How to Retrieve Substring in Batch Script
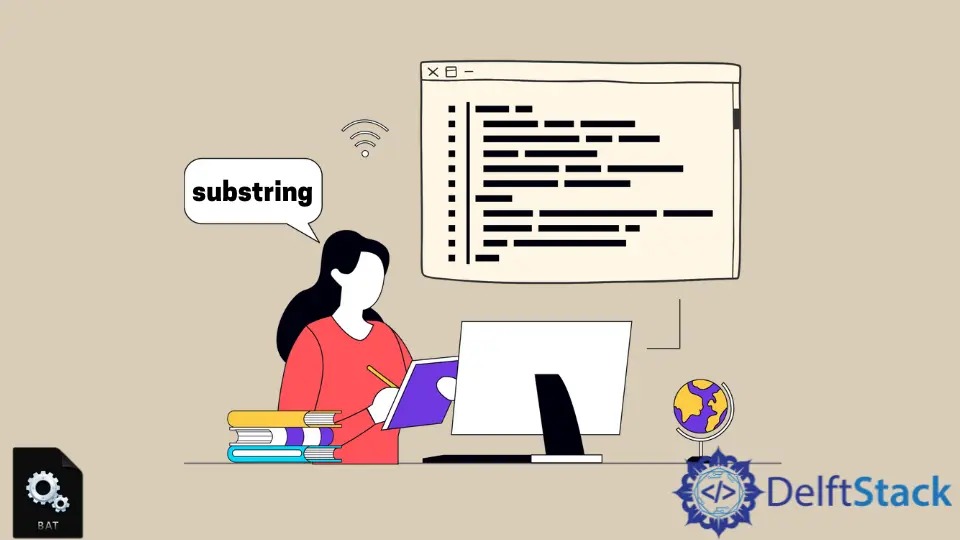
The string is an array of characters, and the substring is a specific part of the string. Sometimes we need to retrieve substrings for various purposes like matching or finding specific substrings.
This article will tackle the string’s methods to get a substring.
Retrieve Substring From a String in Batch Script
We need to follow these general formats to retrieve specific characters from a string variable.
%VARIABLE:~START_INDEX%
or
%VARIABLE:~START_INDEX,END_INDEX%
The START_INDEX
is a numeric value indicating the string’s starting point. You can use negative numbers, but a negative number will count backward from the ending point of the string.
The END_INDEX
is a numeric value indicating the string’s ending point. You can use negative numbers, but a negative number will count backward from the ending point of the string.
Remember, the indexing starts from 0
and not 1
.
In the following example, we will declare a string variable containing numeric and alphabetic characters. We’ll retrieve the numeric and alphabetic characters from the string as a substring.
Batch Script:
@echo off
SET testString=abcdefgh123456789
SET numericChars=%testString:~8,16%
SET normalChars=%testString:~0,7%
ECHO Numeric characters: %numericChars% Alphabetic characters: %normalChars%
In this 1st line code(SET testString=abcdefgh123456789
), we declared a variable named testString
and assigned it with numeric and alphabetic characters.
In this 2nd line code(SET numericChars=%testString:~8,16%
), we retrieve the numeric character part of the string, which is 123456789
, and assign the result to a variable named numericChars
. The start index is 8
, and the ending index is 16
.
3rd line of code(SET normalChars=%testString:~0,7%
), we retrieved the alphabetic character part of the string abcdefgh
and assigned the result to a variable named normalChars
. The start index is 0, and the ending index is 7.
We printed our results with this last code line (ECHO Numeric characters: %numericChars% Alphabetic characters: %normalChars%
).
Output:
Numeric characters: 123456789 Alphabetic characters: abcdefg
Remember the example here are written using Batch Script and only work in a windows CMD environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn