
switch (errno) {
case EFAULT:
fprintf(stderr, "'name' argument is an invalid address");
break;
case EINVAL:
fprintf(stderr, "'len' argument is negative");
break;
default:
perror("gethostname");
}
exit(EXIT_FAILURE);
}
printf("Hostname: %s\n", hostname);
exit(EXIT_SUCCESS);
}
Output:
Hostname: lenovo-pc
Use the uname
Function to Find System Hostname in C
Alternatively, one can utilize the uname
function call to find the system hostname. uname
can generally retrieve several data points about the system, namely the operating system name, operating system release date, and version, Hardware identifier, and hostname. Notice though, uname
stores the above information in a utsntheame
structure defined in <sys/utsname.h>
header file. The function takes the pointer to the utsname
structure and returns the zero value on a successful call. uname
also sets errno
on failure, and EFAULT
value is defined as an indication when the passed pointer to a utsname
structure is invalid.
#include <stdio.h>
#include <stdlib.h>
#include <sys/utsname.h>
enum { MAX_SIZE = 256 };
int main(void) {
struct utsname uts;
if (uname(&uts) == -1) perror("uname");
printf("Node name: %s\n", uts.nodename);
printf("System name: %s\n", uts.sysname);
printf("Release: %s\n", uts.release);
printf("Version: %s\n", uts.version);
printf("Machine: %s\n", uts.machine);
exit(EXIT_SUCCESS);
}
Output:
Hostname: lenovo-pc
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook