How to Set Command Timeout in Batch
-
Method 1: Using the
timeout
Command - Method 2: Using PowerShell to Enforce a Timeout
- Method 3: Implementing a Loop with Timeout Logic
- Conclusion
- FAQ
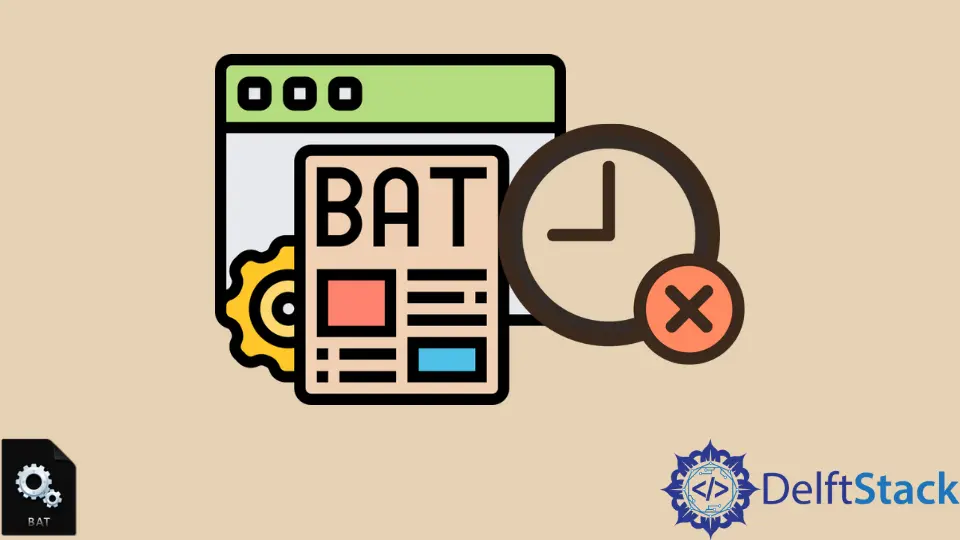
Setting a command timeout in Batch scripts can be crucial for maintaining the efficiency and reliability of your automated processes. Whether you’re running long commands or managing complex operations, having a timeout can prevent your scripts from hanging indefinitely.
In this article, we will explore various methods to set command timeouts specifically within the context of Git commands. You’ll learn how to implement these timeouts effectively, ensuring your Batch scripts remain robust and responsive. Let’s dive into the world of Batch scripting and discover how to manage command timeouts seamlessly.
Before we get into the specifics, it’s essential to understand what a command timeout is. In simple terms, a command timeout is a predefined period during which a command must be executed. If the command exceeds this time limit, it will be terminated automatically. This feature is particularly useful when working with Git commands in a Batch script, as it helps avoid situations where a script could hang due to unresponsive commands.
Method 1: Using the timeout
Command
One of the simplest ways to set a command timeout in a Batch file is by using the timeout
command. This command allows you to specify a wait time in seconds before proceeding to the next command. Here’s how you can implement it:
@echo off
setlocal
set TIMEOUT_DURATION=30
git clone https://github.com/example/repo.git
timeout /t %TIMEOUT_DURATION% /nobreak
echo Clone operation timed out.
Output:
Clone operation timed out.
In this example, we set a timeout duration of 30 seconds. The git clone
command attempts to clone a repository from GitHub. If the command does not complete within the specified time, the script will proceed to the next line, printing a message that the clone operation has timed out. This method is straightforward and effective for simple timeout needs.
The timeout
command is a built-in utility in Windows, making it widely accessible. However, it doesn’t actually terminate the Git command; it merely waits for a specified duration before moving on. If you need a more forceful approach to terminate the command, consider using the next method.
Method 2: Using PowerShell to Enforce a Timeout
If you’re looking for a more robust solution that can actually terminate a command after a timeout, PowerShell is a great option. You can invoke a PowerShell command from your Batch script to achieve this. Here’s how:
@echo off
setlocal
set TIMEOUT_DURATION=30
powershell -Command "Start-Process git -ArgumentList 'clone https://github.com/example/repo.git' -Wait -Timeout %TIMEOUT_DURATION%"
echo Clone operation completed or timed out.
Output:
Clone operation completed or timed out.
In this example, we use PowerShell’s Start-Process
cmdlet to initiate the git clone
command. The -Wait
parameter ensures that the script waits for the command to complete, while the -Timeout
parameter specifies the maximum duration allowed for the command to run. If the command exceeds this duration, it is terminated, and the script prints a message indicating that the operation has either completed or timed out.
Using PowerShell provides a more controlled environment for managing command timeouts. It allows you to enforce stricter limits and handle scenarios where commands may hang indefinitely. This method is particularly useful for more complex Batch scripts that require precise control over command execution.
Method 3: Implementing a Loop with Timeout Logic
Another effective way to set a command timeout in Batch scripts is to implement a loop that checks the status of the command over time. This method offers flexibility and control, allowing you to customize the timeout behavior. Here’s an example:
@echo off
setlocal
set TIMEOUT_DURATION=30
set START_TIME=%TIME%
git clone https://github.com/example/repo.git
:checkTimeout
set CURRENT_TIME=%TIME%
call :timeDiff START_TIME CURRENT_TIME DIFF
if %DIFF% lss %TIMEOUT_DURATION% (
timeout /t 1 >nul
goto checkTimeout
)
echo Clone operation timed out.
exit /b
:timeDiff
setlocal
set /a "start=%1"
set /a "current=%2"
set /a "diff=current-start"
endlocal & set %3=%diff%
exit /b
Output:
Clone operation timed out.
In this example, we define a loop that continuously checks whether the elapsed time has exceeded the specified timeout duration. The :timeDiff
subroutine calculates the difference between the start time and the current time. If the difference is less than the timeout duration, the script waits for one second and checks again. If the timeout is reached, it prints a message indicating that the clone operation has timed out.
This method offers a high degree of customization and can be adapted for various scenarios. It allows you to set different timeout durations for different commands, making your Batch scripts more versatile and powerful.
Conclusion
Setting command timeouts in Batch scripts is an essential practice for ensuring that your automated processes run smoothly and efficiently. Whether you choose to use the simple timeout
command, leverage PowerShell for more robust control, or implement a custom loop for timeout logic, each method has its advantages. By incorporating these strategies into your Batch scripts, you can avoid potential pitfalls and enhance the reliability of your automation tasks. Remember, the key is to choose the method that best fits your specific needs and workflow.
FAQ
-
What is a command timeout in Batch scripts?
A command timeout is a predefined period during which a command must complete execution. If it exceeds this time limit, the command is terminated. -
Can I set a timeout for any command in Batch scripts?
Yes, you can set a timeout for any command in Batch scripts using various methods, including the timeout command, PowerShell, or custom loops. -
Why would I use PowerShell for command timeouts?
PowerShell offers more control and can actually terminate commands that exceed the specified timeout, making it a more robust solution compared to the simple timeout command. -
Is it possible to customize timeout durations for different commands?
Yes, you can customize timeout durations for different commands by implementing loops or using variables to set different timeout values. -
Can I use these methods for commands other than Git?
Absolutely! While this article focuses on Git commands, the methods discussed can be applied to any command in Batch scripts.