How to Get the Date in Batch Script
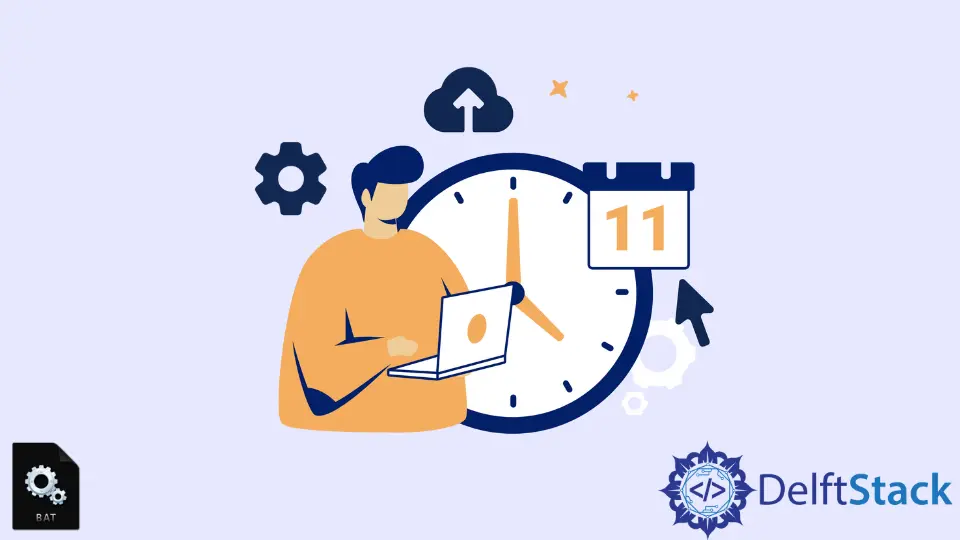
Sometimes, we need to find the date for various purposes of the system. We can get the current date easily by using the Batch Script.
In this article, we will see how we can get the date using the Batch Script. Also, we will see necessary examples and explanations to make the topic easier to understand.
Get the Date in Batch Script
In the example below, we will write a program in Batch that will provide the current date. Our code will look like this:
@echo off
ECHO Today is - %DATE%
When you run the program, the program will show the output below.
Output:
Today is - Wed 06/01/2022
An Advanced Example to Get the Date in Batch Script
This code will provide the date in a default format, MM/DD/YYYY
. If you want to get the date in a different format like DD/MM/YYYY
or YYYY/MM/DD
, you must follow the example below.
This is a bit advanced example that enables you to customize the date format. The code will look like this:
@ECHO off
SET YY=%DATE:~-4%
SET MM=%DATE:~-7,2%
SET DD=%DATE:~-10,2%
ECHO In format YYYY/MM/DD - %YY%-%MM%-%DD%
ECHO In format DD/MM/YYYY - %DD%-%MM%-%YY%
The whole date is provided as a string. So, to organize the data in our format, we have to trim the Day, Month, and Year parts from the string, and in our example, we already did that in our first three lines.
Firstly, we trimmed the Year part, then the Month part, and lastly, the Day part. Finally, we organize the data in our format.
If you run the example, you will get an output like the one below.
Output:
In format YYYY/MM/DD - 2022-01-06
In format DD/MM/YYYY - 06-01-2022
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn