How to Read File Line by Line Using fscanf in C
-
Use the
fscanf
Function to Read File Line by Line in C -
Use the
fscanf
Function to Read File Word by Word in C
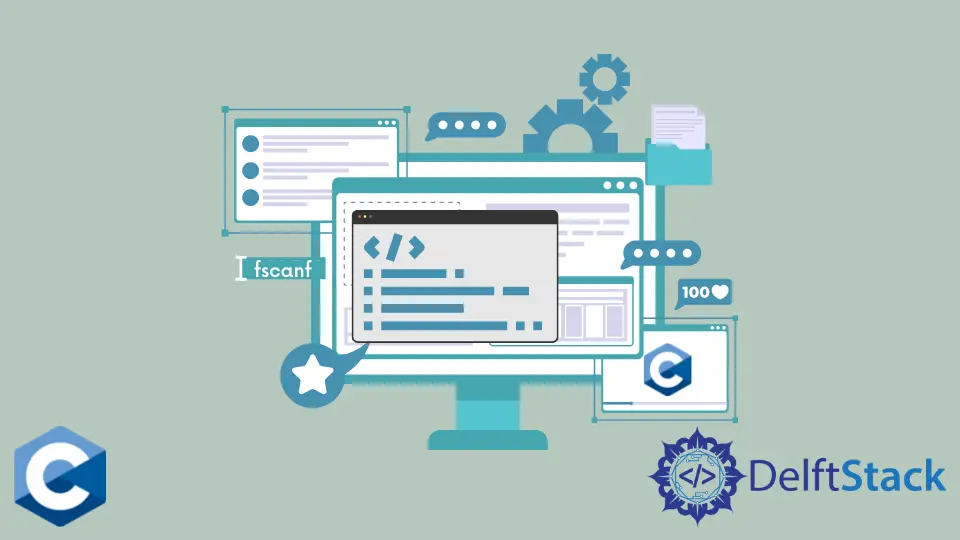
This article will explain several methods of how to read a file line by line using fscanf
in C.
Use the fscanf
Function to Read File Line by Line in C
The fscanf
function is part of the C standard library formatted input utilities. Multiple functions are provided for different input sources like scanf
to read from stdin
, sscanf
to read from the character string, and fscanf
to read from the FILE
pointer stream. The latter can be used to read the regular file line by line and store them in the buffer.
fscanf
takes formatting specification similar to the printf
specifiers, and all of them are listed in full detail on this page. In the following example, we open the sample input file using the fopen
function call and allocate memory of full file size to store the read stream into it. "%[^\n] "
format string is specified to read file stream until the new line character is encountered. fscanf
returns EOF
when the end of the input is reached; thus we iterate with while
loop and print each line one by one.
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
const char *filename = "input.txt";
int main(int argc, char *argv[]) {
FILE *in_file = fopen(filename, "r");
struct stat sb;
stat(filename, &sb);
char *file_contents = malloc(sb.st_size);
while (fscanf(in_file, "%[^\n] ", file_contents) != EOF) {
printf("> %s\n", file_contents);
}
fclose(in_file);
exit(EXIT_SUCCESS);
}
Output:
> Temporary string to be written to file
Note that, even though the previous code will most likely run successfully if the input file name exists in the filesystem, but multiple function calls can fail nevertheless and terminate the program abnormally. The next example code is the modified version, where the error checking routines are implemented.
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
const char *filename = "input.txt";
int main(int argc, char *argv[]) {
FILE *in_file = fopen(filename, "r");
if (!in_file) {
perror("fopen");
exit(EXIT_FAILURE);
}
struct stat sb;
if (stat(filename, &sb) == -1) {
perror("stat");
exit(EXIT_FAILURE);
}
char *file_contents = malloc(sb.st_size);
while (fscanf(in_file, "%[^\n] ", file_contents) != EOF) {
printf("> %s\n", file_contents);
}
fclose(in_file);
exit(EXIT_SUCCESS);
}
Output:
> Temporary string to be written to file
Use the fscanf
Function to Read File Word by Word in C
Another useful case to utilize the fscanf
function is to traverse the file and parse every space-separated token. Note that the only thing to be changed from the previous example is the format specifier to "%[^\n ] "
. stat
system call is to retrieve the file size, and the value is used to pass as the malloc
argument to allocate the buffer. This method may be wasteful for some scenarios, but it ensures that even the largest single-line files can be stored in the buffer.
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
const char *filename = "input.txt";
int main(int argc, char *argv[]) {
FILE *in_file = fopen(filename, "r");
if (!in_file) {
perror("fopen");
exit(EXIT_FAILURE);
}
struct stat sb;
if (stat(filename, &sb) == -1) {
perror("stat");
exit(EXIT_FAILURE);
}
char *file_contents = malloc(sb.st_size);
while (fscanf(in_file, "%[^\n ] ", file_contents) != EOF) {
printf("> %s\n", file_contents);
}
fclose(in_file);
exit(EXIT_SUCCESS);
}
Output:
> Temporary
> string
> to
> be
> written
> to
> file
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook