How to Use
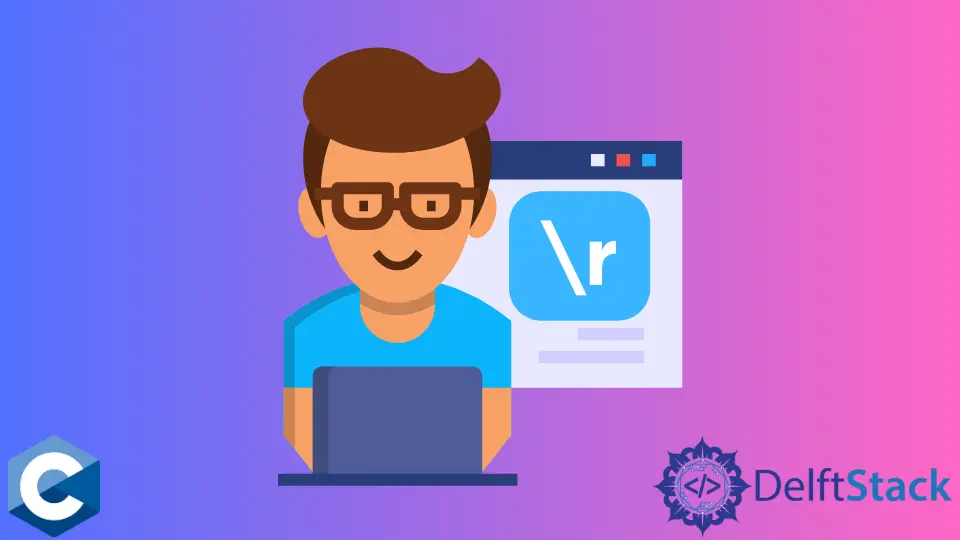
This tutorial will discuss using \r
as the carriage return character or moving the cursor back to the beginning of the line in C.
Use \n
as Carriage Return Character in C
\r
is used as a carriage return character representing the return or Enter key present on a keyboard. The carriage return key changes with respect to an operating system like Windows uses \n
and macOS uses \r
as the carriage return key.
If we want to check if the Enter key is pressed, we can use the \r
or \n
to represent the return or Enter key. For example, we can use the carriage return key if we want to take input from the user and end the input process when the user presses the Enter or return key from the keyboard.
For example, let’s write a code that asks the user for a sentence in lowercase letters, then counts the number of words and characters present in the sentence, and when the user press Enter or return key, the code will end. See the code below.
#include <stdio.h>
int main() {
int c_char = 0;
int c_word = 1;
printf("Enter Sentence: ");
char ch = 'a';
while (ch != '\n') {
ch = getchar();
if (ch == ' ')
c_word++;
else
c_char++;
}
printf("\n Words = %d ", c_word);
printf("Characters = %d", c_char - 1);
getch();
}
Output:
Enter Sentence: hello world
Words = 2 Characters = 10
In the above code, we used two variables, c_char
and c_word
, to store the number of characters and words. We initialized the character used to terminate the loop and compared it with the carriage return character, which is \n
because the code is running on Windows.
We used the getchar()
function to get the characters that are being pressed from the keyboard, and when the return key or enter key is pressed, the loop will break, and the number of words and characters will be printed using the printf()
function. We used the if
statement to check if the space key is pressed, and if it is pressed, we will increment the c_word
variable, and if some other key is pressed, we will increment the c_char
variable.
The getch()
function is used to pause the output until a key is pressed so we can see the output and input. In the above code, we used the \n
as the carriage return key because the code is running on Windows, and if the code does not terminate, we can test the code with \r
.
Use \r
to Move the Cursor to the Beginning of Line in C
The \r
character can also be used to move the cursor back to the beginning of a line in some functions like printf()
, stderr()
, and stdout()
. The cursor will move to the beginning and replace the previous text before the \r
character with the new text present after the \r
character.
For example, let’s use the \r
character inside the printf()
function to replace the text. See the code below.
#include <stdio.h>
int main() {
printf("hello hello \rworld\n");
printf("hello hello \rworld world");
}
Output:
world hello
world world
In the first line of the above code, only the first word or first four characters are replaced by the new word, and the rest of the text remains the same. In the second line of the code, the whole previous text is replaced with the new text because the number of characters in the new text equals the number of the previous text.
All the previous text characters will be replaced only if the new text characters are greater than or equal to the new text characters.