How to Implement Caesar's Cipher in C
- Implement Caesar Cipher to Process Constant String in C
- Implement Caesar Cipher to Process User-Provided String in C
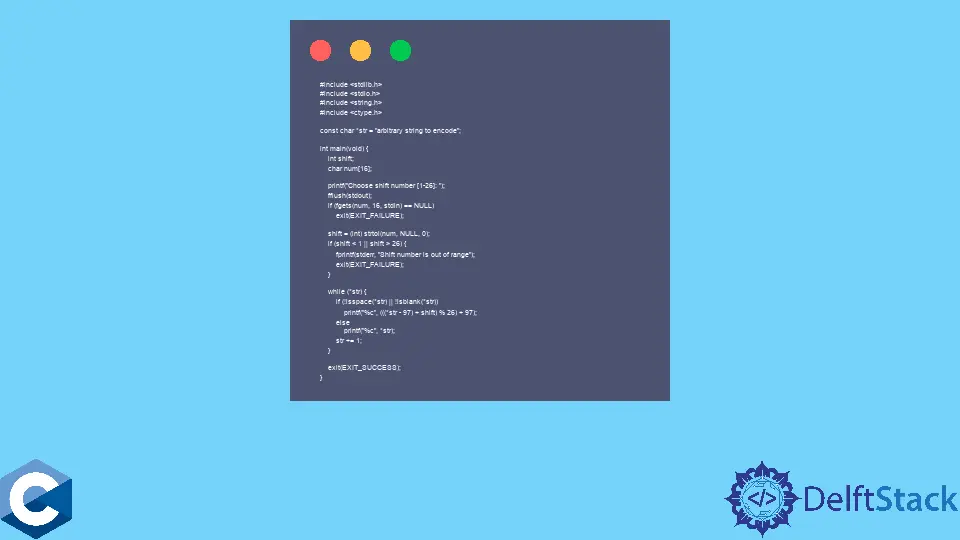
This article will demonstrate multiple methods about how to implement Caesar’s cipher in C.
Implement Caesar Cipher to Process Constant String in C
Caesar cipher is one of the simplest encryption schemes that must not be used for any reasonable secrecy but rather just intellectual curiosity. Caesar is essentially an alphabet rotation technique with the given number of positions. Given the text and position 5, the encrypted version will contain the characters that are right-shifted by 5 places. Note that the rotation direction is not strictly specified as every case has its opposite rotation that gives the same result.
In the following example, we demonstrate how to encrypt the hard-coded string
literal. The rotation position is taken from the user input and checked whether the number is in an interval of [1,26], as this example is specialized for the English alphabet only. Next, we execute the while
loop where a character is checked for non-alphabetic values and only then is shifted with the given places. This code can only process lowercase characters as we employed the 97
value representing the a
in ASCII encoding. Also, we directly output the encoded values char by char to the console.
#include <ctype.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
const char *str = "arbitrary string to encode";
int main(void) {
int shift;
char num[16];
printf("Choose shift number [1-26]: ");
fflush(stdout);
if (fgets(num, 16, stdin) == NULL) exit(EXIT_FAILURE);
shift = (int)strtol(num, NULL, 0);
if (shift < 1 || shift > 26) {
fprintf(stderr, "Shift number is out of range");
exit(EXIT_FAILURE);
}
while (*str) {
if (!isspace(*str) || !isblank(*str))
printf("%c", (((*str - 97) + shift) % 26) + 97);
else
printf("%c", *str);
str += 1;
}
exit(EXIT_SUCCESS);
}
Implement Caesar Cipher to Process User-Provided String in C
Alternatively, we can reimplement the previous code sample to take both text and rotation position from the user input, verify them, and encrypt the given string. In contrast with the previous example, this version includes two fgets
calls and malloc
to allocate dynamic memory for the cleartext. This implementation also deals with lowercase strings and would not be able to encrypt a mixed string correctly.
#include <ctype.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
enum { MAX_LEN = 1024 };
int main(void) {
size_t len;
int shift;
char *text;
char num[16];
text = malloc(MAX_LEN);
if (text == NULL) {
perror("malloc");
exit(EXIT_FAILURE);
}
printf("Input text to be encrypted (lowercase): ");
fflush(stdout);
if (fgets(text, MAX_LEN, stdin) == NULL) exit(EXIT_FAILURE);
len = strlen(text);
if (text[len - 1] == '\n') text[len - 1] = '\0';
len -= 1;
printf("Choose shift number [1-26]: ");
fflush(stdout);
if (fgets(num, 16, stdin) == NULL) exit(EXIT_FAILURE);
shift = (int)strtol(num, NULL, 0);
if (shift < 1 || shift > 26) {
fprintf(stderr, "Shift number is out of range");
exit(EXIT_FAILURE);
}
for (int i = 0; i < len; ++i) {
if (!isspace(text[i]) || !isblank(text[i]))
printf("%c", (((text[i] - 97) + shift) % 26) + 97);
else
printf("%c", text[i]);
}
exit(EXIT_SUCCESS);
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook