How to Fix Free Invalid Pointer Error in C
-
Don’t
free
Pointers That Point to Non-Dynamic Memory Locations - Don’t Free Pointers That Already Have Been Freed
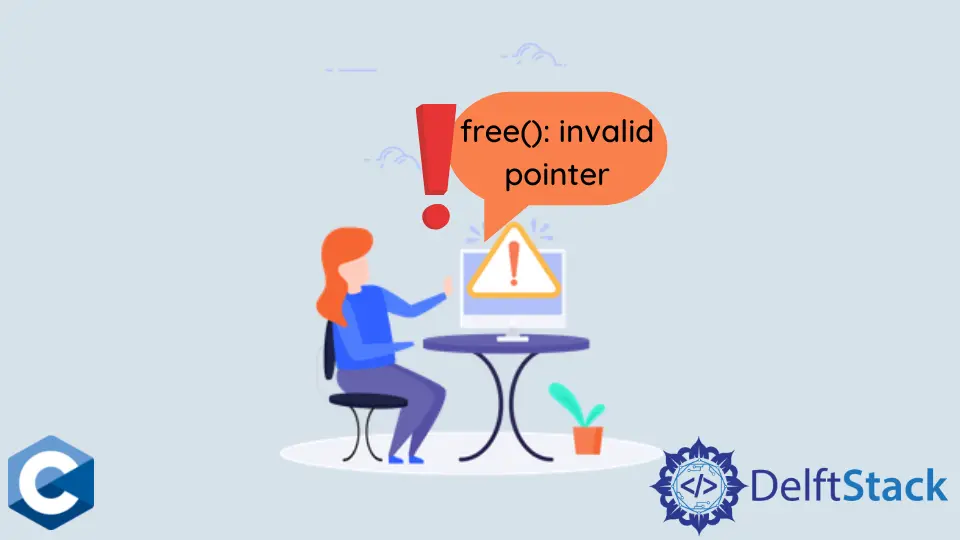
This article will introduce multiple methods about how to fix the free
invalid pointer error in C.
Don’t free
Pointers That Point to Non-Dynamic Memory Locations
free
function call should only be used to deallocate memory from the pointers that have been returned by malloc
, calloc
, or realloc
functions.
The following code shows the scenario where the char*
pointer is assigned a value returned from the malloc
call, but later in the else
block, the same pointer is reassigned with a string literal. This means that the c_str
variable points to the location that is not a dynamic memory region; thus, it is not allowed to be passed to the free
function.
As a result, when the next example is executed, and the program reaches the free
function call, it is aborted, and free(): invalid pointer
error is displayed.
Note that one should not reassign the pointers to dynamic memory locations with different addresses unless there are other pointer variables still pointing to the original location. Finally, you should only call the free
function on pointers that point to heap memory.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, const char *argv[]) {
char *c_str = NULL;
size_t len;
if (argc != 2) {
printf("Usage: ./program string\n");
exit(EXIT_FAILURE);
}
if ((len = strlen(argv[1])) >= 4) {
c_str = (char *)malloc(len);
if (!c_str) {
perror("malloc");
}
strcpy(c_str, argv[1]);
printf("%s\n", c_str);
} else {
c_str = "Some Literal String";
printf("%s\n", c_str);
}
free(c_str);
exit(EXIT_SUCCESS);
}
Don’t Free Pointers That Already Have Been Freed
Another common error when using the dynamic memory is to call the free
function on the pointers that have already been freed. This scenario is most likely when there are multiple pointer variables pointing to the same dynamic memory region.
The following sample code demonstrates one such possible case, where the same location is freed in different scopes.
Note that this example is a single file short program, and it will be easy to diagnose an issue like this, but in the larger codebases, one might find it difficult to track the source without external inspector programs that do static analysis.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, const char *argv[]) {
char *c_str = NULL;
size_t len;
if (argc != 2) {
printf("Usage: ./program string\n");
exit(EXIT_FAILURE);
}
char *s = NULL;
if ((len = strlen(argv[1])) >= 4) {
c_str = (char *)malloc(len);
s = c_str;
if (!c_str) {
perror("malloc");
}
strcpy(c_str, argv[1]);
printf("%s\n", c_str);
free(c_str);
} else {
c_str = "Some Literal String";
printf("%s\n", c_str);
}
free(s);
exit(EXIT_SUCCESS);
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook