The dup2 Function in C
-
Use the
dup2
Function to Duplicate a File Descriptor in C -
Use the
dup
Function to Duplicate a File Descriptor in C
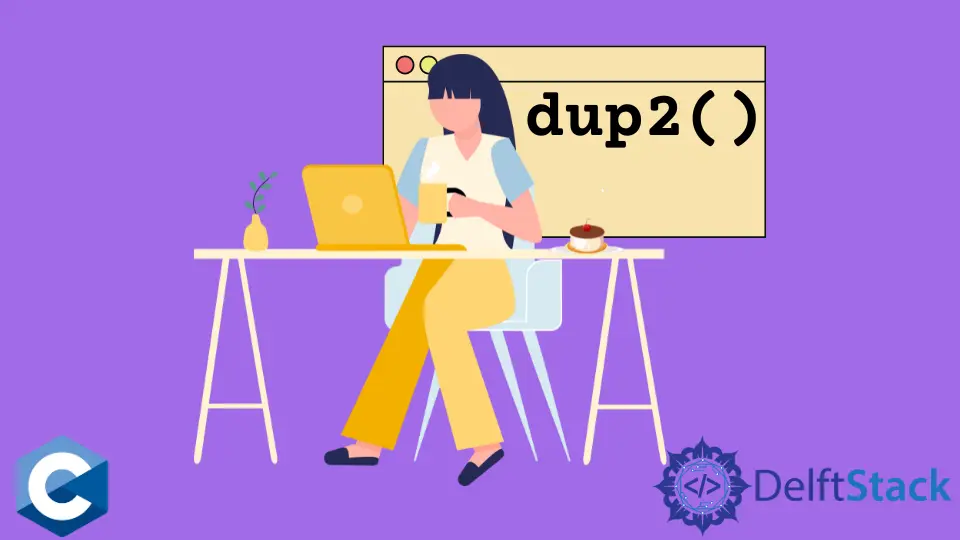
This article will explain several methods of how to use the dup2
function in C.
Use the dup2
Function to Duplicate a File Descriptor in C
Files are usually manipulated after they have been opened using the open
system call. On success, open
returns a new file descriptor associated with the newly opened file. In Unix-based systems, the operating system maintains a list of open files for each running program, called a file table. Each entry is represented using the int
type integer. These integers are called file descriptors in these systems,, and many system calls take file descriptor values as parameters.
Every running program has three open file descriptors by default when the process is created unless they choose to close them explicitly. dup2
function creates a copy of the given file descriptor and assigns a new integer to it. dup2
takes an old file descriptor to be cloned as the first parameter and the second parameter is the integer for a new file descriptor. As a result, both of these file descriptors point to the same file and can be used interchangeably. Note that if the user specifies an integer currently used by the open file as the second parameter, it will be closed and then reused as the cloned file descriptor.
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
extern int counter;
int main(void) {
int fd = open("tmp.txt", O_WRONLY | O_APPEND);
printf("tmp.txt fd = %d\n", fd);
dup2(fd, 121);
dprintf(121, "This string will be printed in tmp.txt file\n");
exit(EXIT_SUCCESS);
}
Output:
tmp.txt fd = 3
The above example demonstrates the basic usage of the dup2
function, where an arbitrary file named tmp.txt
is opened in append
mode, and some formatted text is written to it. The default file descriptor is 3
returned from the open
system call. After we execute the dup2
function call with the second argument of 121
, the same file can be addressed using the new file descriptor. Consequently, we call the dprintf
function, which’s similar to the printf
function except that it takes an additional file descriptor argument specifying the destination for writing the output.
Use the dup
Function to Duplicate a File Descriptor in C
Alternatively, another function called dup
does file descriptor cloning similar to the dup2
. Although, the dup
function takes a single argument of file descriptor to be copied and returns the newly created one automatically. The following example demonstrates dup
usage, where we store the returned value in an int
type and then pass the dprintf
function to the retrieved file descriptor. Note that the user is responsible for implementing the error checking routines for both functions to verify the successful execution. See the dup
/dup2
manual page for the specific details here.
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
extern int counter;
int main(void) {
int fd = open("tmp2.txt", O_WRONLY | O_APPEND);
printf("tmp2.txt fd = %d\n", fd);
int dup_fd = dup(fd);
dprintf(dup_fd, "This string will be printed in tmp2.txt file\n");
exit(EXIT_SUCCESS);
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook