How to Send Email From Batch Script
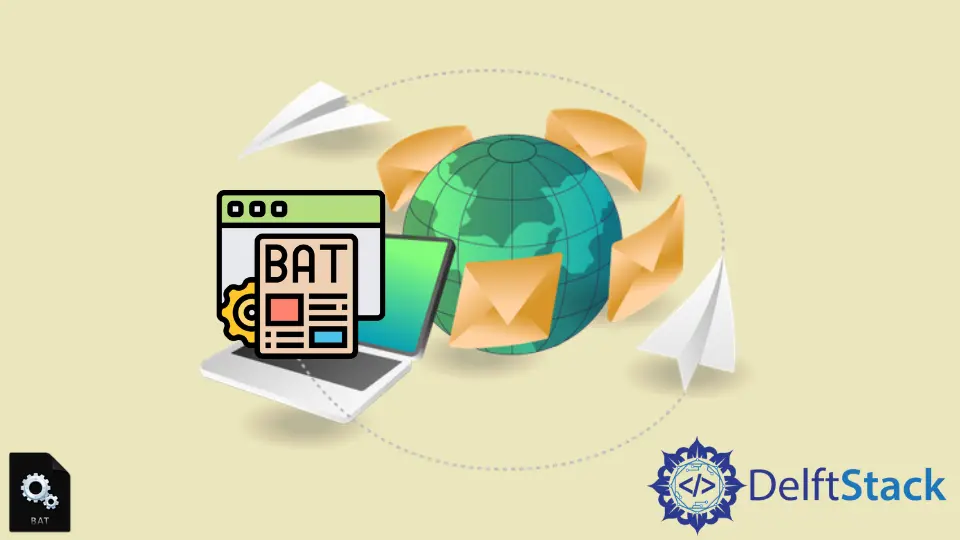
You can follow this article if you want to send an email through a Batch Script.
Use PowerShell to Send Email From Batch Script
This article will send a simple email using Command Prompt and Windows PowerShell. But our code is mainly based on Windows PowerShell, which is quite similar to Batch Script and can also run with the Batch Script.
The general format of code shared below can send mail to a specific destination.
Send-MailMessage
-To "Receiver's email address"
-From "Sender's email address"
-Subject "Subject of the message"
-Body "Your message body here"
-Credential (Get-Credential)
-SmtpServer "smtp.YourServer.com"
-Port 587
Now edit the code according to your preferences, and your sample code will look similar to the one below. Below shared an example through which we will send a mail from alen@gmail.com
to steve@gmail.com
.
Send-MailMessage
-To "steve@gmail.com"
-From "alen@gmail.com"
-Subject "Email to Steve"
-Body "Hi Steve, What's going on?"
-Credential (Get-Credential)
-SmtpServer "smtp.gmail.com"
-Port 587
This is the most basic look of an email sending script in PowerShell. Now let’s see what happens on each line of the example.
Send-MailMessage
holds the whole configuration and setting for an email like the sender’s email address, receiver’s email address, email subject, email body, server details, server port, etc.
-To "steve@gmail.com"
set the email’s destination. We included our recipient email address here.
The line -From "alen@gmail.com"
holds the sender’s email address; we included our server’s mail here. We provided the email subject on this line -Subject "Email to Steve"
.
The line -Body "Hi Steve, What's going on?"
will include the whole message body of the email. -Credential (Get-Credential)
line is for creating PSCredential
objects that provide a set of security credentials like username, password, etc.
The Get-Credential
is a PowerShell cmdlet that creates a credential object. Now the line -SmtpServer "smtp.gmail.com"
provides the SMTP server of the email.
You can customize these settings as per your requirements and preferences. The last line -Port 587
, adds the port number.
Running this program will open a popup window to take necessary security credentials like username and password. Below we discussed how we could run a PowerShell script in Command Prompt.
You can follow that method to run the PowerShell script in Command Prompt.
Run a PowerShell Script in Command Prompt Environment
It is very easy to run a PowerShell script in Command Prompt. First, you need to run the Command Prompt as an administrator.
Type PowerShell
and hit Enter. Now, the PowerShell environment is enabled in your Command Prompt.
Next, copy the code you have recently created based on the example above and hit Enter. You may need to provide necessary security options like username and password before proceeding.
Based on your SMTP server requirements, you may need to change some of them before running the program.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn