How to Use Bitwise Shift Operations in C
-
Use the
<<
Operator to Shift the Number to the Left in C - Use Left Shift to Multiple Integer by Two in C
- Right Shift - Arithmetic vs Logical Shift Difference in C
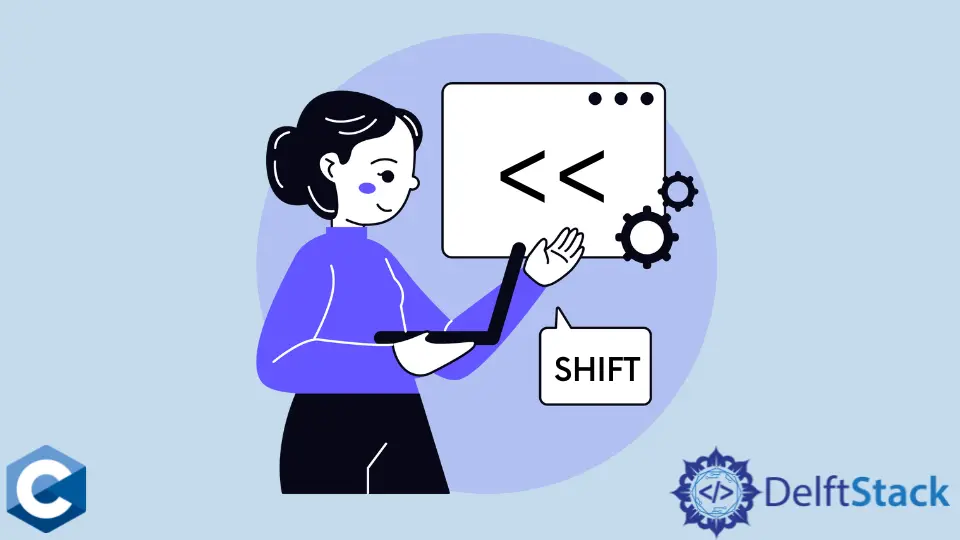
This article will explain several methods of how to use bitwise shift operations in C.
Use the <<
Operator to Shift the Number to the Left in C
Bitwise shift operations are part of every programming language, and they reposition each bit of an integer operand by the specified number of places. To better demonstrate these operations’ effects, we included the function named binary
in the following examples that prints the binary representation for the given integer. Note that this function is only implemented to work with 32-bit integer values. The following example code demonstrates the left shift by two positions and displays the number representations accordingly.
#include <stdio.h>
#include <stdlib.h>
void binary(unsigned n) {
unsigned i;
for (i = 1 << 31; i > 0; i /= 2) (n & i) ? printf("1") : printf("0");
}
int main(int argc, char *argv[]) {
int n1 = 123;
binary(n1);
printf(" : %d\n", n1);
n1 <<= 2;
binary(n1);
printf(" : %d\n", n1);
exit(EXIT_SUCCESS);
}
Output:
00000000000000000000000001111011 : 123
00000000000000000000000111101100 : 492
Use Left Shift to Multiple Integer by Two in C
We can utilize left shift operation to implement the multiplication by two, which can be more efficient on the hardware. Note that when shifting left, there’s no difference between the arithmetic and logical shift. The single position shift for the given integer results in multiplication; thus we can shift further to get the multiplication accordingly.
#include <stdio.h>
#include <stdlib.h>
void binary(unsigned n) {
unsigned i;
for (i = 1 << 31; i > 0; i /= 2) (n & i) ? printf("1") : printf("0");
}
int main(int argc, char *argv[]) {
int n1 = 123;
printf("%d\n", n1);
n1 <<= 1;
printf("%d x2\n", n1);
exit(EXIT_SUCCESS);
}
Output:
492
984 x2
Right Shift - Arithmetic vs Logical Shift Difference in C
It should be mentioned that signed
and unsigned
integers are represented differently underneath the hood. Namely, signed
ones are implemented as two’s complement values. As a result, the most significant bit of negative numbers is 1
called sign bit, whereas positive integers start with 0
as usual. Thus, when we shift the negative numbers right logically, we lose their sign and get the positive integer. So, we need to differentiate logical and arithmetic shifts, the latter of which preserves the most significant bit. Even though there’s difference between concepts, C does not provide separate operators. Additionally, the C standard does not specify the behavior, as it is defined by hardware implementation. As shown in the following example output, the underlying machine conducted the arithmetic shift and preserved the integer’s negative value.
#include <stdio.h>
#include <stdlib.h>
void binary(unsigned n) {
unsigned i;
for (i = 1 << 31; i > 0; i /= 2) (n & i) ? printf("1") : printf("0");
}
int main(int argc, char *argv[]) {
int n2 = -24;
binary(n2);
printf(" : %d\n", n2);
n2 >>= 3;
binary(n2);
printf(" : %d\n", n2);
exit(EXIT_SUCCESS);
}
Output:
11111111111111111111111111101000 : -24
11111111111111111111111111111101 : -3
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook