The For Loop in Batch Script
-
Use the Default
FOR
Loop in Batch Script -
Use the
FOR /L
Loop in Batch Script -
Use the
FOR /R
Loop in Batch Script -
Use the
FOR /D
Loop in Batch Script -
Use the
FOR /F
Loop in Batch Script
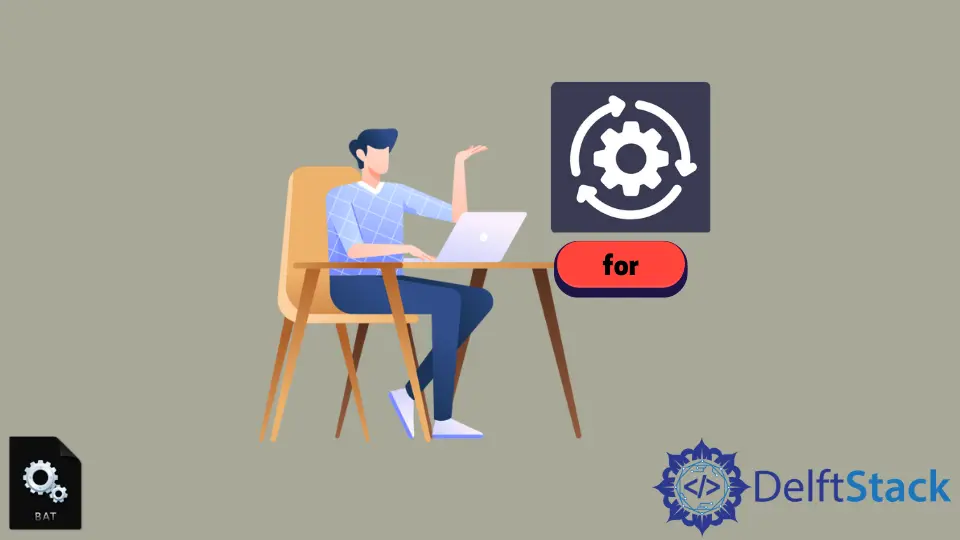
This tutorial will show how to use a FOR
loop in a Batch Script.
Loops are used to execute a specific task continuously until it reaches the number of times specified by the programmer. This FOR
loop has different versions.
Use the Default FOR
Loop in Batch Script
This is the default format that iterates over a list of files. The general format is shown below.
FOR %%variable IN ( File_directory_01 File_directory_02 ) DO command
In the below example, we will copy the list of files to another directory.
Example:
@echo off
FOR %%f IN (E:\testFile\fileOne.data E:\testFile\fileTwo.txt) DO (
copy %%f E:\newDir
)
pause
Use the FOR /L
Loop in Batch Script
Mainly used to provide a list of numbers, the general format or syntax to use the FOR /L
loop is shown below.
FOR /L %%var_name IN (StartValue, Increment, LimitValue) DO YOUR_CODE
Example:
@echo off
FOR /L %%v IN (1 1 5 ) DO (
echo %%v
)
pause
Output:
1
2
3
4
5
Press any key to continue . . .
Use the FOR /R
Loop in Batch Script
This FOR
loop version provides a list of files in a directory after filtering on a specific file type. The general format is provided in the following.
FOR /R "path" %%variable IN ( Filters of file_type ) DO command
The below example provides all the files with the types .txt
and .log
.
Example:
@echo off
FOR /R "C:\Directory" %%F IN (*.txt *.log) DO (
echo %%F
)
pause
Use the FOR /D
Loop in Batch Script
This provides a list of directories. The general format for this is the following.
FOR /D %%variable IN ( directory_filters ) DO command
The below example will list out all the subdirectories of a specified directory.
Example:
@echo off
FOR /D %%v IN ( * ) DO (
echo %%v
)
pause
Use the FOR /F
Loop in Batch Script
This is the complex form that reads the contents of a specific file. The general format to use the FOR /F
loop is shown below.
FOR /F "Criteria" %%i IN ( file.txt ) DO
The below example will read the content of the specified file and show the output based on the criteria.
Example:
@echo off
FOR /F "age=23" %%i IN ( persons.txt ) DO (
echo Full Name: %%i %%j Gender: %%k
)
pause
The full name is divided into two parts: the first and last names.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn