How to Initialize an Array to 0 in C
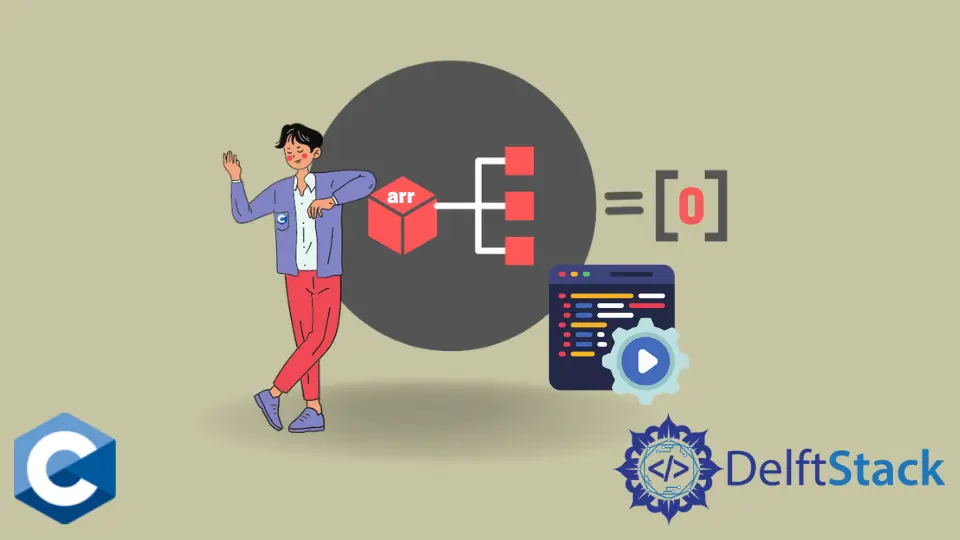
This tutorial introduces how to initialize an array to 0
in C.
The declaration of an array in C is as given below.
char ZEROARRAY[1024];
It becomes all zeros at runtime at the global scope. There is a shorthand method if it is a local array. The declaration and initialization are as below.
char ZEROARRAY[1024] = {0};
If an array is partially initialized, elements that are not initialized will receive the value 0
of the relevant data type. The compiler will fill the unwritten entries with zeros.
If there is no initializer is specified, the objects having static storage will initialize to 0. The declaration is as given below:
static int myArray[10];
An array is initialized to 0 if the initializer list is empty or 0 is specified in the initializer list. The declaration is as given below:
int number[5] = {};
int number[5] = {0};
The most simple technique to initialize an array is to loop through all the elements and set them as 0
.
#include <stdio.h>
int main(void) {
int numberArray[10], counter;
for (counter = 0; counter < 5; counter++) {
numberArray[counter] = 0;
}
printf("Array elements are:\n");
for (counter = 0; counter < 5; counter++) {
printf("%d", numberArray[counter]);
}
return 0;
}
Output:
Array elements are:
00000
Use C Library Function memset()
The function memset()
is a library function from string.h
. It is used to fill a block of memory with a particular value.
The syntax of memset()
function is below.
void *memset(void *pointerVariable, int anyValue, size_t numberOfBytes);
Where,
pointerVariable
is a pointer variable to the block of memory to fill.anyValue
is the value to be set. This is an integer value, but the function fills the block of memory using this value’s unsigned char conversion.numberOfBytes
is the number of bytes to be set to the value.
This function returns a pointer to the memory area pointerVariable
.
The complete program is as below:
#include <stdio.h>
#include <string.h>
void printArrayvalues(int anyArray[], int anyNumber) {
int index;
for (index = 0; index < anyNumber; index++) printf("%d ", anyArray[index]);
}
int main(void) {
int number = 10;
int arrayValues[number];
memset(arrayValues, 0, number * sizeof(arrayValues[0]));
printf("Array after memset()\n");
printArrayvalues(arrayValues, number);
return 0;
}
Output:
Array after memset()
0 0 0 0 0 0 0 0 0 0
Initialize the Array to Values Other Than 0
Initialization of an array to values other than 0
with gcc
is as below:
int myArrayValues[1024] = {[0 ... 1023] = -1};
Every member of an array can be explicitly initialized by omitting the dimension. The declaration is as below:
int myArrayValues[] = {1, 2, 3, 4, 5, 6, 7, 8, 9};
The compiler will deduce the dimension from the initializer list and for multidimensional arrays, only the outermost dimension may be omitted.
int myPoints[][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};