How to SendKeys in Batch Script
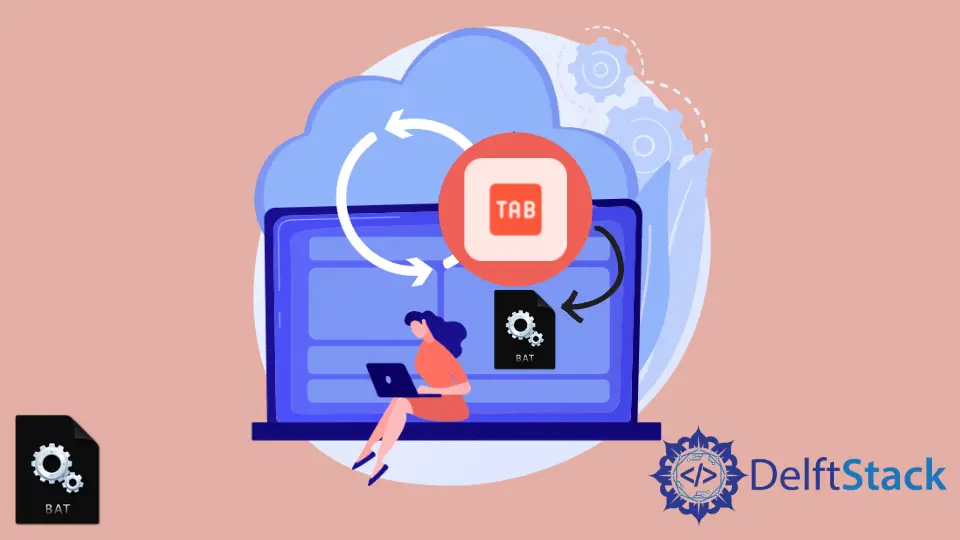
This is an interesting topic. In this article, we will generate a keypress from the Batch script.
For this, we don’t need to press the key from the keyboard. Our script will automatically generate the keypress programmatically.
We will also see an example with an explanation to make the topic easy to understand.
SendKeys
in Batch Script
The example below will open a browser window and press the Tab key to move on the input field. The code for our example will look like the below.
@if (@CodeSection == @Batch) @then
@echo off
SET SendKeys=CScript //nologo //E:JScript "%~F0"
START chrome -new-window --incognito "https://google.com/"
TIMEOUT /t 3
%SendKeys% "%USERNAME%"
%SendKeys% "{TAB}"
GOTO :EOF
@end
// JScript section
var WshShell = WScript.CreateObject("WScript.Shell");
WshShell.SendKeys(WScript.Arguments(0));0
In the above example, through the line SET SendKeys=CScript //nologo //E:JScript "%~F0"
, we send keys to the keyboard buffer by using %SendKeys%
. This script will open a browser window.
Through the line TIMEOUT /t 3
, we set a timer to wait for the web to load. We use the Tab key to move on different fields.
So we will press a Tab key to move on fields from our Batch scripts. Remember, this example is the combination of JScript.
JScript is the legacy ECMAScript dialect used in Microsoft’s Internet Explorer.
Some Important Keys
Key | Code |
---|---|
BACKSPACE | {BACKSPACE} , {BS} , or {BKSP} |
BREAK | {BREAK} |
CAPS LOCK | {CAPSLOCK} |
DEL or DELETE | {DELETE} or {DEL} |
DOWN ARROW |
{DOWN} |
END | {END} |
ENTER | {ENTER} or ~ |
ESC | {ESC} |
HELP | {HELP} |
HOME | {HOME} |
INS or INSERT | {INSERT} or {INS} |
LEFT ARROW |
{LEFT} |
NUM LOCK | {NUMLOCK} |
PAGE DOWN | {PGDN} |
PAGE UP | {PGUP} |
PRINT SCREEN | {PRTSC} |
RIGHT ARROW |
{RIGHT} |
SCROLL LOCK | {SCROLLLOCK} |
TAB | {TAB} |
UP ARROW |
{UP} |
F1 | {F1} |
F2 | {F2} |
F3 | {F3} |
F4 | {F4} |
F5 | {F5} |
F6 | {F6} |
F7 | {F7} |
F8 | {F8} |
F9 | {F9} |
F10 | {F10} |
F11 | {F11} |
F12 | {F12} |
F13 | {F13} |
F14 | {F14} |
F15 | {F15} |
F16 | {F16} |
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn