How to System-Generate Filename With Timestamp in Batch Script
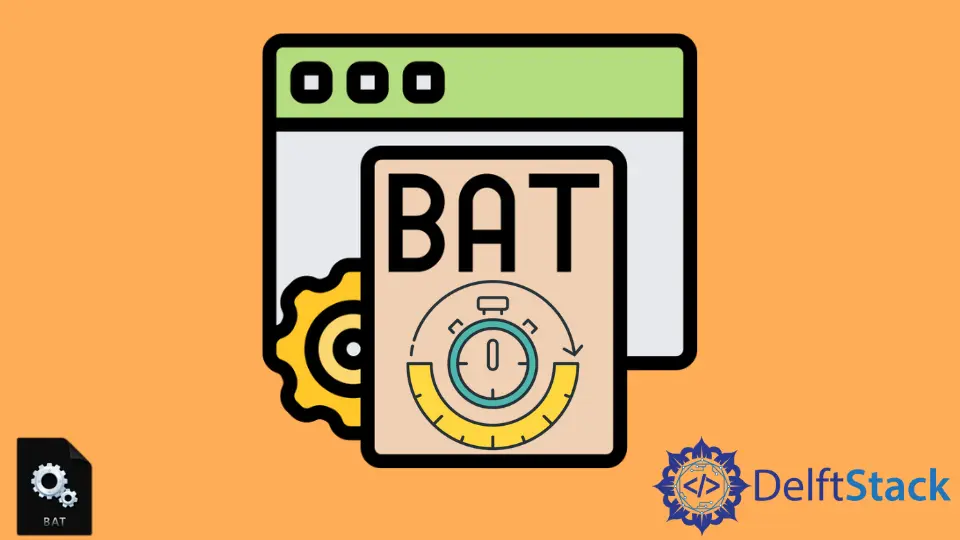
Sometimes, we generate system logs from a Batch Script. But, the problem here is the log file’s name; if the file name is not unique, it may replace the file that is named the same.
In this article, we will see how we can generate a unique file name from the system using the current time and date. Also, we will discuss a code and its explanation to make it easier to understand.
System-Generated Filename With Timestamp in Batch Script
We are using time and date here because the time and date are not always the same and provide information on the file name. In the below example, we will generate a log file with the name that is the combination of time and date.
The code for our example will look like the below:
@ECHO off
SET YY=%date:~10,4%
SET MM=%date:~4,2%
SET DD=%date:~7,2%
SET HH=%time:~0,2%
IF %HH% lss 10 (SET HH=0%time:~1,1%)
SET NN=%time:~3,2%
SET SS=%time:~6,2%
SET MS=%time:~9,2%
SET NEWFILENAME=%YY%%MM%%DD%-%HH%%NN%%SS%
ECHO "This is a data" > "G:\Directory\access_%NEWFILENAME%.txt"
Remember, Batch contains built-in variables for time and date; these are %time%
and %date%
. These variables produce time and date in a string format.
In our code, we separated the parts of the date from the string using these lines:
SET YY=%date:~10,4%
SET MM=%date:~4,2%
SET DD=%date:~7,2%
Similarly, we separated the parts of the time from the string using these lines:
SET HH=%time:~0,2%
IF %HH% lss 10 (SET HH=0%time:~1,1%)
SET NN=%time:~3,2%
SET SS=%time:~6,2%
SET MS=%time:~9,2%
Then, we created the filename with the combination of time and date using the line:
SET NEWFILENAME=%YY%%MM%%DD%-%HH%%NN%%SS%
And lastly, we created a file with the system-generated name in a specific directory using the line:
ECHO "This is a data" > "G:\Directory\access_%NEWFILENAME%.txt"
When you run this script on your command prompt, you will see a file whose name is the combination of date and time. And the file contains the text This is a data
.
Please note that the code we shared in this article is written in Batch script and only runs on the Windows Command Prompt environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn