How to Compare Strings in C
-
Use the
strcmp
Function to Compare Strings -
Use the
strncmp
Function to Compare Only Certain Parts of Strings -
Use
strcasecmp
andstrncasecmp
Functions to Compare Strings Ignoring the Case of Letters
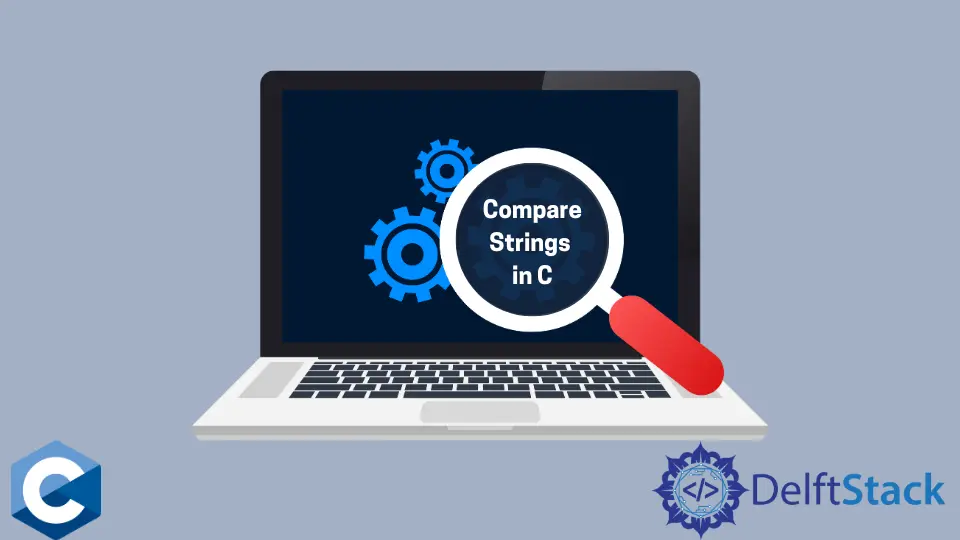
This article will introduce multiple methods about how to compare strings in C.
Use the strcmp
Function to Compare Strings
The strcmp
function is the standard library feature defined in the <string.h>
header. C-style strings are just character sequences terminated by the \0
symbol, so the function would have to compare each character with iteration.
strcmp
takes two character strings and returns the integer to denote the result of the comparison. The returned number is negative if the first string is lexicographically less than the second string, or positive if the latter one is less than the former, or 0
if the two strings are identical.
Note that, in the following example, we invert the return value of the function and insert it into the ?:
conditional statement to print the corresponding output to the console.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
const char* str1 = "hello there 1";
const char* str2 = "hello there 2";
const char* str3 = "Hello there 2";
!strcmp(str1, str2) ? printf("strings are equal\n")
: printf("strings are not equal\n");
!strcmp(str1, str3) ? printf("strings are equal\n")
: printf("strings are not equal\n");
exit(EXIT_SUCCESS);
}
Output:
strings are not equal
strings are not equal
Use the strncmp
Function to Compare Only Certain Parts of Strings
strncmp
is another useful function defined in the <string.h>
header, and it can be utilized to compare only several characters from the beginning of the strings.
strncmp
takes the third argument of integer type to specify the number of characters to compare in both strings. The return values of the function are similar to the ones returned by the strcmp
.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
const char* str1 = "hello there 1";
const char* str2 = "hello there 2";
!strncmp(str1, str2, 5) ? printf("strings are equal\n")
: printf("strings are not equal\n");
exit(EXIT_SUCCESS);
}
Output:
strings are equal
Use strcasecmp
and strncasecmp
Functions to Compare Strings Ignoring the Case of Letters
strcasecmp
function behaves similarly to the strcmp
function except that it ignores the letter case. This function is POSIX compliant and can be utilized on multiple operating systems along with strncasecmp
, which implements the case insensitive comparison on the certain number of characters in both strings. The latter parameter can be passed to the function with the third argument of type size_t
.
Notice that the return values of these functions can be used directly in conditional statements.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
const char* str1 = "hello there 2";
const char* str3 = "Hello there 2";
!strcasecmp(str1, str3) ? printf("strings are equal\n")
: printf("strings are not equal\n");
!strncasecmp(str1, str3, 5) ? printf("strings are equal\n")
: printf("strings are not equal\n");
exit(EXIT_SUCCESS);
}
Output:
strings are equal
strings are equal
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook