%G Format Specifier in C
- What is the %g Format Specifier?
- Using %g in C
-
Precision Control with
%g
- Differences Between %g and Other Specifiers
- Conclusion
- FAQ
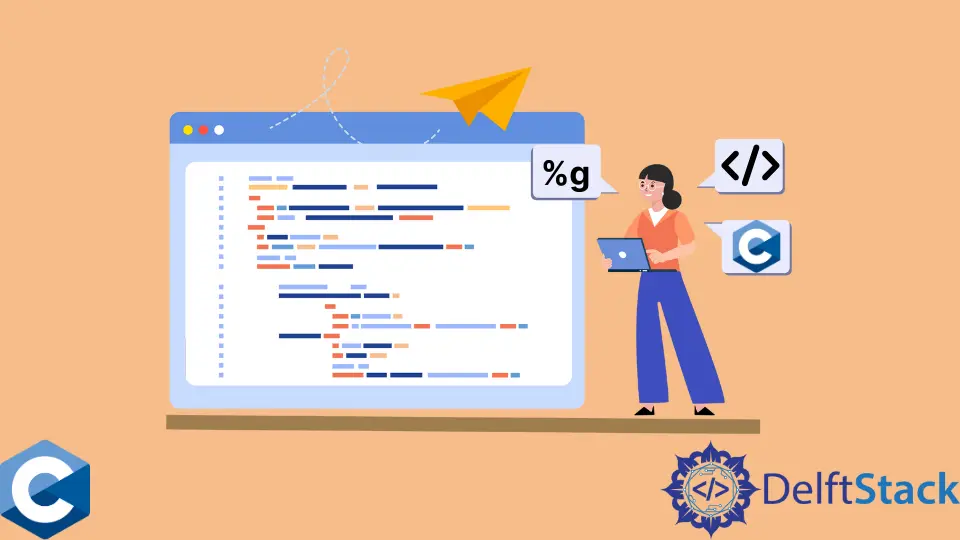
When diving into the world of C programming, one of the essential elements you will encounter is format specifiers. Among these, the %g
format specifier plays a crucial role in how floating-point numbers are represented and displayed.
This article will explore the %g
specifier in detail, shedding light on its function, usage, and the nuances that set it apart from other format specifiers like %f and %e. Whether you’re a beginner looking to understand the basics or an experienced programmer wanting to refine your skills, this guide will provide valuable insights into using the %g format specifier effectively in your C programs.
What is the %g Format Specifier?
Following are some of the format specifiers used in the C programming language.
Format Specifier | Description |
---|---|
%g |
Similar as %e or %E |
%f |
Float Format Specifier |
%c |
Character Format Specifier |
%s |
String Format Specifier |
%e |
Scientific notation of floats |
%d |
Integer Format Specifier |
%x |
Hexadecimal representation |
The %g format specifier is used in C to format floating-point numbers. It automatically chooses between two formats: fixed-point notation (%f) and scientific notation (%e), depending on the value being represented. This is particularly useful for displaying numbers in a concise manner without unnecessary trailing zeros. For example, if you have a very small or very large number, %g will format it in a way that is both readable and precise.
When using %g, the output will not include any decimal places if the number is an integer or if the trailing zeros can be omitted. This adaptability makes it a preferred choice for many programmers, especially when dealing with dynamic numerical data.
Using %g in C
To effectively utilize the %g format specifier, you can start with a simple C program. Below is a basic example that demonstrates its usage.
#include <stdio.h>
int main() {
float num1 = 123456.789;
float num2 = 0.000012345;
float num3 = 0.00000000012345;
printf("Using %%g format specifier:\n");
printf("Number 1: %g\n", num1);
printf("Number 2: %g\n", num2);
printf("Number 3: %g\n", num3);
return 0;
}
Output:
Using %g format specifier:
Number 1: 123456
Number 2: 1.2345e-05
Number 3: 1.2345e-10
In this example, we define three floating-point numbers. The first number is large enough to be displayed in fixed-point notation, while the second and third numbers are small enough to be represented in scientific notation. Notice how %g automatically determines the best format for each number, simplifying the output process.
Precision Control with %g
One of the powerful features of the %g format specifier is its ability to control precision. You can specify the number of significant digits you want to display, which can be particularly helpful in scientific computations where precision is key. Here’s how you can implement precision control in your C code.
#include <stdio.h>
int main() {
float num1 = 123.456789;
float num2 = 0.000123456;
printf("Using %%g with precision:\n");
printf("Number 1 (3 significant digits): %.3g\n", num1);
printf("Number 2 (5 significant digits): %.5g\n", num2);
return 0;
}
Output:
Using %g with precision:
Number 1 (3 significant digits): 1.23e+02
Number 2 (5 significant digits): 0.00012346
In this code snippet, we specify precision using the format %.3g
and %.5g
. The first number is rounded to three significant digits, while the second is rounded to five. This level of control allows you to tailor the output to your needs, ensuring that you present numerical data in the most effective way possible.
Differences Between %g and Other Specifiers
Understanding the differences between %g and other format specifiers like %f and %e is vital for any C programmer. The %f specifier always displays numbers in fixed-point notation, while %e always uses scientific notation. The %g specifier, on the other hand, intelligently chooses between these formats based on the value.
Here’s a quick comparison:
%f
: Fixed-point notation, always shows decimal points.%e
: Scientific notation, always shows the exponent.%g
: Chooses between %f and %e, omitting trailing zeros and unnecessary decimal points.
Let’s see this in action with a simple program.
#include <stdio.h>
int main() {
float num = 123456.789;
printf("Using %%f: %f\n", num);
printf("Using %%e: %e\n", num);
printf("Using %%g: %g\n", num);
return 0;
}
Output:
Using %f: 123456.789000
Using %e: 1.234568e+05
Using %g: 123456
As you can see, the %f specifier displays the full number with decimal points, while %e presents it in scientific notation. The %g specifier opts for a more concise representation, making it easier to read and understand.
Conclusion
In summary, the %g format specifier in C is a versatile tool for formatting floating-point numbers. Its ability to switch between fixed-point and scientific notation, along with precision control, makes it an invaluable asset for any programmer. By understanding how to effectively use %g, you can enhance the clarity and readability of your numerical outputs, ensuring that your programs communicate information effectively. Whether you are developing scientific applications or simply need to display numbers, mastering %g will undoubtedly improve your coding skills.
FAQ
-
What does the %g format specifier do in C?
The %g format specifier is used to format floating-point numbers, automatically choosing between fixed-point and scientific notation based on the value. -
How do I control the precision of the %g specifier?
You can control the precision by specifying it in the format string, for example, %.3g for three significant digits. -
How does %g differ from %f and %e?
%f always uses fixed-point notation, %e uses scientific notation, while %g chooses the most appropriate format based on the value. -
Can %g display negative numbers?
Yes, the %g specifier can display negative floating-point numbers just like %f and %e. -
Is there a limit to the number of significant digits with %g?
Yes, the number of significant digits can be controlled, but it is generally limited by the precision of the floating-point representation in C.
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn