If ELSE Condition in Batch Script
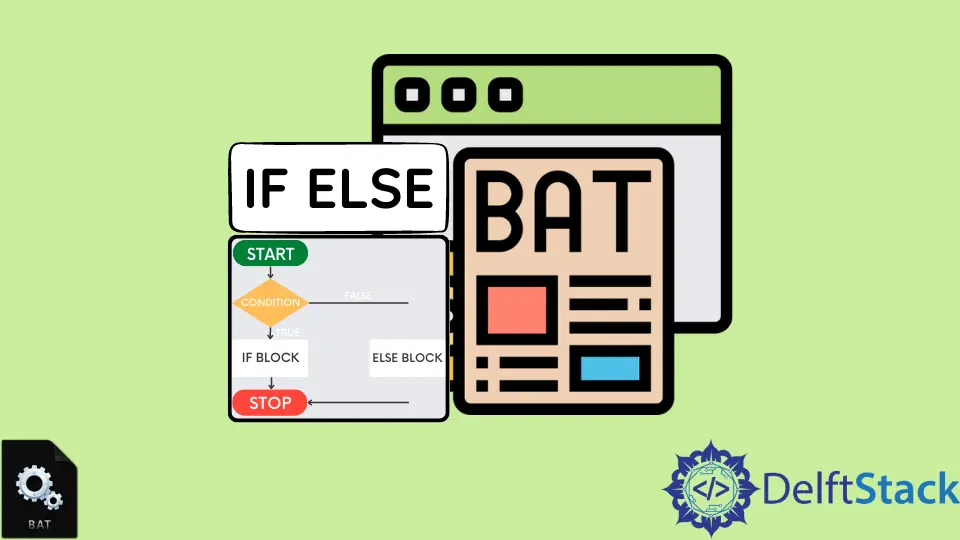
This article will discuss the most used IF ... ELSE
condition and see an example regarding the IF ... ELSE
command.
Use the IF ELSE
Condition in a Batch Script
The general format for the IF ... ELSE
command is something like this IF [CONDITION] [COMMANDS] ELSE [COMMANDS]
. Let’s have an example and describe it for better understanding.
We’ll sum two variables in this example and check whether the result is less than 20.
Batch Script:
SET /A x = 10
SET /A y = 5
SET /A z = %x% + %y%
ECHO Sum of a and b is %z%
IF %z% LSS 20 (echo The result is less than 20) ELSE (echo The result is greater than 20)
In the line SET /A x = 10
, we created an arithmetic variable x
and assigned it with the value of 10
. On the next line, SET /A y = 5
, we declared another variable y
and assigned it with the value of 5
.
SET /A z = %x% + %y%
this line declares another variable which is z
that holds the resulting value of the sum of x
and y
. The next line ECHO Sum of a and b is %z%
shows the output of the result of the sum of x
and y
.
The last line IF %z% LSS 20 (echo Result is less than 20) ELSE (echo Result is greater than 20)
is an IF ... ELSE
command that checks whether the value of c
which holds the resulting value of the sum of x
and y
is less then 20
. If the result is less than 20, it will print Result is less than 20
; otherwise, it will print Result is greater than 20
.
You can notice that we used the keyword LSS
. This keyword is an operator that stands for Less Than
, and it returns true
when the left-side value is less than the right-side value.
Output:
The result is less than 20
Operators We Can Use With the IF ELSE
Command
You can use relational operators with the IF ... ELSE
command.
EQU
- EqualsNEQ
- Not EqualsLSS
- Less thanLEQ
- Less than or equalsGTR
- Greater thanGEQ
- Greater than or equals
Also, we have logical operators that you can use with the IF ... ELSE
command.
AND
- The logicalAND
operatorOR
- The logicalOR
operatorNOT
- The logicalNOT
operator
Note that you can use ==
as equals too. Then you need not use the keyword EQU
.
The example discussed here is written using Batch Script and only work in a windows CMD environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn