How to Check if a Specified Environmental Variable Contains a Substring
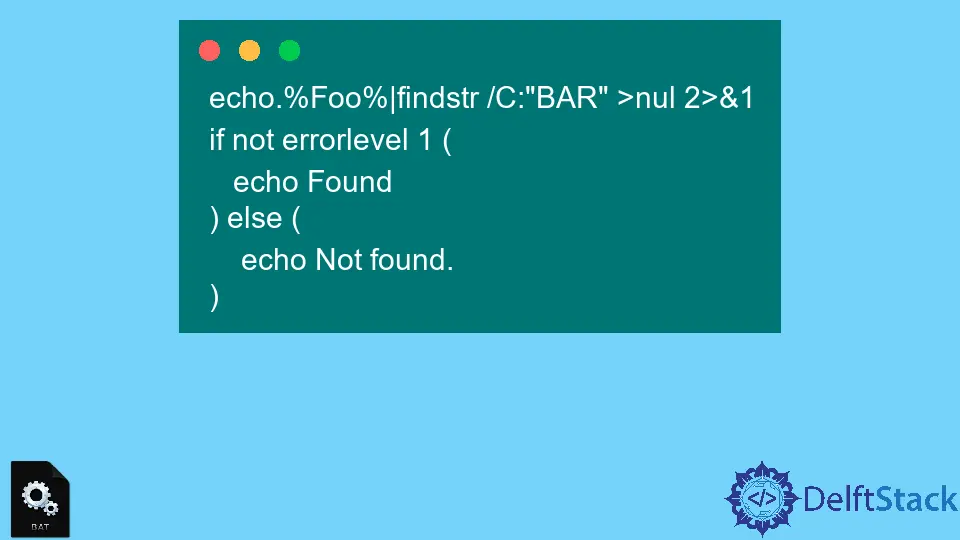
This article discusses how we can use the Batch command to test if a certain environmental variable contains a specific substring. We will cover two Batch scripts we can use in the scenario mentioned above.
Check if a Specified Environmental Variable Contains a Substring
Assuming we have an environmental variable defined as Foo
and want to check if it contains the substring BAR
. How do we go about it?
We can use the trusted findstr
command in such a scenario, as illustrated below.
echo.%Foo%|findstr /C:"BAR" >nul 2>&1 && echo Found || echo Not found.
You can branch instead of echoing, but if you require numerous statements based on that, the following is simpler.
echo.%Foo%|findstr /C:"BAR" >nul 2>&1
if not errorlevel 1 (
echo Found
) else (
echo Not found.
)
The above script will return Found
if the variable contains the specified substring. It will return Not found
if the variable does not contain the substring.
In a nutshell, we have covered two scripts we can use to test if a certain environmental variable contains a specific substring.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedInRelated Article - Batch Variable
- How to Check for Empty Variables in Batch
- How to Read a File Into a Variable in Batch Script
- How to Remove Double Quotes From Variables in a Batch File
- How to Declare Variables in Batch Script