How to Run Batch Script in C#
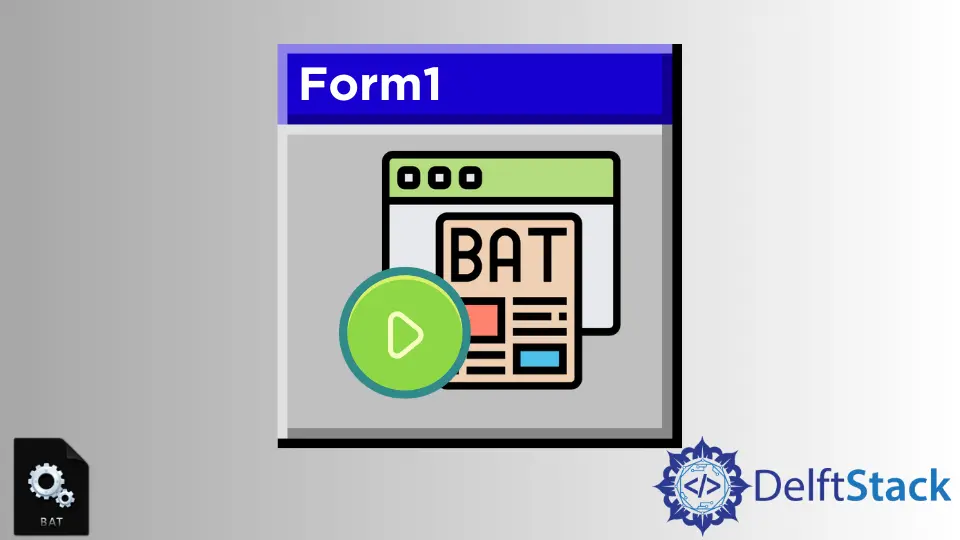
In this article, we will see how we can write a C# program that can run a Batch file from a directory.
Run Batch Script in C#
In C#, when we want to execute a Batch file, it acts as a process. You can follow the example code below to run a batch script using the C# program.
System.Diagnostics.Process pros = new System.Diagnostics.Process();
pros.StartInfo.FileName = "C:\\MyDir\\simple.bat";
pros.StartInfo.WorkingDirectory = "C:\\MyWorkDir";
pros.Start();
In the example code above, we execute a batch script named simple.bat
. Here you need to set the working directory before starting the process.
The above example is the piece of code that can run a batch file from a specified directory. In the following snippet, we have shortly performed the same task.
Code - C#:
using System;
using System.Collections.Generic;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Diagnostics;
namespace BatchLoader {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Button_Click(object sender, EventArgs e) {
// initialize empty process
Process pros = null;
try {
string BatFileDir = string.Format(@"D:\"); // directory of the file
pros = new Process();
pros.StartInfo.WorkingDirectory = BatFileDir;
pros.StartInfo.FileName = "Mybat.bat"; // batch file name to be execute
pros.StartInfo.CreateNoWindow = false;
pros.Start(); // run batch file
pros.WaitForExit();
MessageBox.Show("Batch file successfully executed !!");
} catch (Exception ex) {
Console.WriteLine(ex.StackTrace.ToString());
}
}
}
}
First, we initialize all the necessary packages to our code. Then we initialize all the graphical components.
We provide the action to run the batch file through a button. Through the line Process pros = null;
, we initialize an empty process.
We keep our main part of the code in an exception handler as it can generate runtime errors. Through the line string BatFileDir = string.Format(@"D:\");
we take a string containing the file’s directory.
After that, we declared a new process and initialized the working directory with the variable BatFileDir
. We set the filename through the line pros.StartInfo.FileName = "Mybat.bat";
and disable opening new window by the line pros.StartInfo.CreateNoWindow = false;
.
Then we execute the batch file through the line pros.Start();
. The line pros.WaitForExit();
make the program wait until it finishes the execution of the Batch file.
Lastly, we showed a message to the user that the Batch file was successfully executed through the line MessageBox.Show("Batch file successfully executed !!");
.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn