How to Run Python File in Batch Script
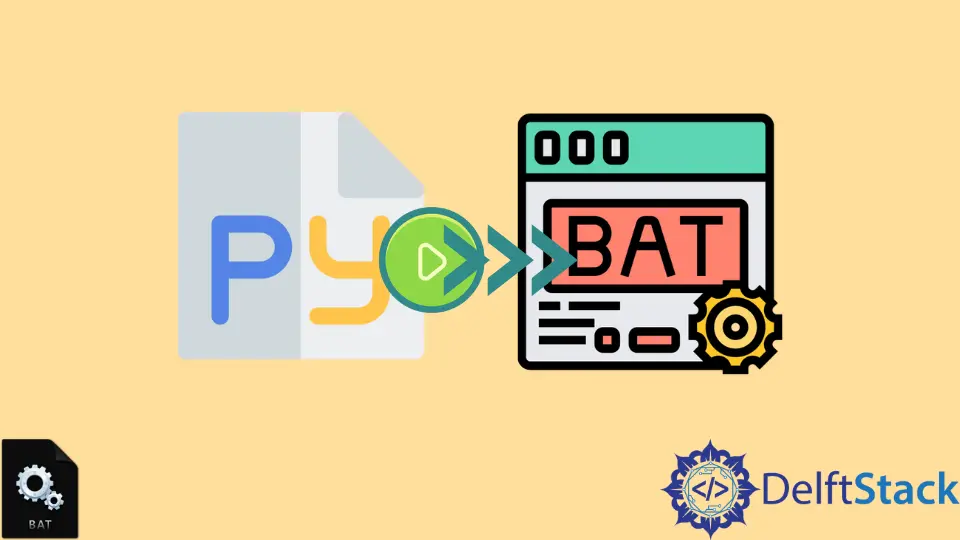
In this article, we’ll discuss some methods to run a Python file with a Batch script. Before we go forward, you need to check whether your system contains Python in your system environment.
You can check it by using the following command in Windows CMD.
Command:
python -V
If the output of this command is something like this Python 2.7.1
, then it’s okay. Otherwise, you need to download Python and set it to your system environment.
Directly Refer to the Python Script (Method 1)
After installing Python on your system, you need to locate the file named python.exe
.
Syntax:
D:\YourLocationTo\python.exe D:\Directory\Yourfile.py
Then use the following command.
Command:
c:\python27\python.exe c:\TextPython.py %*
In the above example, we run the file named TextPython.py
.
Refer to the Batch Script (Method 2)
This way, we will create a Batch script that will run a Python script.
Syntax:
python c:\Directory\YourPythonScript.py %*
Let’s have an example using this command. In the example below, we will run a Python script named Yourfile.py
through the Batch script.
Command:
@echo off
PYTHON D:\Directory\Yourfile.py %*
PAUSE
Using the above-shared methods, you can run a Python file through your Batch script. Remember, you need to check whether Python is enabled in your system as a system environment.
Without enabling Python, these methods will not work. Note that the code we shared in this article is written in Batch Script and only runs on the Windows Command Prompt environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn