argc and argv in C
-
Use the
int argc, char *argv[]
Notation to Get Command-Line Arguments in C -
Use
memccpy
to Concatenate Command-Line Arguments in C
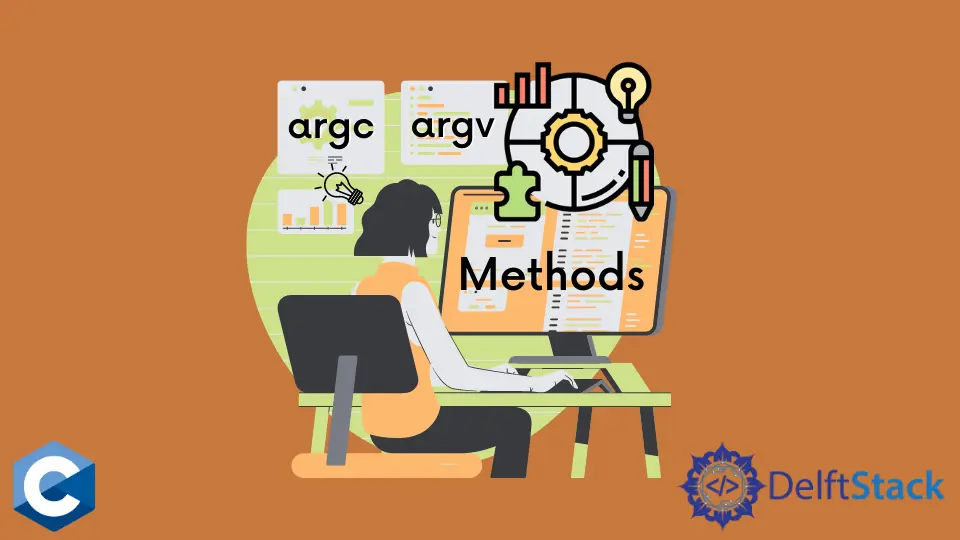
This article will explain several methods of using command-line arguments, argc
and argv
, in C.
Use the int argc, char *argv[]
Notation to Get Command-Line Arguments in C
When a program gets executed, the user can specify the space-separated strings called command-line arguments. These arguments are made available in the program’s main
function and can be parsed as individual null-terminated strings. To access the arguments, we should include parameters as int argc, char *argv[]
, representing the number of arguments passed and the array of strings containing command-line arguments. The first string in the array is the program name itself as per the convention; thus, the number of arguments argc
includes the program name. We can print every command-line argument with simple iteration through argv
array, as demonstrated in the following example.
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[]) {
for (int i = 0; i < argc; i++) printf("argv[%d] = %s\n", i, argv[i]);
exit(EXIT_SUCCESS);
}
Sample Command:
./program hello there
Output:
argv[0] = ./program
argv[1] = hello
argv[2] = there
argv
array of null-terminated strings is terminated with a NULL
pointer to denote the last argument. Thus, we can utilize this feature to implement the argument printing loop by evaluating the argv
pointer itself and incrementing it until equals NULL
. Note that it’s better to make a separate char*
pointer for the loop to preserve the array’s original address in case it’s needed later in the program. The following sample code assumes the same command is executed as in the previous example.
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[]) {
char **ptr;
for (ptr = argv; *ptr != NULL; ptr++) printf("%s\n", *ptr);
exit(EXIT_SUCCESS);
}
Output:
./program
hello
there
Use memccpy
to Concatenate Command-Line Arguments in C
The memccpy
function is part of the standard library string utilities used to concatenate the argv
array strings. Note that memccpy
is similar to memcpy
except that it takes the fourth argument to specify the character when to stop copying. We exploit the latter feature to copy the string contents only and stop at terminating null byte. In the following example, we check if the user-provided precisely two arguments (except the program name) and only then continue the program’s execution. Consequently, we chain two memccpy
calls to copy both arguments and print the concatenated string to the stdout
.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: ./program string1 string2\n");
exit(EXIT_FAILURE);
}
size_t size = strlen(argv[1]) + strlen(argv[2]);
char buf[size];
memccpy(memccpy(buf, argv[1], '\0', size) - 1, argv[2], '\0', size);
printf("%s\n", buf);
exit(EXIT_SUCCESS);
}
Output:
hellothere
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook