For Each Loop in C
- Understanding the Basics of Looping in C
-
Using a
for
Loop to Simulate Foreach Behavior -
Using a
while
Loop for Iteration - Creating a Custom Foreach Function
- Conclusion
- FAQ
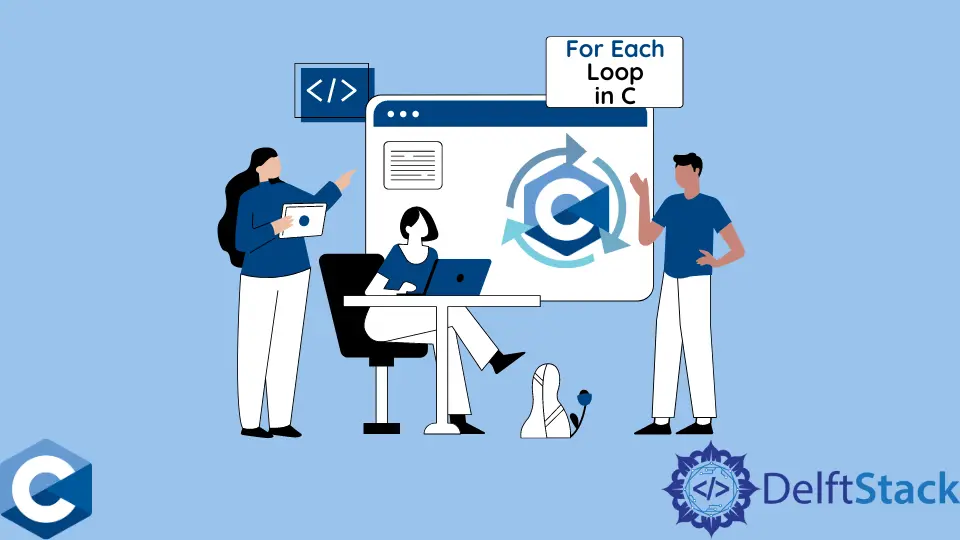
When it comes to programming in C, one question often arises: does C support a foreach loop? This is an interesting topic because many modern programming languages, such as Python and JavaScript, include a foreach construct that simplifies the iteration over collections. However, C, being a lower-level language, does not have a built-in foreach loop. Instead, C programmers typically use traditional for loops or while
loops to achieve similar functionality.
In this article, we will delve into the mechanisms available in C for iterating over arrays and collections. We will also explore how to implement a foreach-like behavior using other constructs, providing you with a clearer understanding of how to work with collections in C.
Understanding the Basics of Looping in C
Before diving into alternatives to the foreach loop, it’s essential to grasp the basic looping constructs available in C. The most common loops in C are the for loop, while
loop, and do-while loop. Each of these has its specific use cases, but none directly replicate the functionality of a foreach loop.
A for loop is typically used when the number of iterations is known beforehand. It consists of three parts: initialization, condition, and increment. The while
loop, on the other hand, continues to execute as long as a specified condition is true. The do-while loop is similar to the while
loop but guarantees at least one execution of the loop body, as the condition is checked after the loop’s body is executed.
Using a for
Loop to Simulate Foreach Behavior
While C does not have a foreach loop, you can simulate its behavior using a for loop. This method allows you to iterate over an array or collection in a straightforward manner.
Here’s a simple example of using a for loop to iterate through an array of integers:
#include <stdio.h>
int main() {
int numbers[] = {10, 20, 30, 40, 50};
int length = sizeof(numbers) / sizeof(numbers[0]);
for (int i = 0; i < length; i++) {
printf("%d\n", numbers[i]);
}
return 0;
}
Output:
10
20
30
40
50
In this example, we declare an array of integers called numbers
. We calculate the length of the array by dividing the total size of the array by the size of a single element. The for loop then iterates through each index of the array, printing each number to the console. This mimics the behavior of a foreach loop by allowing you to access each element in the collection sequentially.
Using a while
Loop for Iteration
Another way to achieve a foreach-like iteration in C is by using a while
loop. This method can be particularly useful when you do not know the number of iterations beforehand or when dealing with dynamic data structures.
Here’s how you can use a while
loop to iterate through an array:
#include <stdio.h>
int main() {
int numbers[] = {10, 20, 30, 40, 50};
int length = sizeof(numbers) / sizeof(numbers[0]);
int index = 0;
while (index < length) {
printf("%d\n", numbers[index]);
index++;
}
return 0;
}
Output:
10
20
30
40
50
In this example, we initialize an index variable to zero. The while
loop continues as long as the index is less than the length of the array. Inside the loop, we print the current element and then increment the index. This approach is flexible and can be adapted to work with different data structures, such as linked lists or dynamically allocated arrays.
Creating a Custom Foreach Function
If you want to encapsulate the foreach functionality, you can create a custom function that takes a function pointer as an argument. This allows you to define what action to perform on each element of the array.
Here’s an example of a custom foreach function in C:
#include <stdio.h>
void foreach(int *array, int length, void (*func)(int)) {
for (int i = 0; i < length; i++) {
func(array[i]);
}
}
void printElement(int element) {
printf("%d\n", element);
}
int main() {
int numbers[] = {10, 20, 30, 40, 50};
int length = sizeof(numbers) / sizeof(numbers[0]);
foreach(numbers, length, printElement);
return 0;
}
Output:
10
20
30
40
50
In this code, we define a foreach
function that accepts an array, its length, and a function pointer. The printElement
function is passed as an argument to foreach
, which prints each element of the array. This method provides a more modular approach, allowing you to define different operations without modifying the iteration logic.
Conclusion
While C does not have a built-in foreach loop, it offers several constructs that allow for similar functionality. By using for loops, while
loops, or creating custom functions, C programmers can effectively iterate over collections and perform operations on each element. Understanding these alternatives not only enhances your programming skills but also deepens your appreciation for the flexibility and power of the C language. Whether you’re working on a small project or a large application, mastering these looping techniques will serve you well.
FAQ
-
Does C have a built-in foreach loop?
C does not have a built-in foreach loop. Instead, you can use for loops orwhile
loops to iterate over collections. -
Can I create a custom foreach function in C?
Yes, you can create a custom function that mimics the foreach behavior by accepting an array and a function pointer to perform operations on each element. -
What is the difference between for and
while
loops in C?
A for loop is typically used when the number of iterations is known, while awhile
loop continues executing as long as a specified condition is true. -
How can I iterate over a linked list in C?
You can use awhile
loop to traverse a linked list by starting from the head and moving to the next node until you reach the end. -
Is it possible to use a foreach-like construct in C with function pointers?
Yes, by creating a custom foreach function that accepts a function pointer, you can achieve a similar effect to a foreach loop.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn