How to Take String Input in Batch Script
-
Using the
SET
Command - Using the Input Redirection
- Using FOR Loop to Capture Multiple Strings
- Conclusion
- FAQ
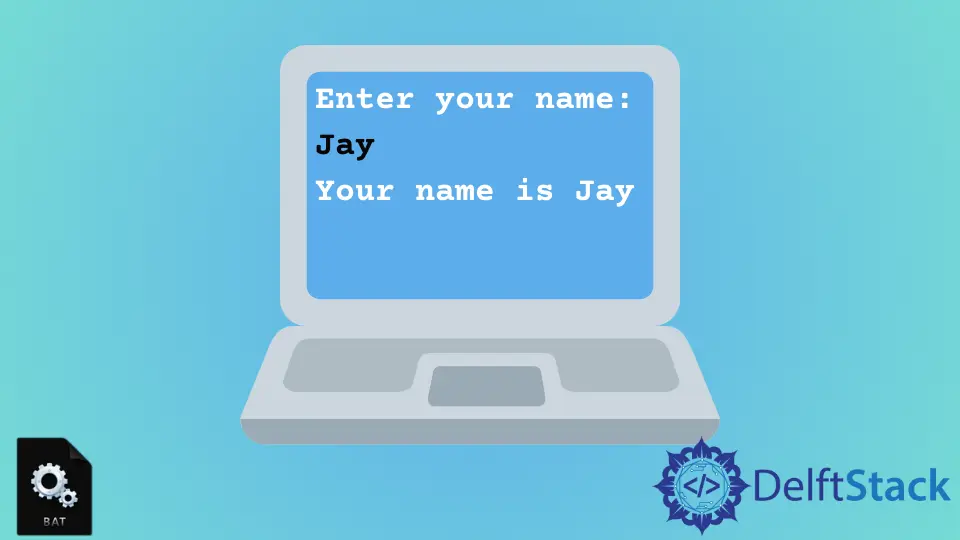
Batch scripting is a powerful tool for automating tasks in Windows environments. One of the fundamental aspects of batch scripts is the ability to take user input, particularly string input. This capability allows you to create more interactive scripts that can respond to user needs.
In this tutorial, we will explore how to take string input in batch scripts, providing you with step-by-step instructions and examples. Whether you are a beginner or have some experience with batch scripting, this guide will help you enhance your scripts and make them more dynamic. Let’s dive in!
Using the SET
Command
The most common method to take string input in a batch script is by using the SET
command. This command allows you to define a variable and assign a value to it, which can be inputted by the user during the script’s execution. Here’s a simple example of how to do this:
@echo off
set /p userInput=Please enter a string:
echo You entered: %userInput%
Output:
You entered: [user's input]
In this example, the script starts with @echo off
, which prevents the commands from being displayed in the command prompt. The set /p
command prompts the user to enter a string and assigns it to the variable userInput
. After the user inputs their string, the script echoes back what they entered. This method is straightforward and effective for capturing string input in batch scripts.
Using the Input Redirection
Another way to take string input in batch scripts is through input redirection. This method is particularly useful for scripts that need to read input from a file or from standard input. Here’s how you can achieve this:
@echo off
echo Please enter a string:
set /p userInput=
echo You entered: %userInput%
Output:
You entered: [user's input]
In this script, we first prompt the user to enter a string. The set /p
command is again used to capture the input. The difference here is that we can redirect input from a file if needed, making this method versatile. For example, if you have a text file containing strings, you can redirect its content into the batch script using the <
operator. This approach allows you to handle larger inputs or multiple strings efficiently.
Using FOR Loop to Capture Multiple Strings
If you need to capture multiple strings in a single run, using a FOR
loop can be an effective solution. This method allows you to iterate through a set of inputs, capturing each one as needed. Here’s an example:
@echo off
setlocal enabledelayedexpansion
set /p count=How many strings do you want to enter?
set i=1
:loop
if !i! leq %count% (
set /p userInput=Enter string !i!:
echo You entered: !userInput!
set /a i+=1
goto loop
)
Output:
You entered: [user's input for string 1]
You entered: [user's input for string 2]
...
In this script, we first ask the user how many strings they want to enter. The setlocal enabledelayedexpansion
command allows us to use the variable !i!
within the loop. The if
statement checks if the current count is less than or equal to the number of strings the user wants to input. The loop continues until the user has entered the specified number of strings, echoing each one back to them. This method is particularly useful for scenarios where you need to gather multiple pieces of input from the user.
Conclusion
Taking string input in batch scripts is a fundamental skill that can greatly enhance your automation scripts. By using methods like the SET
command, input redirection, and loops, you can create interactive and user-friendly scripts. These techniques not only streamline your workflow but also make your scripts more adaptable to different situations. As you gain more experience with batch scripting, you’ll find that these input methods can be combined and modified to suit your specific needs. Happy scripting!
FAQ
-
How do I take user input in a batch script?
You can use theSET /P
command to prompt the user for input and assign it to a variable. -
Can I capture multiple strings in a single execution?
Yes, you can use aFOR
loop to capture multiple strings by iterating based on user input.
-
What is the purpose of
@echo off
in a batch script?
The@echo off
command prevents the script commands from being displayed in the command prompt, making the output cleaner. -
Can I redirect input from a file in a batch script?
Yes, you can use input redirection with the<
operator to read input from a file. -
Is it possible to manipulate the captured string input?
Yes, you can perform various string manipulations using built-in commands in batch scripts.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn