How to Use File Redirection in C
- Understanding File Redirection in C
- Redirecting Output to a File
- Redirecting Input from a File
- Error Handling in File Redirection
- Conclusion
- FAQ
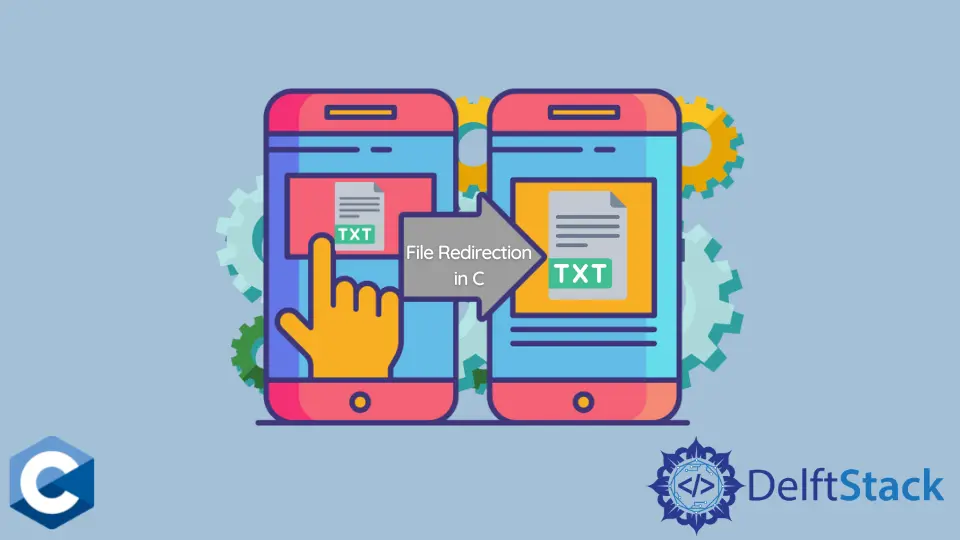
File redirection is a powerful feature in C programming that allows you to read from and write to files seamlessly. This capability enhances the way you interact with data, making your programs more versatile and efficient. Whether you are logging output for analysis or reading input from a file, understanding file redirection is crucial for any C programmer.
In this article, we will explore how to use file redirection in C, complete with code examples and explanations. By the end, you’ll be equipped with the knowledge to implement file redirection effectively in your C applications.
Understanding File Redirection in C
File redirection in C involves using file pointers and standard I/O functions to manage input and output streams. The standard streams in C include stdin
, stdout
, and stderr
. By redirecting these streams, you can control where your program reads input from or sends output to. This is particularly useful for applications that require data processing or logging.
In C, file redirection typically involves using the fopen
, fprintf
, fscanf
, and fclose
functions. Let’s dive into how you can implement file redirection step by step.
Redirecting Output to a File
Redirecting output to a file can be accomplished using the fopen
function to create or open a file, followed by using fprintf
to write data to that file. Here’s a simple example demonstrating this process:
#include <stdio.h>
int main() {
FILE *file = fopen("output.txt", "w");
if (file == NULL) {
printf("Error opening file!\n");
return 1;
}
fprintf(file, "Hello, World!\n");
fprintf(file, "This is a test of file redirection in C.\n");
fclose(file);
return 0;
}
Output:
The file "output.txt" will contain:
Hello, World!
This is a test of file redirection in C.
In this code, we first open a file named output.txt
in write mode using fopen
. If the file opens successfully, we proceed to write two lines of text into it using fprintf
. Finally, we close the file with fclose
. If the file cannot be opened, an error message is displayed. This method is straightforward and allows you to log data or save results from your program.
Redirecting Input from a File
Just as you can redirect output to a file, you can also redirect input from a file. This is useful when you want your program to read data without requiring user input interactively. Below is an example of how to read from a file:
#include <stdio.h>
int main() {
FILE *file = fopen("input.txt", "r");
if (file == NULL) {
printf("Error opening file!\n");
return 1;
}
char buffer[100];
while (fgets(buffer, sizeof(buffer), file)) {
printf("%s", buffer);
}
fclose(file);
return 0;
}
In this example, we open a file named input.txt
in read mode. If the file opens successfully, we read each line using fgets
and print it to the console. This method allows you to process large datasets stored in files without manual input, making it efficient for data analysis or batch processing tasks.
Error Handling in File Redirection
Error handling is an essential aspect of file operations in C. It ensures that your program behaves predictably even when something goes wrong, such as a missing file or insufficient permissions. Let’s see how to implement basic error handling in file redirection:
#include <stdio.h>
int main() {
FILE *file = fopen("data.txt", "r");
if (file == NULL) {
perror("Error opening file");
return 1;
}
int number;
while (fscanf(file, "%d", &number) == 1) {
printf("Read number: %d\n", number);
}
fclose(file);
return 0;
}
In this code, we use perror
to print a descriptive error message if the file cannot be opened. The fscanf
function is used to read integers from the file. This approach ensures that your program can gracefully handle errors, providing users with informative feedback instead of crashing unexpectedly.
Conclusion
File redirection in C is a vital skill that can significantly enhance your programming capabilities. By mastering the techniques of redirecting input and output, you can create more efficient and user-friendly applications. Whether you’re logging data or processing files, understanding how to manage file streams will elevate your programming skills. With the examples provided, you should feel confident in implementing file redirection in your own projects.
FAQ
-
What is file redirection in C?
File redirection in C is the process of using file pointers and standard I/O functions to manage input and output streams, allowing data to be read from or written to files. -
How do I redirect output to a file in C?
You can redirect output to a file using thefopen
function to open a file in write mode and then usingfprintf
to write data to that file. -
Can I read input from a file in C?
Yes, you can read input from a file using thefopen
function to open a file in read mode and then usingfgets
orfscanf
to read data from it. -
How can I handle errors when working with files in C?
You can handle errors by checking if the file pointer returned byfopen
isNULL
and using functions likeperror
to display descriptive error messages. -
What are the common functions used for file operations in C?
Common functions for file operations in C includefopen
,fclose
,fprintf
,fscanf
,fgets
, andfputs
.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook