How to Use IF ELSE and GOTO in Batch Script
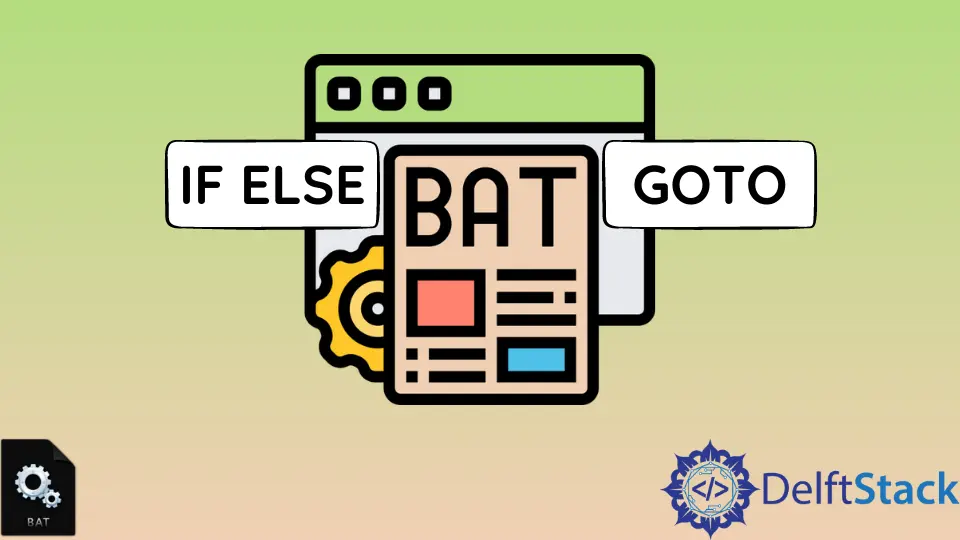
In this article, we’ll combine IF ELSE
and GOTO
commands to understand how we can make these commands work together by providing an example with an explanation for better understanding.
Use IF ELSE
and GOTO
Statement in Batch Script
IF ... ELSE
is a conditional command. Besides, GOTO
is a keyword through which you can skip specific parts of a code from execution.
The general format for IF ... ELSE
is IF [CONDITION] [COMMANDS] ELSE [COMMANDS]
, and the general format for GOTO
is GOTO LABEL
. The LABEL
is the specific point where you want to start from.
In our example, we’ll make a sum of two values and check whether they are less than 20. If it’s less than 20, we will show a message that the resulting value is less than 20.
Otherwise, we will show a message that the message is greater than 20.
Batch Script:
@echo off
SET /A x = 10
SET /A y = 25
SET /A z = %x% + %y%
ECHO The Sum of a and b is %z%
IF %z% LSS 20 ( GOTO :lessThan )
echo The result is greater than 20
GOTO :end
:lessThan
echo The result is less than 20
:end
In the line SET /A x = 10
, we created an arithmetic variable x
and assigned it with the value of 10
. On the next line, SET /A y = 5
, we declared another variable y
and assigned it with the value of 5
.
SET /A z = %x% + %y%
this line declares another variable which is z
that holds the resulting value of the sum of x
and y
. The next line ECHO Sum of a and b is %z%
shows the output of the result of the sum of x
and y
.
IF %z% LSS 20 ( GOTO :lessThan )
this line checks whether the resulting value is less than 20. If it’s less than 20, it will skip the next line and start from the tag lessThan
where we show a message The result is less than 20
.
Otherwise, It will print a message The result is greater than 20
, and through the line GOTO :end
, the code will jump to the tag :end
.
Output:
The Sum of a and b is 35
The result is greater than 20
The methods discussed in this article are written using a Batch Script and only work in a windows CMD environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn