How to Remove X Characters of a File Name using Batch
- File Explorer Rename on Windows
- Bulk Rename Files Using Command Prompt
- Batch File Remove X Characters of a File Name
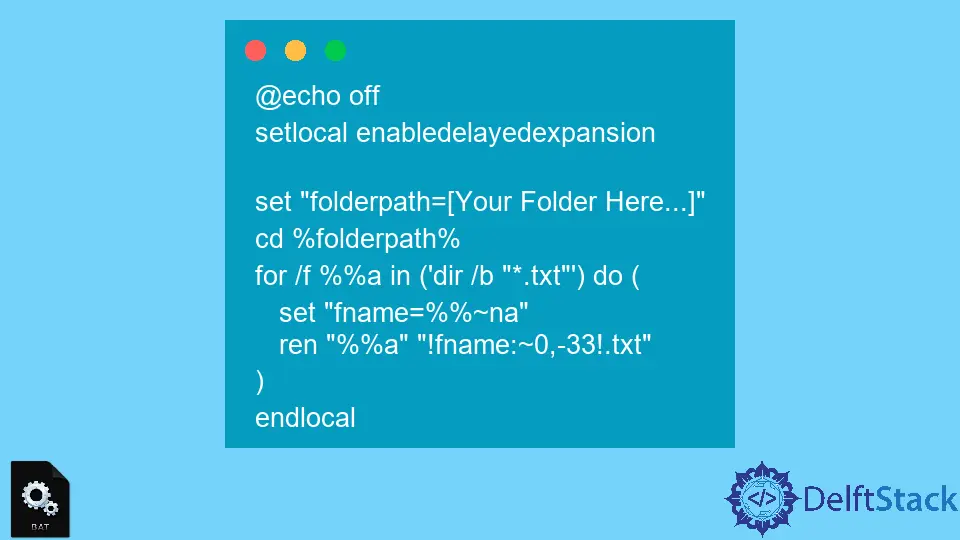
This article illustrates how we can remove specific characters from a file’s filename with a Batch script. We will cover several ways of renaming files on Windows below.
File Explorer Rename on Windows
The least flexible renaming choices are provided by File Explorer, despite being one of the simplest.
- Navigate to the folder that contains the files you want to rename.
- Put the files in the desired renaming order.
- Select all of the files in the folder using CTRL+A, then pick the
Rename
option from the context menu. - Enter the new file name after typing it in.
Bulk Rename Files Using Command Prompt
Windows Command Prompt is a special tool to perform commands, repair files, run Batch files, and start scripts. You can perform amazing tasks with the right syntax, like managing and fixing disk partitions, running applications, and even Batch renaming files.
Here’s how to rename files in Batch in Windows using the Command Prompt.
For Batch file renaming, the Windows Command Prompt provides a little more versatility.
The ren
command allows you to rename several files at once. Rename is abbreviated as ren
.
Although you can change file extensions and use the wildcard characters *
and ?
with this command, you cannot move files into new folders after renaming them.
Rename a Single File
To rename a single file, use the command below.
ren filename.jpg newfilename.jpg
Rename Digits in Multiple Files
You can modify the names of numerous files by using wildcard characters. For instance, you can use the following command to alter the number of digits in your file names.
ren document??.txt document3??.txt
The program can locate any matching files while also producing the renamed files because the question mark wildcard behaves as any character in this case.
Batch Rename Files With a Suffix
Consider adding a suffix to a collection of files. You can accomplish that by issuing the command:
ren *.* ???????-test.*
The asterisk wildcard replaces any characters in this command. *.*
denotes the ability to search for any file in this folder with any extension.
The command is instructed to utilize existing file names up to seven characters in the second portion (complete with all the question marks) but to append -test
as a suffix. The asterisk once more instructs the command to apply to any file extension.
Move the -test
element of the command to the front if you want to add a prefix, like in the following example:
ren *.* test-???????.*
Batch File Remove X Characters of a File Name
You can also remove a portion of a filename using Batch file renaming. Consider a set of files with names like "jan-budget.xlsx", "feb-budget.xlsx", "mar-budget.xlsx"
and so forth.
The"-budget"
suffix can be removed using the following command.
ren ???-budget.xlsx ???.xlsx
Let’s look at a practical example.
Assuming we have a directory with a file, we want to remove the last 33 characters of each file name. How do we go about it?
Here is a Batch file we can use to accomplish the above.
@echo off
setlocal enabledelayedexpansion
set "folderpath=[Your Folder Here...]"
cd %folderpath%
for /f %%a in ('dir /b "*.txt"') do (
set "fname=%%~na"
ren "%%a" "!fname:~0,-33!.txt"
)
endlocal
The above script will remove the last 33 characters of the file contained in the specified folder.
In a nutshell, we can use the ren
command to Batch file rename multiple files on Windows.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn