How to Get the Current Batch File Directory
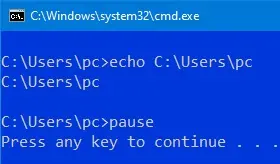
This article demonstrates how you can determine the location of a Batch file. Batch scripts are awesome for automation.
Sometimes you may need to get the location of the Batch file. This article will help you determine your working directory and the Batch file location.
Batch File Location
We can use various statics and variables within a Batch file to determine different locations. Let’s look at some examples.
The %cd%
variable will point to the current working directory. For example, we included it in our Batch file, shown below.
echo %cd%
pause
As shown below, we will get the working directory for running the script.
We will include the static illustrated below to get the name and full path of our Batch file.
%0
This should return the full path and name of our Batch file, as shown below.
To get only the full path without the name, use the parameter extension below.
%~dp0
In a nutshell, there are different ways to determine the working directory and the Batch file location.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn