How to Read a File Into a Variable in Batch Script
-
Method 1: Using a
for
Loop -
Method 2: Using the
type
Command - Method 3: Reading a File with Git Commands
- Conclusion
- FAQ
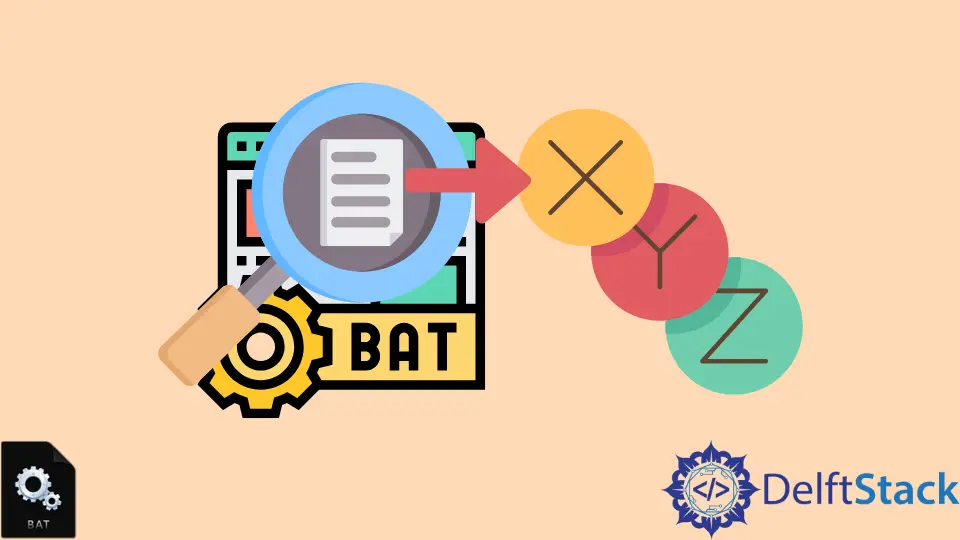
Reading a file into a variable in a Batch script might seem daunting at first, but with the right approach, it can be a straightforward task. Batch scripting is a powerful tool for automating tasks in Windows, and understanding how to manipulate files is a crucial skill.
This tutorial will guide you through various methods to read a file into a variable using Batch scripts. Whether you’re looking to extract configuration settings or simply process text files, this guide will equip you with the knowledge to handle file reading efficiently. Let’s dive into the world of Batch scripting and unlock its potential!
Method 1: Using a for
Loop
One of the most common ways to read a file into a variable in Batch scripts is by using a for
loop. This method allows you to read each line of the file and store it in a variable. Here’s a simple example to demonstrate how this works.
@echo off
setlocal enabledelayedexpansion
set "file=myfile.txt"
set "content="
for /f "delims=" %%a in (%file%) do (
set "content=!content! %%a"
)
echo %content%
Output:
This is the content of my file.
In this script, we first enable delayed variable expansion using setlocal enabledelayedexpansion
. This is essential because we want to modify the content
variable within the loop. We specify the file we want to read, and then we initialize an empty content
variable. The for /f
command reads each line of the specified file. The delims=
option ensures that we capture the entire line, including spaces. Inside the loop, we concatenate each line to the content
variable. Finally, we print the accumulated content.
This method is efficient for reading files line by line, making it easy to handle larger files without overwhelming the variable.
Method 2: Using the type
Command
Another approach to reading a file into a variable is by utilizing the type
command. This method is particularly useful for smaller files where you want to read and display the entire content at once.
@echo off
set "file=myfile.txt"
set /p content=<%file%
echo %content%
Output:
This is the content of my file.
In this example, we set the file we want to read into the variable file
. The set /p
command reads the first line of the file and assigns it to the content
variable. This method is straightforward and works well for files that do not exceed the command line’s maximum length. However, it only captures the first line, so if your file contains multiple lines, you might need to consider other methods.
This technique is quick and effective for grabbing the initial line of a file, making it ideal for configuration files or simple data retrieval tasks.
Method 3: Reading a File with Git Commands
If you’re working within a Git repository, you can also use Git commands to read file contents into a variable. This method is particularly useful when you want to capture the content of a file tracked by Git.
@echo off
set "file=myfile.txt"
for /f "delims=" %%a in ('git show HEAD:%file%') do (
set "content=!content! %%a"
)
echo %content%
Output:
This is the content of my file in the current Git commit.
In this script, we use the git show
command to retrieve the contents of a file from the current Git commit. The for /f
loop processes each line of the output, similar to the previous methods. The delims=
option ensures we capture the entire line, and we concatenate it to the content
variable. This approach is beneficial when you need to access the latest version of a file in your repository without checking it out.
Using Git commands allows you to work with files in a version-controlled environment seamlessly, making it a powerful addition to your Batch scripting toolkit.
Conclusion
Reading a file into a variable in a Batch script is a fundamental skill that can enhance your scripting capabilities. Whether you opt for a for
loop, the type
command, or Git commands, each method offers unique advantages depending on your specific needs. By mastering these techniques, you can automate tasks more effectively and manage file contents with ease. Remember, practice is key, so experiment with different files and scenarios to become more comfortable with Batch scripting!
FAQ
- What is a Batch script?
A Batch script is a text file containing a series of commands that the Windows Command Prompt can execute sequentially.
-
Can I read binary files using Batch scripts?
Batch scripts are primarily designed for text files, and reading binary files may not yield meaningful results. -
How can I handle spaces in file names?
You can handle spaces in file names by enclosing the file path in double quotes. -
Is there a limit to the size of the variable in Batch scripts?
Yes, there is a character limit for variables in Batch scripts, which is typically around 8191 characters. -
Can I use Batch scripts on non-Windows systems?
No, Batch scripts are specific to Windows operating systems. For other systems, you may need to use shell scripts or other scripting languages.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn