How to Dynamically Allocate an Array in C
-
Use the
malloc
Function to Allocate an Array Dynamically in C -
Use the
realloc
Function to Modify the Already Allocated Memory Region in C - Use Macro To Implement Allocation for Array of Given Objects in C
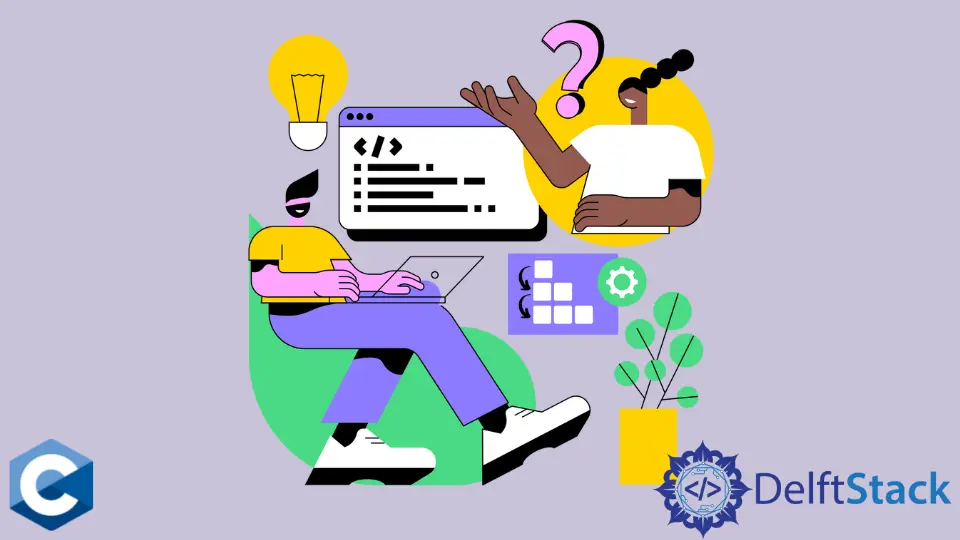
This article will demonstrate multiple methods of how to allocate an array dynamically in C.
Use the malloc
Function to Allocate an Array Dynamically in C
malloc
function is the core function for allocating the dynamic memory on the heap. It allocates the given number of bytes and returns the pointer to the memory region. Thus, if one wants to allocate an array of certain object types dynamically, a pointer to the type should be declared at first. Next, malloc
should be called by passing the number of elements multiplied by the single object’s size as an argument.
In the following example, we allocate the memory to store a character string. errno
is set to 0 as required by the secure coding standard, and the pointer returned from the malloc
call is checked to verify the successful execution of the function. Finally, the memmove
function is utilized to copy the string to the allocated memory location.
#include <errno.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define SIZE 100
const char *str = "random string to be moved";
int main() {
char *arr = NULL;
errno = 0;
arr = malloc(SIZE * sizeof(char));
if (!arr) {
perror("malloc");
exit(EXIT_FAILURE);
}
memmove(arr, str, strlen(str));
printf("arr: %s\n", arr);
free(arr);
exit(EXIT_SUCCESS);
}
Output:
arr: random string to be moved
Use the realloc
Function to Modify the Already Allocated Memory Region in C
The realloc
function is used to modify the memory region’s size previously allocated by the malloc
call. It takes the original memory address and the new size as the second argument. Note that realloc
may return the same pointer as passed or a different one based on the size requested and the available memory after the given address. Meanwhile, the contents of the previous array will be unchanged up to a newly specified size.
#include <errno.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define SIZE 100
const char *str = "random string to be moved";
int main() {
char *arr = NULL;
errno = 0;
arr = malloc(SIZE);
if (!arr) {
perror("malloc");
exit(EXIT_FAILURE);
}
int num = 102; // User Provided Value
for (int i = 0; i < num; ++i) {
if (i > SIZE) {
arr = realloc(arr, 2 * SIZE);
if (!arr) {
perror("realloc");
exit(EXIT_FAILURE);
}
}
arr[i] = 'a';
}
free(arr);
exit(EXIT_SUCCESS);
}
Use Macro To Implement Allocation for Array of Given Objects in C
Usually, malloc
is used to allocate an array of some user-defined structures. Since the malloc
returns the void
pointer and can be implicitly cast to any other type, a better practice is to cast the returned pointer to the corresponding type explicitly. Because it’s relatively easy to miss things and not include proper notation, so we implemented a macro expression that takes the number of elements in the array and the object type to automatically construct the correct malloc
statement, including the proper cast.
#include <errno.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define SIZE 100
typedef enum {
Jan,
Feb,
MAR,
APR,
MAY,
JUN,
JUL,
AUG,
SEP,
OCT,
NOV,
DEC
} month;
typedef struct {
unsigned char dd;
month mm;
unsigned yy;
} date;
#define MALLOC_ARRAY(number, type) ((type *)malloc((number) * sizeof(type)))
int main() {
date *d = NULL;
errno = 0;
d = MALLOC_ARRAY(SIZE, date);
if (!d) {
perror("malloc");
exit(EXIT_FAILURE);
}
free(d);
exit(EXIT_SUCCESS);
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook