How to Check if a File Exists Using Batch
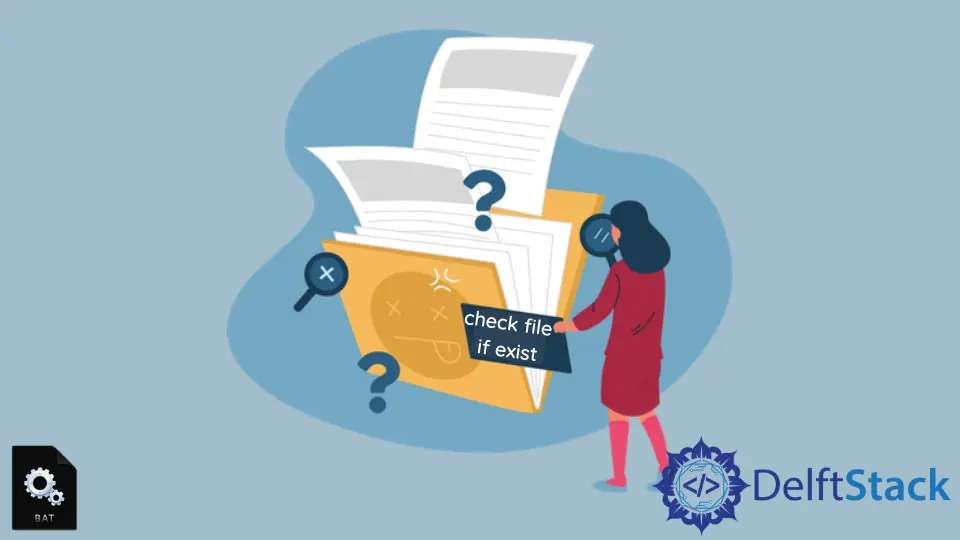
This article will demonstrate checking if a file exists or not using a Batch script through an example code.
Check if a File Exists Using a Batch Script
The general format or syntax for the code to check if a file exists is provided below.
IF EXIST filename.txt (
action if file exists
) ELSE (
action if the file doesn't exist
)
The below example will check whether the file simple.txt
exists or not. If the file exists, it will show the message File exists!!!
; if the file doesn’t exist, it will show the message: File missing!!!
.
Example code:
IF EXIST simple.txt (
echo File exists!!!
) ELSE (
echo File missing!!!
)
Output:
File exists!!!
Remember, the methods discussed here are written using Batch Script and only work in the Windows CMD.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn