How to Get the Size of Array in C
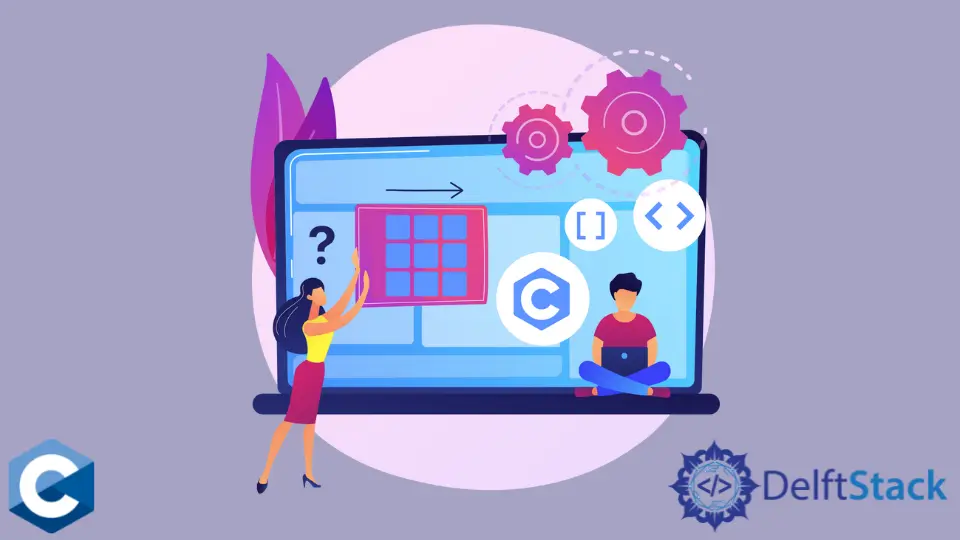
This tutorial introduces how to determine the length of an array in C. The sizeof()
operator is used to get the size/length of an array.
sizeof()
Operator to Determine the Size of an Array in C
The sizeof()
operator is a compile-time unary operator. It is used to calculate the size of its operand. It returns the size of a variable. The sizeof()
operator gives the size in the unit of byte.
The sizeof()
operator is used for any data type such as primitives like int
, float
, char
, and also non-primitives data type as an array
, struct
. It returns the memory allocated to that data type.
The output may be different on different machines like a 32-bit system can show different output and a 64-bit system can show different of the same data types.
Syntax of sizeof()
:
sizeof(operand)
- The
operand
is a data-type or any operand.
sizeof()
Operator for Primitive Data Types in C
This program uses an int
, float
as a primitive data type.
#include <stdio.h>
int main(void) {
printf("Size of char data type: %u\n", sizeof(char));
printf("Size of int data type: %u\n", sizeof(int));
printf("Size of float data type: %u\n", sizeof(float));
printf("Size of double data type: %u\n", sizeof(double));
return 0;
}
Output:
Size of char data type: 1
Size of int data type: 4
Size of float data type: 4
Size of double data type: 8
Get Length of Array in C
If we divide the array’s total size by the size of the array element, we get the number of elements in the array. The program is as below:
#include <stdio.h>
int main(void) {
int number[16];
size_t n = sizeof(number) / sizeof(number[0]);
printf("Total elements the array can hold is: %d\n", n);
return 0;
}
Output:
Total elements the array can hold is: 16
When an array is passed as a parameter to the function, it treats as a pointer. The sizeof()
operator returns the pointer size instead of array size. So inside functions, this method won’t work. Instead, pass an additional parameter size_t size
to indicate the number of elements in the array.
#include <stdio.h>
#include <stdlib.h>
void printSizeOfIntArray(int intArray[]);
void printLengthIntArray(int intArray[]);
int main(int argc, char* argv[]) {
int integerArray[] = {0, 1, 2, 3, 4, 5, 6};
printf("sizeof of the array is: %d\n", (int)sizeof(integerArray));
printSizeOfIntArray(integerArray);
printf("Length of the array is: %d\n",
(int)(sizeof(integerArray) / sizeof(integerArray[0])));
printLengthIntArray(integerArray);
}
void printSizeOfIntArray(int intArray[]) {
printf("sizeof of the parameter is: %d\n", (int)sizeof(intArray));
}
void printLengthIntArray(int intArray[]) {
printf("Length of the parameter is: %d\n",
(int)(sizeof(intArray) / sizeof(intArray[0])));
}
Output (in a 64-bit Linux OS):
sizeof of the array is : 28 sizeof of the parameter is : 8 Length of the array
is : 7 Length of the parameter is : 2
Output (in a 32-bit Windows OS):
sizeof of the array is : 28 sizeof of the parameter is : 4 Length of the array
is : 7 Length of the parameter is : 1