Equivalent of Batch Script for macOS
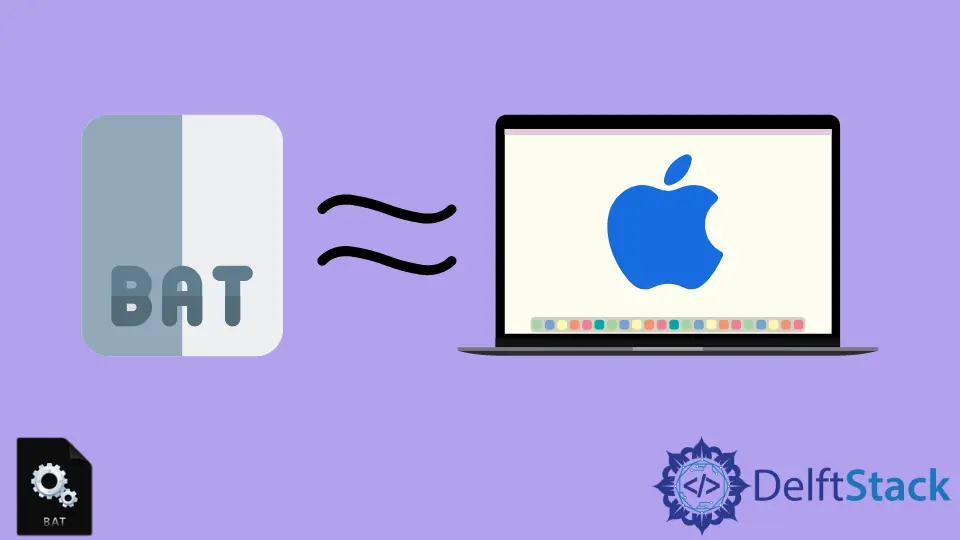
For Windows, we have a specialized scripting language name Batch, whose file extension is .bat
. On the other hand, we have a specialized scripting language for Linux, Bash, with the extension .sh
.
This article will introduce a scripting language for macOS similar to Bash and Batch, AppleScript.
Equivalent of Batch Script for macOS
AppleScript is a scripting language created by Apple specially built for macOS operating system environment. Through this scripting language, a user can directly control scriptable Macintosh applications.
It’s also a part of macOS. Using this scripting language, you can create scripts with a set of instructions, automate repetitive tasks, combine features, and many more.
Below shared a simple AppleScript to print Hello World !!!
. The code for the example is:
tell application "My Application"
display alert "Hello world!!!"
end tell
The equivalent for this code in Batch looks like this:
@echo off
ECHO Hello world!!!
Both codes will display the same output, but the AppleScript code will show the output in a popup alert.
Output:
Hello world!!!
An Advanced Example of AppleScript
This is an advanced example where we focused on AppleScript’s GUI feature. In our example below, we will display a popup message with some text.
The AppleScript code for this example will be like the below.
display dialog "Some text here" with icon stop buttons {"OK"} default button {"OK"}
The above code is a basic notification dialogue with the support of AppleScript’s GUI.
AppleScript contains a small number of commands but support user by providing frameworks through which you can perform many task-specific commands. The extension to AppleScript is .SCPT
.
AppleScript update continuously updates with the update of macOS by the Apple corporation. Below shared some exciting features of AppleScript.
Key Features of AppleScript
- AppleScript supports full Unicode.
- AppleScript enhanced application object.
- AppleScript contains updated scriptable system preferences.
- AppleScript contains numerous functions, including the read and write function.
- AppleScript provides Frameworks support.
- AppleScript provides the support for folder action.
- AppleScript provides descriptive error message support.
- AppleScript supports automating tasks.
Please note that the first code we shared here is written in AppleScript, which can only be run in Apple’s macOS environment, and the second code we shared in this article is written in Batch Script and only runs on the Windows Command Prompt environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn