How to Loop Through Files in Subdirectories using Batch
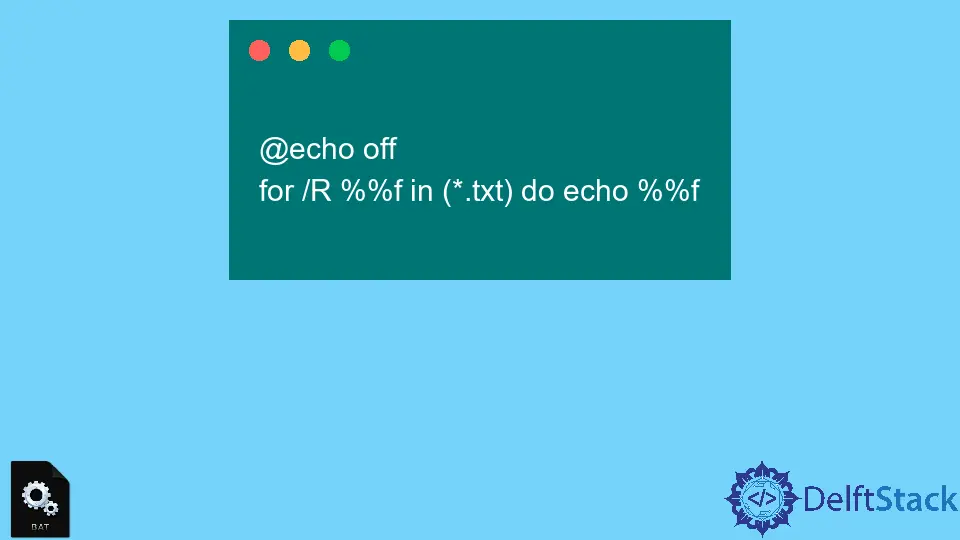
This article illustrates how we can write a Batch script that loops through files in subdirectories. We will cover an example to explain the concept.
Batch File to Loop Through Files in Subdirectories
Let’s assume we have the directory structure illustrated below.
- Main directory (Contains our .bat file and some top-level directories)
- Sub-directory
- Search Directory
- A bunch of files (Files we want to loop through)
We can get the list of files and folders using the command below in our Main directory
.
for /f %%f in ('dir /b /r *') do echo %%f
However, we want the files in the Search directory
. Here’s an example of the structure of a file in the Search directory
.
C:\Users\pc\Search16\0045\search\FP585.txt
Instead of using the /f
, as illustrated in the previous example command, we can use /R
to loop through all files in all subdirectories, as illustrated below.
@echo off
for /R %%f in (*.txt) do echo %%f
The command above will display a list of all the files with a .txt
file extension in all subdirectories.
In conclusion, as discussed above, we can substitute /R
for /f
when writing Batch commands to loop through files in all subdirectories.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn