How to Compile a C File on Mac
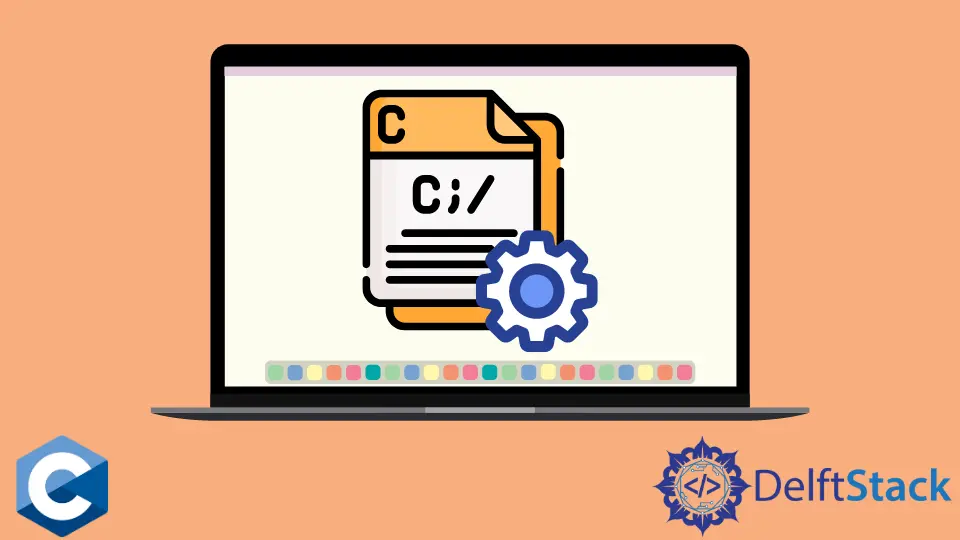
This article shows how to compile a .c
file on macOS and guides the requirements for successfully executing the .c
file.
Compile a .c
File on Mac Operating System
Compiling a .c
file on the macOS is very simple and can be done as follows.
gcc -o program program.c
Further, we run the as given below to execute the executable file.
./program
But having the right tools to execute the .c
file is most important. There are two ways to get the right tools for compiling the .c
code on macOS.
Method 1:
-
Write and save your code as a
program.c
. -
Now, we need a compiler to compile the
.c
code. Navigate to theApp Store
and install theXCode
and the development tools. -
Install the command-line tools in mac Terminal.
-
Use
xcode-select --install
to install command-line tools. -
Once we are done with this, the easiest way is to get the advantage of the
Xcode
IDE or can usegcc
as given above. We can also use theclang
(clang
LLVM compiler) instead of thegcc
if we use the latest version of macOS X.clang program.c -o program
-
Lastly, execute the program as follows.
./program
Method 2:
-
Install the
gcc
compiler. -
Make sure you are in the file’s directory first.
cd directory/path/to/file
-
Compile the code as mentioned below.
gcc program.c -o program
-
Execute the program from the terminal as follows.
./program
You can dig more about C compilers for macOS here.