How to Get User Input in C
-
Use the
scanf
Function to Get User Input According to Given Format in C -
Use
scanf
Parse User Input Based on the Given Formatting
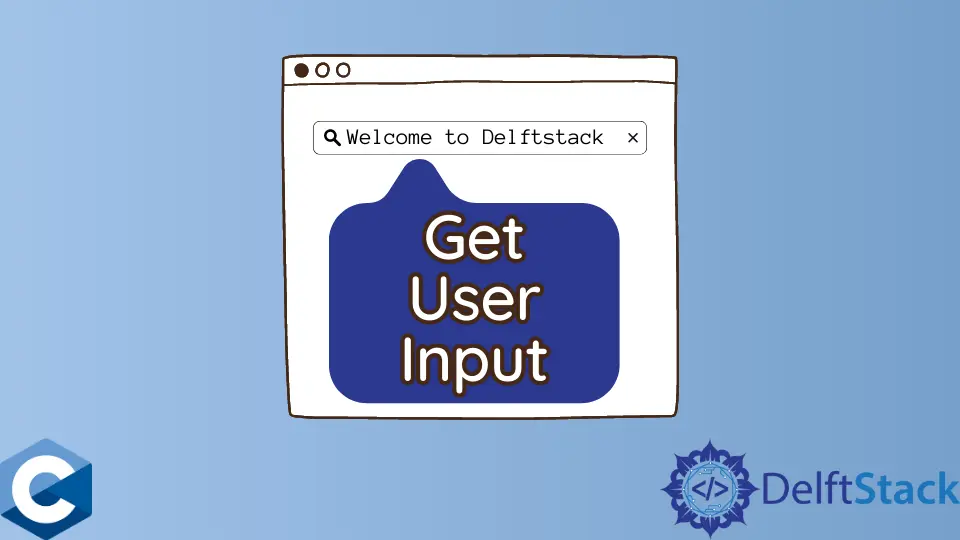
This article will demonstrate multiple methods about how to get the user input in C.
Use the scanf
Function to Get User Input According to Given Format in C
The scanf
function processes the user input as the formatted text and stores the converted string value in the given pointer. The function prototype is similar to printf
family of functions. It takes format string argument as a directive on how to process the input characters and then a variable number of pointer arguments to store corresponding values.
Note that, %[^\n]
specifier directs the scanf
to process every character before the first newline character as one string and store it to the char*
buffer. The destination buffer should be big enough for the user input string.
Also, an optional character m
can be used in the string conversion specifiers, and it will force the function to allocate a sufficient buffer size to store the input string. Thus, the user would need to call the free
function after the given pointer is no longer needed.
#include <stdio.h>
#include <stdlib.h>
int main(void) {
char str1[1000];
printf("Input the text: ");
scanf("%[^\n]", str1); // takes everything before '\n'
printf("'%s'\n", str1);
exit(EXIT_SUCCESS);
}
Output:
Input the text: temp string to be processed
'temp string to be processed'
Alternatively, we can utilize scanf
to process any text input before the given character by modifying the format string specifier. The following example shows the scanf
call that scans the user input until the first character of value 9
is found.
#include <stdio.h>
#include <stdlib.h>
int main(void) {
char str1[1000];
printf("Input the text: ");
scanf(" %[^9]*", str1); // takes everything before '9' in string
printf("'%s'\n", str1);
exit(EXIT_SUCCESS);
}
Output:
Input the text: temporary string 32j2 923mlk
'temporary string 32j2 '
Use scanf
Parse User Input Based on the Given Formatting
Another useful feature of the scanf
function is to parse the user input based on the given format. *
character is used in the format string to discard the matching the characters by following conversion specifier.
The next code sample demonstrates when the scanf
parses text inputs consisting of mandatory :
symbol and only store characters after the given symbol to the end of the line. This option might be useful for scanning the fixed format texts where certain characters are present at delimiter positions.
#include <stdio.h>
#include <stdlib.h>
int main(void) {
char str1[1000];
printf("Input the text: ");
scanf("%*[^:]%*c%[^\n]", str1);
printf("'%s'\n", str1);
exit(EXIT_SUCCESS);
}
Output:
Input the text: temporary value of var3: 324 gel
' 324 gel'
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook