The extern Keyword in C
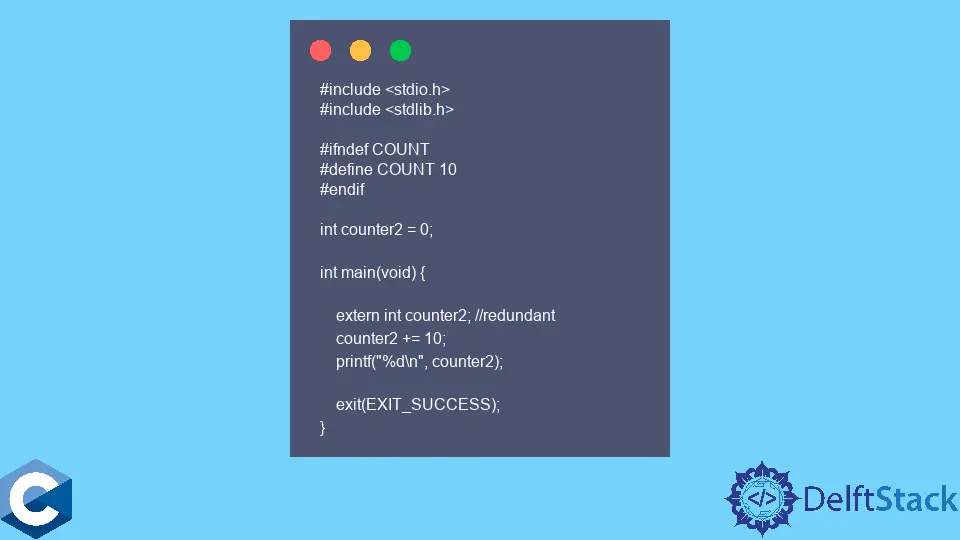
This article will demonstrate multiple methods about how to use the extern
keyword in C.
Use the extern
Keyword to Declare a Variable Defined in Other Files in C
Generally, the C language variables have 3 different linkage types: external linkage, internal linkage, or no linkage. If a variable is defined in a block or function scope, it’s considered to have no linkage. A variable that has the file scope can have internal or external linkage. Namely, if a global variable is declared with the static
qualifier, it has the internal linkage, implying that the variable can be used in a single source file (translation unit to be more precise). A global variable without a static
qualifier is considered to have external linkage and can be used in different source files. To use the global variable defined in some other source file, it should be declared using the keyword extern
.
#include <stdio.h>
#include <stdlib.h>
#ifndef COUNT
#define COUNT 10
#endif
extern int counter;
int main(void) {
printf("counter: ");
for (int i = 0; i < COUNT; ++i) {
counter += 1;
printf("%d, ", counter);
}
printf("\b\b \n");
exit(EXIT_SUCCESS);
}
Output:
counter: 1, 2, 3, 4, 5, 6, 7, 8, 9, 10
extern
qualified variables are usually defined somewhere in different files. Still, technically one can declare global variables in the same file with the extern
keyword, as shown in the following example. This type of declaration is essentially redundant because the compiler automatically makes variable names visible anywhere in the same file scope.
#include <stdio.h>
#include <stdlib.h>
#ifndef COUNT
#define COUNT 10
#endif
int counter2 = 0;
int main(void) {
extern int counter2; // redundant
counter2 += 10;
printf("%d\n", counter2);
exit(EXIT_SUCCESS);
}
Mind though, extern
declared variables should not be initialized as it leads to compiler warnings if we define const char*
global variable in one source file and initialize it with some string literal value. Declaring the same variable without const
qualifier in the different source file will compile without warnings, and it will remove the constness of the object. Thus, if the user tries to modify the read-only string tmp
, the compiler will not throw an error, but the program will hit the segmentation fault.
#include <stdio.h>
#include <stdlib.h>
#ifndef COUNT
#define COUNT 10
#endif
extern int counter;
// extern int counter = 3; // erroreous
extern char *tmp; // erroreous
int counter2 = 0;
int main(void) {
printf("counter: ");
for (int i = 0; i < COUNT; ++i) {
counter += 1;
printf("%d, ", counter);
}
printf("\b\b \n");
tmp[0] = 'S'; // segmentation fault
printf("%s", tmp);
exit(EXIT_SUCCESS);
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook