Null Terminated Strings in C
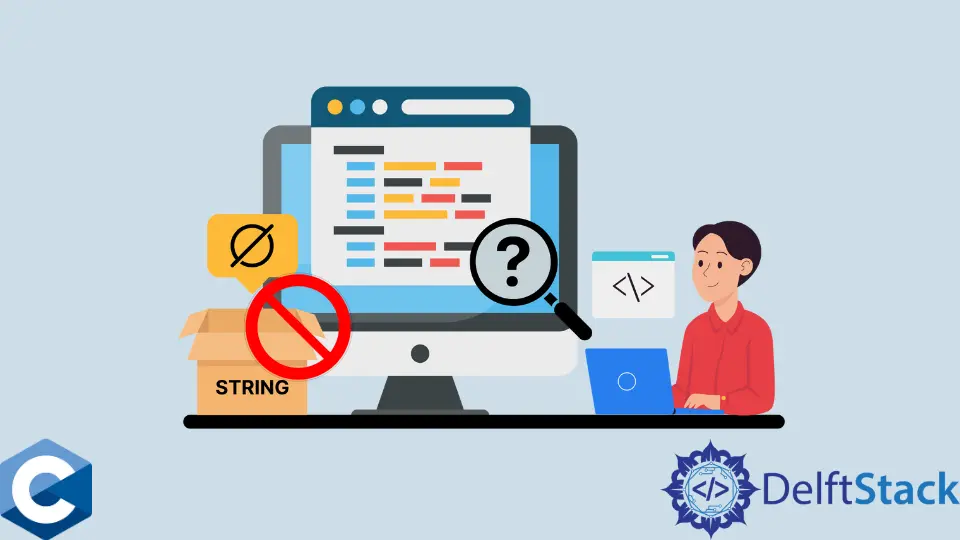
This small article will discuss the use of strings in C language. Strings in C language are formed using the character arrays.
Strings in C
In reality, strings are a one-dimensional array of characters that are closed with the null character \0
. The characters that make up the string are then followed by a null to form a string with a null termination.
The following code snippet creates a string with the null character at the end. For placing the null character at the end, we need to keep the length of the string one character greater than the original word count of the string.
char test[5] = {'T', 'e', 's', 't', '\0'};
This is one way to initialize the string in C. Note that the string size is set to 5, but the number of characters in the strings is 4, and the 5th character is the null character \0
.
You can also initialize a string as in the following code segment.
char test[] = "Test";
Hence, placing the null character at the end of the string is not mandatory. The C compiler does that for us automatically.
The terminating null character will not be printed when we print both strings. Let us look at the example below.
#include <stdio.h>
int main() {
char test[5] = {'T', 'e', 's', 't', '\0'};
char test2[] = "Test";
printf("Test message: %s\n", test);
printf("Test message 2: %s\n", test2);
}
We have created two strings in the above code segment and initialized them with the same data. We explicitly placed a null character at the end with the first one.
At the same time, it is not placed in the second one. When we print these strings, both will have the same data.
Output:
C-Strings Library Functions in C
Many functions are supported in C for null-terminated strings. Numerous functions are provided in the string
class; some are as follows.
No | Functions | Description |
---|---|---|
1 | strcpy(str1, str2); |
It copies str1 into str2. |
2 | strcat(str1, str2); |
It joins str2 and str1 such that str1 is followed by str2 |
3 | strlen(str1); |
It tells the length of str1. |
4 | strcmp(str1, str2); |
It has three results: 1 if str1 and str2 are equal; negative if str1 < str2; positive if str1> str2 |
5 | strchr(str1, c); |
Returns a reference to the the first occurrence of c in str1 |
6 | strstr(str1, str2); |
Returns a reference to the first occurrence of str2 in str1 |
The following example uses some of these functions to demonstrate the use of strings in C.
#include <stdio.h>
#include <string.h>
int main() {
char mystr1[12] = "First";
char mystr2[12] = "Second";
char mystr3[12];
int length;
/* copy the first string into the third */
strcpy(mystr3, mystr1);
printf("strcpy( mystr3, mystr1) : %s\n", mystr3);
/* concatenates first and second */
strcat(mystr1, mystr2);
printf("strcat( mystr1, mystr2): %s\n", mystr1);
/* length of the first string after concatenation */
length = strlen(mystr1);
printf("strlen(mystr1) : %d\n", length);
return 0;
}
Output: