How to Flush stdout Output Stream in C
- Understanding Output Buffering in C
- Method 1: Using fflush
- Method 2: Using setbuf
- Method 3: Using setvbuf
- Conclusion
- FAQ
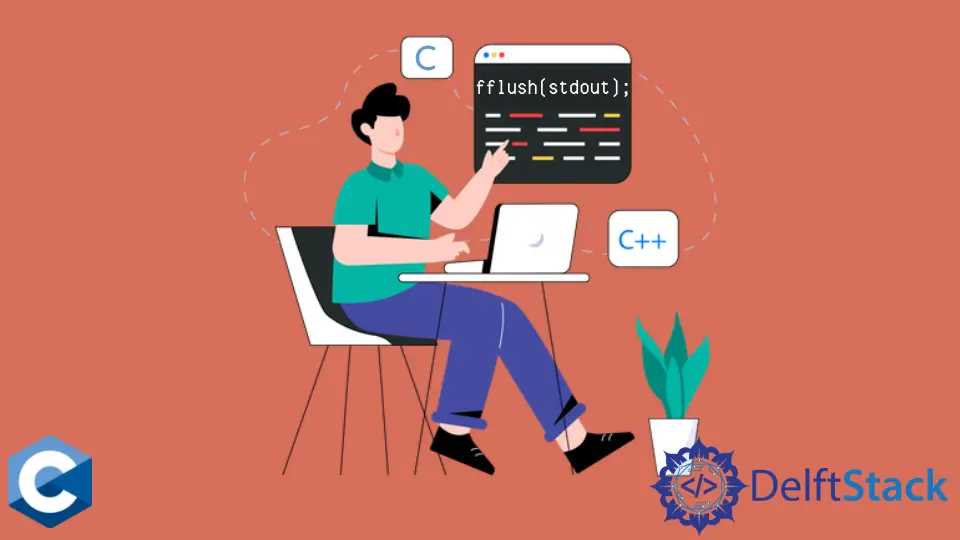
Flushing the stdout
output stream in C is a crucial task, especially when dealing with buffered output. When you print data to the console, it doesn’t always appear immediately. This can be problematic in scenarios where real-time feedback is essential, such as in interactive applications or when debugging.
In this article, we’ll explore the methods to flush the stdout
stream effectively, ensuring that your output reaches its destination promptly. Whether you’re a beginner or an experienced programmer, understanding how to manage output streams can significantly enhance your coding efficiency. Let’s dive into the various techniques available for flushing stdout
in C.
Understanding Output Buffering in C
Before we get into the specifics of flushing stdout
, it’s important to understand how output buffering works in C. When you use functions like printf
, the output is stored in a buffer rather than being sent directly to the console. This buffering improves performance by reducing the number of system calls. However, if you want to see the output immediately, you need to flush the buffer.
The C standard library provides several functions to manage output buffers. By default, stdout
is line-buffered if it is connected to a terminal, meaning the buffer is flushed when a newline character is encountered. However, if you’re working with files or pipes, you may need to flush the buffer manually.
Method 1: Using fflush
One of the most straightforward ways to flush the stdout
output stream is by using the fflush
function. This function forces the output buffer to be flushed, sending any buffered data to the console immediately.
Here’s a simple example to demonstrate this:
#include <stdio.h>
int main() {
printf("Hello, World!");
fflush(stdout);
return 0;
}
Output:
Hello, World!
In this code snippet, we first print “Hello, World!” to the console. By calling fflush(stdout)
, we ensure that the output is displayed immediately. This is particularly useful in programs where timing is critical, such as in user interfaces or when debugging.
The fflush
function can also be used with file streams, allowing for greater control over output. However, it’s essential to note that using fflush
on input streams is undefined behavior. Always use it with output streams to avoid unexpected results.
Method 2: Using setbuf
Another method to manage output buffering in C is through the setbuf
function. This function allows you to control whether the output is buffered and how it’s buffered. If you want to disable buffering entirely, you can set the buffer to NULL
.
Here’s how you can do this:
#include <stdio.h>
int main() {
setbuf(stdout, NULL);
printf("This will be displayed immediately.\n");
return 0;
}
In this example, calling setbuf(stdout, NULL)
disables buffering for the stdout
stream. As a result, every call to printf
will display output immediately. This can be beneficial in scenarios where you want to ensure that every piece of output is visible to the user without delay.
However, keep in mind that disabling buffering can impact performance, especially in programs that generate a lot of output. Use this method judiciously to maintain a balance between performance and immediate feedback.
Method 3: Using setvbuf
The setvbuf
function offers more advanced control over buffering compared to setbuf
. It allows you to specify the type of buffering (fully buffered, line buffered, or unbuffered) and even define a custom buffer. This function is particularly useful when you need fine-tuned control over output behavior.
Here’s a quick example:
#include <stdio.h>
int main() {
char buffer[1024];
setvbuf(stdout, buffer, _IOFBF, sizeof(buffer));
printf("Buffered output with custom buffer.\n");
fflush(stdout);
return 0;
}
Output:
Buffered output with custom buffer.
In this code, we create a custom buffer of 1024 bytes and set it as the buffer for stdout
using setvbuf
. The _IOFBF
parameter specifies that the output should be fully buffered. After printing the message, we call fflush(stdout)
to ensure that the output is displayed immediately.
Using setvbuf
can enhance performance in applications where output is generated in bulk, as it allows you to control how and when the buffer is flushed. However, be cautious when using custom buffers, as improper management can lead to memory issues.
Conclusion
Flushing the stdout
output stream in C is an essential skill for developers looking to manage console output effectively. By using functions like fflush
, setbuf
, and setvbuf
, you gain control over when and how your output appears on the screen. Whether you’re building interactive applications or debugging complex code, understanding these methods ensures that your output is timely and reliable. With practice, you can enhance your coding efficiency and improve the user experience in your applications.
FAQ
-
What does flushing the
stdout
output stream mean?
Flushing thestdout
output stream means forcing the buffered output to be sent to the console immediately. -
When should I use
fflush(stdout)
?
You should usefflush(stdout)
when you want to ensure that the output appears on the console right away, especially in interactive applications or during debugging. -
Is it safe to use
fflush
on input streams?
No, usingfflush
on input streams is considered undefined behavior in C. It should only be used with output streams. -
What is the difference between
setbuf
andsetvbuf
?
setbuf
simply allows you to set a buffer for a stream, whereassetvbuf
provides more control over the buffering mode and allows you to define a custom buffer. -
Can disabling buffering affect performance?
Yes, disabling buffering can lead to decreased performance, especially in programs that generate a lot of output, as each output operation requires a system call.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook