How to Prompt User Input and Use Results in Batch Script
- Understanding User Input in Batch Scripts
- Using User Input to Execute Commands
- Validating User Input in Batch Scripts
- Advanced User Input Techniques
- Conclusion
- FAQ
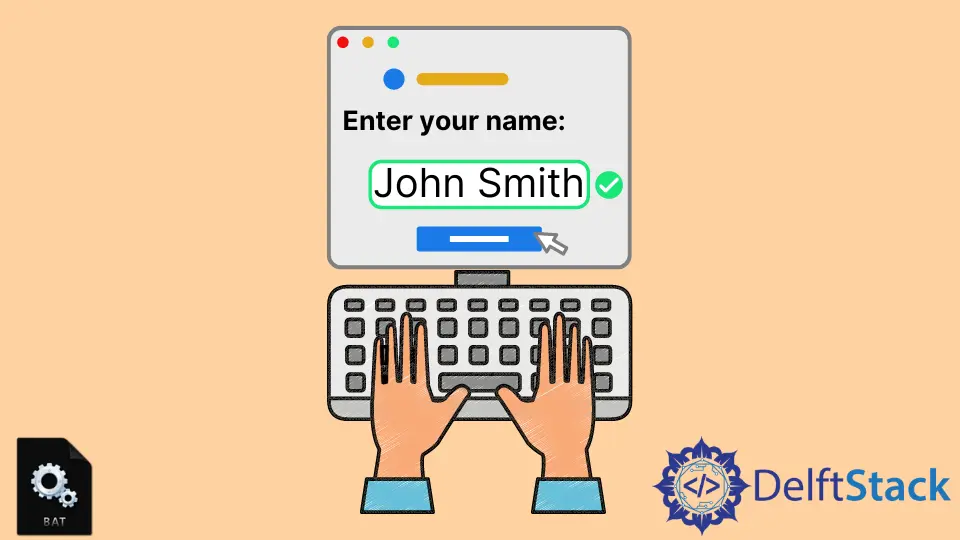
In the world of scripting, automating tasks can significantly enhance productivity. One of the most useful features of a batch script is its ability to take user input and utilize that data in various commands. Whether you’re creating a simple script to gather user information or a more complex program that executes commands based on user input, mastering this skill is essential.
This tutorial will guide you through the process of prompting for user input in a batch script and using the results effectively. By the end of this article, you’ll have a solid understanding of how to incorporate user input into your scripts, enhancing their functionality and user experience.
Understanding User Input in Batch Scripts
Before diving into the code, it’s essential to understand how batch scripts handle user input. In a batch script, the SET
command is used to assign values to variables. To prompt the user for input, we use the SET /P
command, which allows us to display a message and read the user’s response. This input can then be used in subsequent commands.
Here’s a simple example to illustrate this:
@echo off
SET /P userInput="Please enter your name: "
echo Hello, %userInput%!
Output:
Please enter your name: John
Hello, John!
In this example, the script prompts the user to enter their name. The input is stored in the variable userInput
, which is then used in an echo command to greet the user. This simple interaction demonstrates how user input can make your scripts more dynamic and engaging.
Using User Input to Execute Commands
Now that we understand how to capture user input, let’s explore how we can leverage that input to execute commands. This method can be particularly useful when you want to perform different actions based on user responses.
Consider the following batch script, which allows users to choose a directory to navigate to:
@echo off
SET /P dirChoice="Enter a directory name (e.g., Documents, Downloads): "
cd %dirChoice%
echo You are now in the %dirChoice% directory.
Output:
Enter a directory name (e.g., Documents, Downloads): Documents
You are now in the Documents directory.
In this script, the user is prompted to enter a directory name. The cd
command uses the input to change the current directory accordingly. This approach makes the script interactive and allows users to navigate their file system efficiently. The flexibility of using user input in commands can significantly enhance the usability of your batch scripts.
Validating User Input in Batch Scripts
While capturing user input is straightforward, validating that input is crucial to ensure your script runs smoothly without errors. You can implement basic validation by checking if the user input is empty or if it matches a specific format. Here’s an example that checks if the user has entered a valid directory:
@echo off
SET /P dirChoice="Enter a directory name: "
IF EXIST "%dirChoice%" (
cd "%dirChoice%"
echo You are now in the %dirChoice% directory.
) ELSE (
echo The directory "%dirChoice%" does not exist. Please try again.
)
Output:
Enter a directory name: NonExistentFolder
The directory "NonExistentFolder" does not exist. Please try again.
In this script, the IF EXIST
command checks whether the directory entered by the user actually exists. If it does, the script changes the directory; if not, it informs the user that the directory does not exist. This validation step is essential in making your scripts robust and user-friendly, preventing potential errors that could disrupt the flow of execution.
Advanced User Input Techniques
For more advanced scenarios, you can create scripts that handle multiple inputs or even loop until valid input is received. This can be particularly useful in situations where you want to ensure the user provides the correct data before proceeding. Here’s an example of a script that continues to prompt the user until they enter a valid number:
@echo off
:askUser
SET /P userInput="Please enter a number between 1 and 10: "
IF "%userInput%" GEQ "1" IF "%userInput%" LEQ "10" (
echo You entered a valid number: %userInput%.
) ELSE (
echo Invalid input. Please try again.
GOTO askUser
)
Output:
Please enter a number between 1 and 10: 15
Invalid input. Please try again.
Please enter a number between 1 and 10: 5
You entered a valid number: 5.
In this script, the :askUser
label allows the script to loop back to the input prompt if the user enters a number outside the specified range. The use of GOTO
effectively creates a loop, enhancing user interaction by ensuring they provide valid input before the script continues. This method not only strengthens the user experience but also minimizes errors in execution.
Conclusion
Incorporating user input into batch scripts is a powerful way to enhance their interactivity and functionality. By utilizing commands like SET /P
, IF EXIST
, and GOTO
, you can create scripts that respond dynamically to user actions. Whether you’re prompting for a name, navigating directories, or validating numbers, these techniques open up a world of possibilities for automating tasks and improving user engagement. With practice, you’ll find that user input can significantly elevate the effectiveness of your batch scripts.
FAQ
-
How do I capture user input in a batch script?
You can capture user input using theSET /P
command, which prompts the user for input and stores it in a variable. -
Can I validate user input in a batch script?
Yes, you can validate user input using conditional statements likeIF
to check for specific criteria or existence. -
What happens if the user enters invalid input?
You can handle invalid input by providing error messages and prompting the user to try again using loops. -
Are there any limitations to user input in batch scripts?
Batch scripts have limitations in terms of data types and complex validations compared to more advanced scripting languages. -
How can I improve the user experience in my batch scripts?
You can enhance user experience by providing clear prompts, validating inputs, and handling errors gracefully.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn