For \F in Batch Script
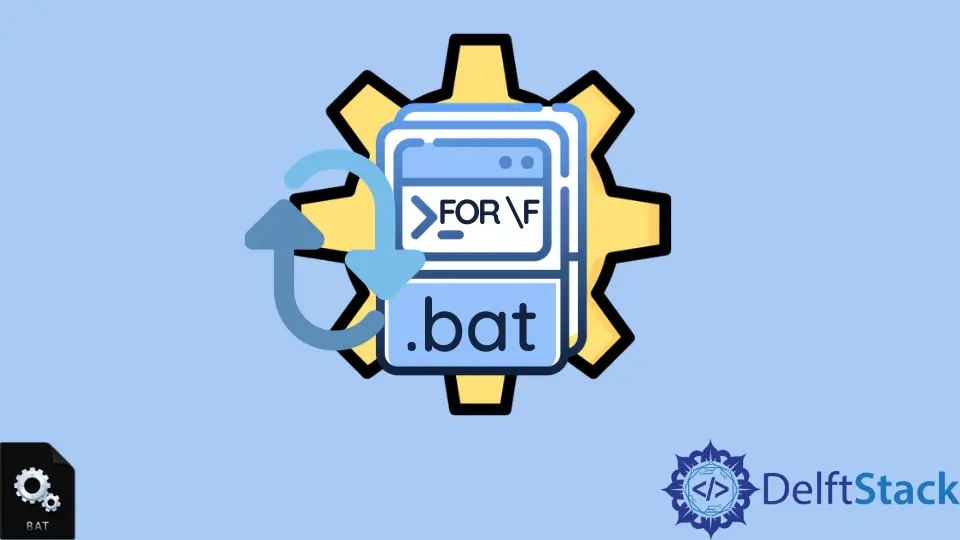
This article will discuss using the FOR /F
loop in Batch Script.
Use the FOR /F
Loop in Batch Script
The general format for this type of FOR
loop is shown below:
FOR /F ["YOUR_OPTIONS_HERE"] %%PEREMETER IN (file_name_here) DO (
command
)
Let’s see the options available for this command and an example. The options for this command are shown below:
DELIMS=xxx
- Provides the delimiter characters; contains a default value for strings,SPACE
orTAB
.SKIP=n
- Specifies the number of lines that should be skipped.TOKENS=n
- Holds the line numbers that should be checked or read.
The below example finds the Name
and Gender
from a text file. Here, tokens=1,2
checks the first and second lines, and delims=;
excludes the symbol ;
every time it is found.
Example:
@echo off
FOR /F "tokens=1,2 delims=;" %%i IN ( test_list.txt ) DO (
echo Full Name: %%i %%j Gender: %%k
)
pause
Here the full name is divided into two parts: First name and Last name.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn