How to Use the fork Function in C
- What is the fork Function?
- Understanding Process Creation
- Handling Process Termination
- Conclusion
- FAQ
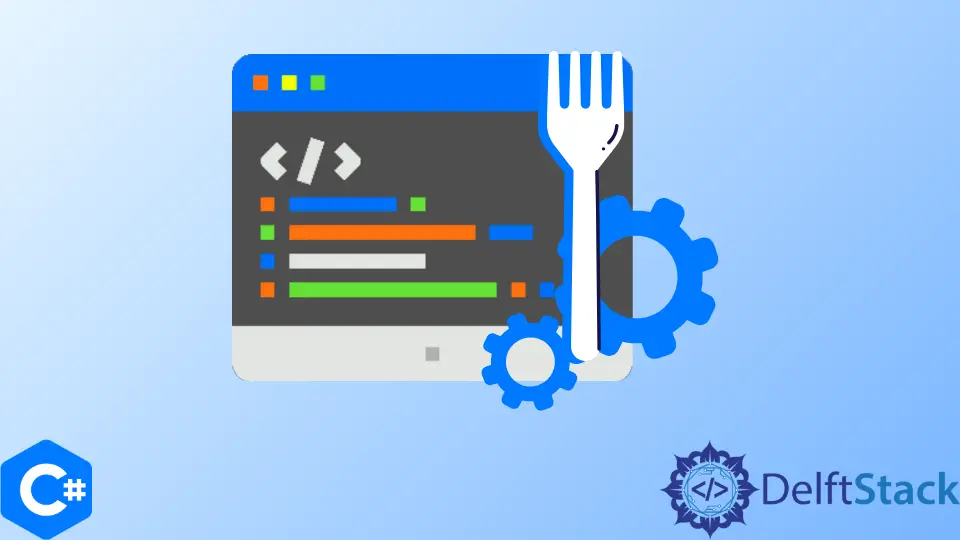
The fork
function in C is a powerful tool that allows you to create new processes by duplicating the existing one. This is a fundamental concept in Unix-like operating systems, enabling multitasking and process management. Understanding how to use fork
can significantly enhance your programming skills, especially when working on applications that require concurrent execution.
In this article, we will delve into the details of the fork
function, demonstrating its usage with clear examples. Whether you’re a beginner or looking to refine your skills, this guide will provide you with the knowledge needed to effectively implement process creation in your C programs.
What is the fork Function?
The fork
function is defined in the unistd.h
header file and is used to create a new process. When fork
is called, it creates a child process that is an exact duplicate of the parent process. The child process receives a unique process ID (PID), which can be used to distinguish between the two processes. The return value of fork
helps determine whether the code is executing in the parent or child process. The parent receives the child’s PID, while the child receives a return value of 0.
Here’s a simple example to illustrate how fork
works:
cCopy#include <stdio.h>
#include <unistd.h>
int main() {
pid_t pid = fork();
if (pid < 0) {
perror("Fork failed");
return 1;
} else if (pid == 0) {
printf("This is the child process with PID: %d\n", getpid());
} else {
printf("This is the parent process with PID: %d and child PID: %d\n", getpid(), pid);
}
return 0;
}
Output:
textCopyThis is the parent process with PID: 1234 and child PID: 1235
This is the child process with PID: 1235
In this example, we first include the necessary headers. The fork
function is called, and based on its return value, we determine whether we are in the parent or child process. The parent prints its PID along with the child’s PID, while the child simply prints its own PID. This demonstrates the basic functionality of fork
in creating processes.
Understanding Process Creation
When you call fork
, the operating system creates a new process. This involves copying the entire memory space of the parent process to the child process. Although they share the same code, data, and stack segments initially, any changes made in one process do not affect the other. This behavior is known as copy-on-write, which optimizes memory usage.
The fork
function is crucial in scenarios where concurrent execution is needed. For example, web servers often use fork
to handle multiple requests simultaneously, allowing them to serve multiple clients without blocking.
Here’s another example that demonstrates how to create multiple child processes:
cCopy#include <stdio.h>
#include <unistd.h>
int main() {
for (int i = 0; i < 3; i++) {
pid_t pid = fork();
if (pid < 0) {
perror("Fork failed");
return 1;
} else if (pid == 0) {
printf("Child %d with PID: %d\n", i + 1, getpid());
return 0; // Child exits
}
}
// Parent process waits for all children to finish
for (int i = 0; i < 3; i++) {
wait(NULL);
}
printf("All child processes have finished.\n");
return 0;
}
Output:
textCopyChild 1 with PID: 1236
Child 2 with PID: 1237
Child 3 with PID: 1238
All child processes have finished.
In this code, we loop three times to create three child processes. Each child prints its own PID. The parent process waits for all children to complete before printing a final message. This example showcases how to manage multiple processes effectively.
Handling Process Termination
When a child process finishes executing, it can either terminate normally or be terminated by the parent. It’s essential for the parent to handle the termination of its children to avoid zombie processes, which occur when a child process has finished executing but still has an entry in the process table.
You can use the wait
system call to wait for a child process to terminate. Here’s how you can modify the previous example to include process termination handling:
cCopy#include <stdio.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
for (int i = 0; i < 3; i++) {
pid_t pid = fork();
if (pid < 0) {
perror("Fork failed");
return 1;
} else if (pid == 0) {
printf("Child %d with PID: %d\n", i + 1, getpid());
return 0; // Child exits
}
}
// Parent process waits for all children to finish
for (int i = 0; i < 3; i++) {
int status;
wait(&status);
printf("A child process has finished with status: %d\n", status);
}
printf("All child processes have finished.\n");
return 0;
}
Output:
textCopyChild 1 with PID: 1239
Child 2 with PID: 1240
Child 3 with PID: 1241
A child process has finished with status: 0
A child process has finished with status: 0
A child process has finished with status: 0
All child processes have finished.
In this modified example, the parent process waits for each child to finish and prints their termination status. This practice is crucial for resource management and ensures that no orphan or zombie processes remain.
Conclusion
The fork
function is an essential aspect of process management in C programming. By understanding how to create and manage child processes, you can develop applications that utilize concurrent execution effectively. Whether you’re building a server or a simple multitasking application, mastering fork
will significantly enhance your programming toolkit.
In this article, we’ve covered the basics of fork
, how to handle process termination, and provided examples to illustrate these concepts. With this knowledge, you’re well on your way to creating robust applications that can handle multiple tasks simultaneously.
FAQ
-
What does the
fork
function return?
Thefork
function returns a positive PID to the parent process, 0 to the child process, and a negative value if the fork fails. -
How can I prevent zombie processes?
You can prevent zombie processes by using thewait
system call in the parent process to wait for the child processes to terminate. -
Can I use
fork
in a loop?
Yes, you can usefork
in a loop to create multiple child processes, but ensure you handle their termination properly. -
What happens to the child process when the parent exits?
If the parent process exits before the child, the child process becomes an orphan and is adopted by the init process. -
Is
fork
available in Windows?
Thefork
function is primarily a Unix/Linux feature. Windows has its own methods for process creation, such asCreateProcess
.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook