How to Get Substring in C
- Understanding Strings in C
- Method 1: Using a Custom Function
-
Method 2: Using the Standard Library Function
strncpy
- Method 3: Using Pointer Arithmetic
- Conclusion
- FAQ
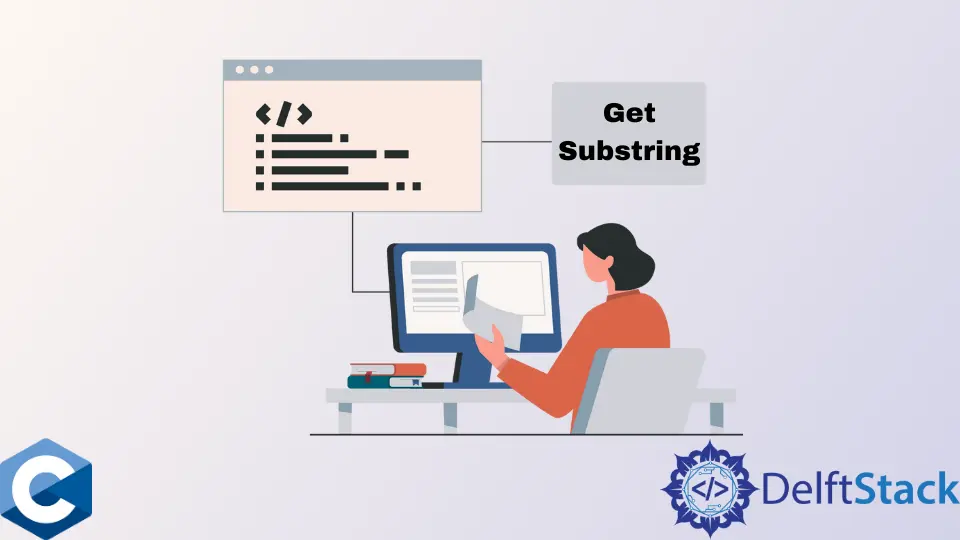
In the world of programming, extracting a substring from a string is a common task. Whether you’re processing user input or manipulating data, knowing how to get a substring in C can be incredibly useful.
This article delves into various methods to achieve this, illustrating each approach with clear examples. By the end of this guide, you’ll not only understand how to extract substrings in C but also gain insight into string manipulation techniques that can enhance your coding skills. So, let’s get started and unlock the power of substrings in C!
Understanding Strings in C
Before diving into substring extraction, it’s essential to grasp how strings work in C. Unlike higher-level languages, C does not have a dedicated string data type. Instead, strings are represented as arrays of characters, terminated by a null character (\0
). This means that any string manipulation involves careful handling of character arrays.
To extract a substring, you typically need to specify the starting position and the length of the substring you want. This can be done using a simple loop or by leveraging existing C library functions.
Method 1: Using a Custom Function
One of the simplest ways to extract a substring in C is by writing a custom function. This function will take the original string, the starting index, and the length of the desired substring as parameters. Here’s how you can implement it:
#include <stdio.h>
#include <string.h>
void getSubstring(char *source, char *dest, int start, int length) {
int i;
for (i = 0; i < length && source[start + i] != '\0'; i++) {
dest[i] = source[start + i];
}
dest[i] = '\0';
}
int main() {
char str[] = "Hello, World!";
char sub[20];
getSubstring(str, sub, 7, 5);
printf("Substring: %s\n", sub);
return 0;
}
Output:
Substring: World
In this example, the getSubstring
function takes three parameters: the source string, a destination string to store the substring, and the starting index along with the desired length. The loop iterates through the source string, copying each character to the destination until the specified length is reached or the end of the string is encountered. Finally, it appends a null character to terminate the substring properly.
Method 2: Using the Standard Library Function strncpy
If you’re looking for a more straightforward approach, C’s standard library provides a function called strncpy
. This function allows you to copy a specified number of characters from one string to another. Here’s how you can use it to extract a substring:
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, World!";
char sub[20];
strncpy(sub, str + 7, 5);
sub[5] = '\0'; // Null-terminate the substring
printf("Substring: %s\n", sub);
return 0;
}
Output:
Substring: World
In this code snippet, we utilize strncpy
to copy five characters starting from the seventh index of the original string. After copying, we explicitly add a null character to ensure the substring is properly terminated. This method is efficient and leverages built-in functionality, making it less error-prone.
Method 3: Using Pointer Arithmetic
Another elegant way to extract a substring in C is through pointer arithmetic. This method can be particularly efficient, as it allows you to manipulate the string directly without using additional arrays. Here’s an example:
#include <stdio.h>
void getSubstring(char *source, char *dest, int start, int length) {
source += start; // Move the pointer to the starting position
for (int i = 0; i < length && *source != '\0'; i++, source++) {
dest[i] = *source;
}
dest[length] = '\0'; // Null-terminate the substring
}
int main() {
char str[] = "Hello, World!";
char sub[20];
getSubstring(str, sub, 7, 5);
printf("Substring: %s\n", sub);
return 0;
}
Output:
Substring: World
In this method, we adjust the source pointer to point directly to the starting index. The loop then copies characters from the original string to the destination until the specified length is reached or the end of the string is encountered. This approach is efficient and can be more intuitive once you become familiar with pointer operations.
Conclusion
Extracting substrings in C is a fundamental skill that enhances your ability to manipulate strings effectively. Whether you choose to write a custom function, use strncpy
, or leverage pointer arithmetic, each method offers unique advantages. By mastering these techniques, you can tackle a variety of programming challenges with confidence. Remember, practice is key; the more you work with strings, the more proficient you’ll become. Happy coding!
FAQ
-
What is a substring in C?
A substring is a contiguous sequence of characters within a string. In C, you can extract it using various methods. -
Can I extract a substring without using additional memory?
Yes, you can manipulate pointers to work directly with the original string, but you still need a destination to store the substring. -
Is there a built-in function in C for substring extraction?
There is no dedicated built-in function for substrings, but functions likestrncpy
can be used to achieve similar results. -
What happens if I try to extract a substring that exceeds the string length?
If you exceed the string length, you may encounter undefined behavior or access invalid memory. Always ensure your indices are within bounds. -
Can I extract substrings from user input in C?
Yes, you can use functions likescanf
to get user input and then apply substring extraction techniques on that input.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook