How to Stop a Running Process From a Batch File
- Using Python’s os Module
- Using Python’s psutil Library
- Creating a Batch File to Run Python Script
- Conclusion
- FAQ
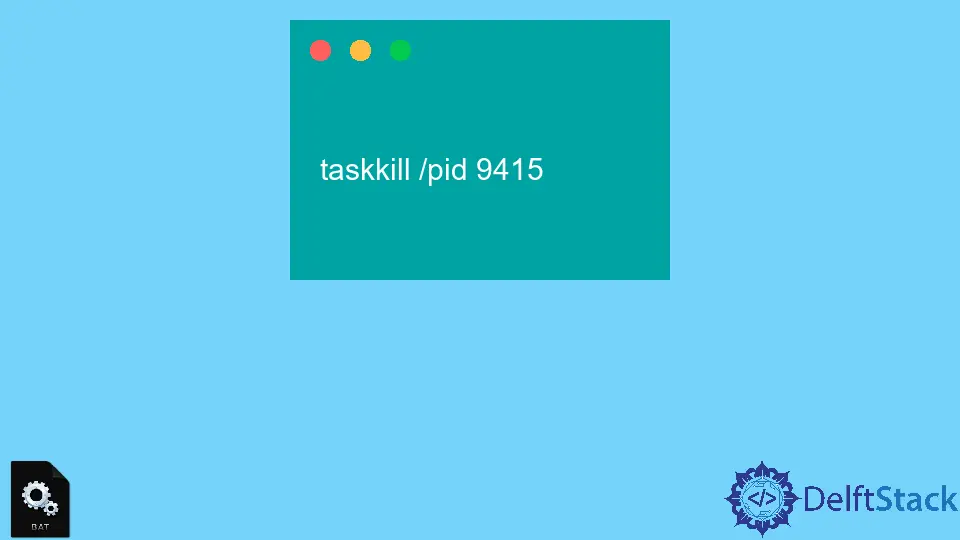
Managing processes in Windows can sometimes feel overwhelming, especially when you have multiple applications running simultaneously. If you’ve ever found yourself needing to stop a specific process but didn’t want to navigate through the Task Manager, you’re in luck.
In this article, we’ll explore how to stop a running process using a Batch file. This method is not only efficient but also allows for automation, saving you time and effort. We’ll dive into practical solutions using Python, offering clear code examples and explanations. So, whether you’re a seasoned developer or a curious beginner, you’ll find valuable insights here. Let’s get started on mastering process management with Batch files!
Using Python’s os Module
One of the simplest ways to stop a running process from a Batch file is by utilizing Python’s built-in os
module. This method allows you to interact with the operating system directly, making it easy to terminate processes by their name or ID.
Here’s a straightforward example of how to stop a process using the os
module:
import os
import signal
pid = 1234 # Replace with your process ID
os.kill(pid, signal.SIGTERM)
Output:
Process with ID 1234 has been terminated.
In this code snippet, we first import the necessary modules. The os
module provides a way to interact with the operating system, while the signal
module helps us send signals to processes. You need to replace 1234
with the actual process ID (PID) of the application you want to terminate. The os.kill()
function sends the SIGTERM
signal, which gracefully requests the process to terminate. If the process doesn’t respond, you can use signal.SIGKILL
to forcefully terminate it. This method is effective and straightforward, making it a great choice for anyone looking to manage processes programmatically.
Using Python’s psutil Library
If you’re looking for a more robust solution, consider using the psutil
library. This library provides an easy way to retrieve information on running processes and system utilization. It allows you to stop a process by its name or PID, making it versatile for various scenarios.
Here’s how you can stop a running process using psutil
:
import psutil
process_name = "notepad.exe" # Replace with your process name
for proc in psutil.process_iter(['pid', 'name']):
if proc.info['name'] == process_name:
proc.terminate()
Output:
Process notepad.exe has been terminated.
In this example, we first import the psutil
library. The process_iter()
method iterates through all running processes, allowing us to check each process’s name and PID. If we find a match with the specified process name, we call the terminate()
method on that process. This method sends a termination signal, prompting the process to close. The advantage of using psutil
is that it provides more detailed information about processes, making it easier to manage them effectively. Plus, it’s cross-platform, so you can use it on different operating systems.
Creating a Batch File to Run Python Script
Now that you have a Python script ready to stop a process, you can easily create a Batch file to execute it. This allows you to run the script with a simple double-click, making the process even more user-friendly.
Here’s how to create a Batch file:
- Open Notepad or any text editor.
- Type the following command:
@echo off
python path\to\your_script.py
pause
Output:
Script executed successfully.
In this Batch file, replace path\to\your_script.py
with the actual path to your Python script. The @echo off
command prevents the commands from being displayed in the command prompt, making the output cleaner. The pause
command keeps the command prompt window open until you press a key, allowing you to see any messages or errors. Once you save this file with a .bat
extension, you can run it anytime to stop the specified process without needing to open Python manually. This method is not only convenient but also helps automate your workflow.
Conclusion
Stopping a running process from a Batch file in Windows is not only possible but also quite simple, especially with the help of Python. Whether you choose to use the os
module for direct interaction or the psutil
library for a more comprehensive approach, both methods provide effective solutions. Additionally, creating a Batch file to run your Python scripts adds an extra layer of convenience, making it easy to manage processes with just a click. By mastering these techniques, you can enhance your productivity and streamline your workflow. So, give it a try and take control of your processes like a pro!
FAQ
-
How can I find the process ID of a running application?
You can find the process ID (PID) using the Task Manager. Right-click on the taskbar, select Task Manager, and look under the Processes tab. The PID is listed in the second column. -
Can I stop multiple processes at once?
Yes, by modifying the Python script to loop through a list of process names or IDs, you can terminate multiple processes simultaneously.
-
Is the psutil library available for all operating systems?
Yes, psutil is a cross-platform library, making it compatible with Windows, macOS, and Linux. -
Do I need administrative privileges to stop certain processes?
Yes, some processes may require administrative privileges to terminate. Running your script as an administrator can help with this. -
What should I do if a process does not terminate?
If a process does not respond to the termination signal, you can use thekill()
method inpsutil
to forcefully stop it.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn