Exponents in C
- Understanding Exponentiation in C
-
Using the
pow()
Function - Calculating Integer Powers with a Loop
- Handling Negative Exponents
- Conclusion
- FAQ
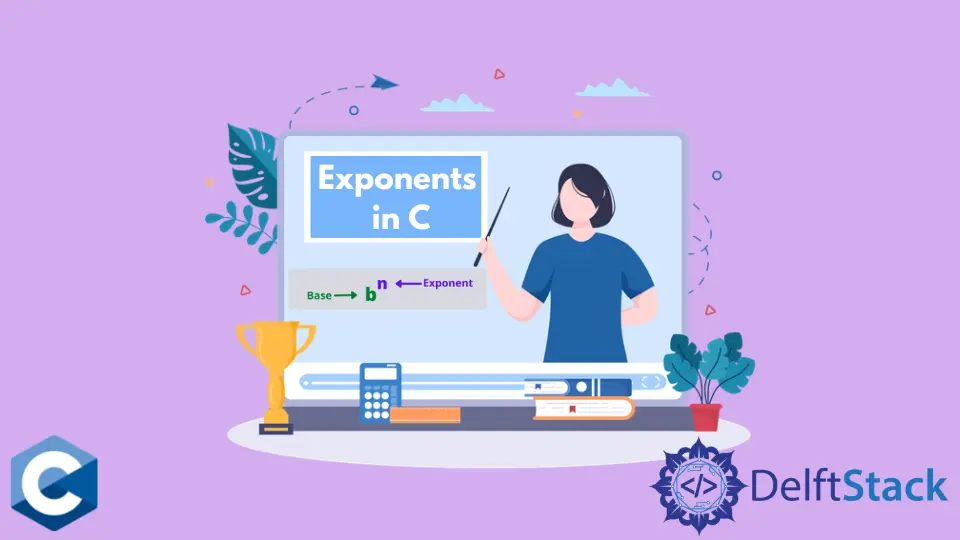
Exponentiation is a fundamental mathematical operation that finds its applications in various programming scenarios, including algorithm development, scientific calculations, and data analysis. In C programming, handling exponents can be done efficiently using built-in functions.
This article will introduce you to the world of exponentiation in C, showing you how to use functions like pow()
from the math library. Whether you want to calculate powers of integers or floating-point numbers, this guide will equip you with the knowledge you need to implement exponentiation effectively in your C programs.
Understanding Exponentiation in C
Before diving into the implementation, it’s essential to understand what exponentiation means. In simple terms, exponentiation is the operation of raising one number to the power of another. For example, in the expression (2^3), 2 is the base, and 3 is the exponent, resulting in 8. In C, you can achieve this using the pow()
function, which is part of the math library.
To use the pow()
function, you need to include the math header file at the beginning of your C program. The function takes two parameters: the base and the exponent, both of which can be floating-point values. The function returns the result as a double, making it suitable for a wide range of applications.
Using the pow()
Function
To perform exponentiation in C, the most common method is to use the pow()
function provided by the math library. Here’s how you can do it:
#include <stdio.h>
#include <math.h>
int main() {
double base = 2.0;
double exponent = 3.0;
double result = pow(base, exponent);
printf("%.2f raised to the power of %.2f is %.2f\n", base, exponent, result);
return 0;
}
Output:
2.00 raised to the power of 3.00 is 8.00
In this example, we include the math library with #include <math.h>
. We then declare our base and exponent as double variables. The pow()
function is called with these two variables, and the result is stored in another double variable named result
. Finally, we print the result using printf()
. This simple yet effective method allows you to calculate powers with ease.
Calculating Integer Powers with a Loop
If you prefer not to use the pow()
function or need a custom implementation, you can calculate the power of an integer using a loop. This method is particularly useful for educational purposes, as it helps you understand the underlying mechanics of exponentiation.
#include <stdio.h>
int main() {
int base = 2;
int exponent = 3;
int result = 1;
for (int i = 0; i < exponent; i++) {
result *= base;
}
printf("%d raised to the power of %d is %d\n", base, exponent, result);
return 0;
}
Output:
2 raised to the power of 3 is 8
In this code snippet, we are using a for
loop to calculate the power of an integer. We initialize result
to 1 and multiply it by the base in each iteration of the loop, which runs exponent
times. Finally, we print the result. This approach is straightforward and allows for a better understanding of how exponentiation works under the hood.
Handling Negative Exponents
Exponentiation isn’t limited to positive integers. In fact, negative exponents can represent fractions. To handle negative exponents in your C program, you can modify the previous loop method or continue using the pow()
function. Here’s how to do it using the pow()
function:
#include <stdio.h>
#include <math.h>
int main() {
double base = 2.0;
double exponent = -3.0;
double result = pow(base, exponent);
printf("%.2f raised to the power of %.2f is %.4f\n", base, exponent, result);
return 0;
}
Output:
2.00 raised to the power of -3.00 is 0.1250
In this example, we use the same pow()
function but with a negative exponent. The result is calculated as ( \frac{1}{2^3} ), which equals 0.125. This demonstrates how the pow()
function can seamlessly handle negative exponents, making it a versatile choice for exponentiation in C.
Conclusion
Exponentiation is a crucial operation in programming, and C provides robust methods to handle it effectively. Whether you choose to use the built-in pow()
function or implement your own logic with loops, understanding how to work with exponents will enhance your programming skills. By mastering these techniques, you can tackle a wide range of mathematical problems in your C projects.
FAQ
-
What is the
pow()
function in C?
Thepow()
function is a built-in function in the math library that calculates the power of a base raised to an exponent. -
Do I need to include any libraries to use exponentiation in C?
Yes, you need to include the math library by using#include <math.h>
at the beginning of your program. -
Can I use negative exponents in C?
Yes, you can use negative exponents with thepow()
function, and it will return the correct fractional result. -
Is there a way to calculate powers without using the
pow()
function?
Yes, you can use a loop to multiply the base by itself for the number of times specified by the exponent. -
What data types can I use with the
pow()
function?
Thepow()
function accepts both integer and floating-point values for the base and exponent, but it returns a double.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook